How to Use the System.exit() Method in Java
- What is the System.exit() Method?
- Basic Usage of System.exit()
- Handling Exceptions with System.exit()
- Exiting from Multiple Threads
- Conclusion
- FAQ
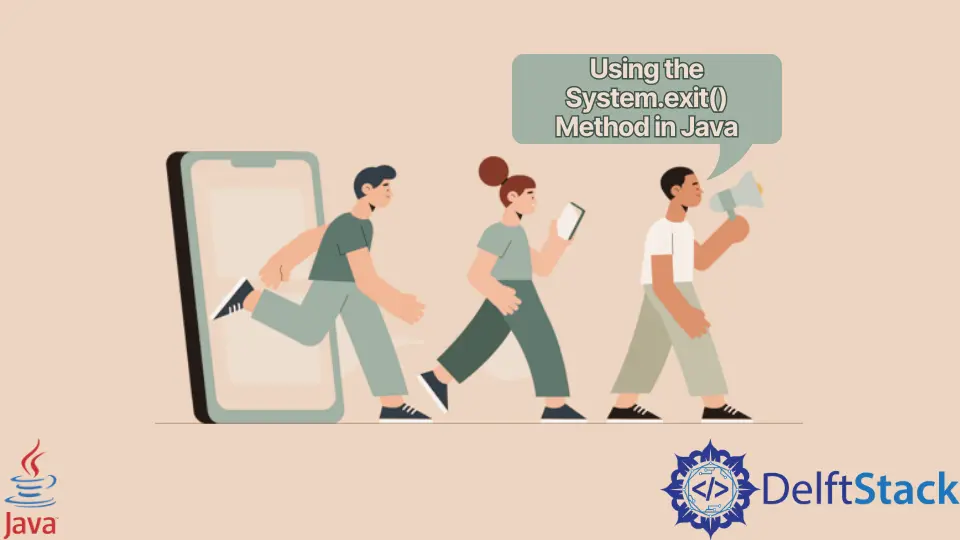
Java is a powerful programming language that offers a variety of methods to manage application behavior. One such method is System.exit()
, which plays a crucial role in terminating a Java program. Understanding how to use this method effectively can enhance your control over application flow, especially in scenarios where you need to exit gracefully or handle errors.
This article will dive into what the System.exit()
method is, how it works, and provide clear examples to help you grasp its functionality. Whether you’re a novice or an experienced Java developer, this guide will equip you with the knowledge to implement this method effectively in your projects.
What is the System.exit() Method?
The System.exit()
method is part of the Java System class and is used to terminate a Java program. This method takes a single integer argument, known as the status code. A status code of zero typically indicates a normal termination, while any non-zero value indicates an abnormal termination or an error. When invoked, System.exit()
initiates the shutdown process of the Java Virtual Machine (JVM), executing any shutdown hooks and finally exiting the program.
Using System.exit()
can be particularly useful in scenarios where you want to terminate the application due to certain conditions, such as encountering an unrecoverable error or when the user chooses to exit the program. However, it’s essential to use this method judiciously, as it will stop all threads and may not allow for proper resource cleanup unless explicitly handled.
Basic Usage of System.exit()
To use the System.exit()
method, you simply call it from your Java code, passing in the appropriate exit status code. Here’s a straightforward example:
public class ExitExample {
public static void main(String[] args) {
System.out.println("Program is about to exit.");
System.exit(0);
}
}
Output:
Program is about to exit.
In this example, the program prints a message indicating that it is about to exit and then calls System.exit(0)
. The zero status code signifies a normal termination. When this program runs, you’ll see the message, and then the JVM will terminate immediately.
The System.exit()
method is often used in applications that need to terminate based on specific conditions. For instance, if a user inputs invalid data, you might want to exit the program with an error status code to indicate that something went wrong. This is where the flexibility of System.exit()
shines, allowing developers to manage their application’s lifecycle effectively.
Handling Exceptions with System.exit()
One of the most practical uses of System.exit()
is in exception handling. When your program encounters an exception that it cannot handle, you can use this method to exit gracefully. Here’s how you can implement it:
public class ExceptionExitExample {
public static void main(String[] args) {
try {
int result = 10 / 0; // This will cause an ArithmeticException
} catch (ArithmeticException e) {
System.out.println("An error occurred: " + e.getMessage());
System.exit(1);
}
System.out.println("This line will not be executed.");
}
}
Output:
An error occurred: / by zero
In this example, we intentionally cause an ArithmeticException
by dividing by zero. The catch block captures the exception and prints an error message. Then, System.exit(1)
is called to terminate the program with a non-zero status code, indicating an error occurred. Notably, the last print statement will not execute because the program has already exited.
Using System.exit()
in this manner ensures that you can handle critical errors appropriately and provide feedback to the user or calling processes about the nature of the failure. It’s a good practice to use meaningful status codes to convey different types of errors, which can aid in debugging and monitoring.
Exiting from Multiple Threads
In multi-threaded applications, you may need to exit the program from a specific thread based on certain conditions. The System.exit()
method can be particularly useful in these scenarios. Here’s an example illustrating how to exit from a thread:
public class MultiThreadExitExample {
public static void main(String[] args) {
Thread thread = new Thread(() -> {
System.out.println("Thread is running.");
if (someCondition()) {
System.out.println("Condition met, exiting.");
System.exit(0);
}
});
thread.start();
}
private static boolean someCondition() {
return true; // Simulating a condition that leads to exit
}
}
Output:
Thread is running.
Condition met, exiting.
In this example, we create a new thread that runs a simple task. If a certain condition is met (in this case, always true for demonstration), the thread prints a message and calls System.exit(0)
. This will terminate the entire Java application, including any other threads that might be running.
Exiting from a thread can be a powerful tool, especially when dealing with background tasks or long-running processes. However, it’s vital to ensure that you are terminating the application intentionally and that it does not lead to data loss or resource leaks. Always consider the implications of abruptly stopping your application and implement necessary cleanup procedures if needed.
Conclusion
The System.exit()
method in Java is a crucial tool for managing application termination. Whether you’re handling exceptions, exiting from multiple threads, or simply needing to stop your program under specific conditions, this method provides the flexibility you need. However, it’s essential to use it wisely, ensuring that your application can exit gracefully and that resources are properly managed. Remember, a well-structured exit strategy can significantly improve the reliability and user experience of your Java applications.
FAQ
-
What does the System.exit() method do?
The System.exit() method is used to terminate the currently running Java Virtual Machine (JVM) and can take an exit status code as an argument. -
What is the difference between exit status 0 and non-zero?
An exit status of 0 indicates a normal termination, while a non-zero status indicates an abnormal termination or an error. -
Can I use System.exit() in a multi-threaded application?
Yes, System.exit() can be called from any thread to terminate the entire application, but it should be used with caution to avoid data loss. -
Is there a way to clean up resources before exiting?
Yes, you can implement shutdown hooks using Runtime.getRuntime().addShutdownHook(Thread) to perform cleanup tasks before the JVM exits. -
How can I handle exceptions with System.exit()?
You can catch exceptions in your code and call System.exit() with a non-zero status code to indicate an error occurred.