What Is a Driver Class in Java
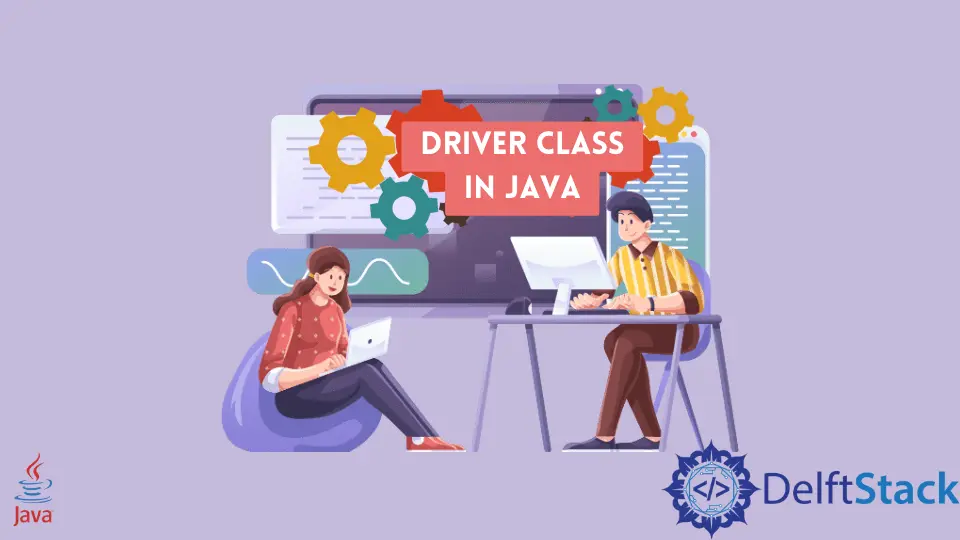
Understanding the concept of a driver class in Java is essential for anyone looking to deepen their knowledge of Java programming, especially when it comes to database connectivity. A driver class acts as a bridge between your Java application and the database, facilitating communication and data exchange.
This article will guide you through the intricacies of driver classes in Java, explain their importance, and provide insight into how to identify and implement them effectively. Whether you’re a beginner or an experienced developer, this comprehensive guide will enhance your understanding of driver classes and their role in Java applications.
What Is a Driver Class?
In Java, a driver class is a specific type of class that implements the JDBC (Java Database Connectivity) API to enable communication between a Java application and a database. Essentially, it acts as a translator, converting Java code into a format that the database can understand. The driver class is crucial for executing SQL queries and retrieving results from the database.
There are several types of JDBC drivers, including:
-
Type 1 Driver: Also known as the JDBC-ODBC bridge driver, this type translates JDBC method calls into ODBC calls. While it is simple to use, it is not recommended for production environments due to performance issues.
-
Type 2 Driver: This driver converts JDBC calls into database-specific calls using native libraries. It offers better performance than Type 1 but requires native database libraries on the client machine.
-
Type 3 Driver: This driver uses a middleware server to convert JDBC calls to database-specific calls. It is platform-independent and does not require native libraries.
-
Type 4 Driver: Also known as the thin driver, this type directly converts JDBC calls into the database-specific protocol. It is the most commonly used driver due to its performance and ease of use.
How to Identify a Driver Class in Java
Identifying the driver class in Java can be done through various methods. Here, we will explore two primary ways: checking the JDBC documentation and using Java code to list available drivers.
Method 1: Checking the JDBC Documentation
One of the simplest ways to identify a driver class is by consulting the JDBC documentation. Most database vendors provide detailed information about their JDBC drivers, including the class names and connection URLs. Here’s how you can do it:
-
Visit the Database Vendor’s Website: Go to the official website of the database you are using (e.g., MySQL, PostgreSQL, Oracle).
-
Locate the JDBC Driver Section: Look for the JDBC driver documentation, which typically includes information about the driver class name and how to set up the connection.
-
Take Note of the Class Name: The documentation will usually specify the fully qualified name of the driver class, which you will use in your Java application.
This method is straightforward and ensures you are using the correct driver class for your specific database version.
Method 2: Using Java Code to List Available Drivers
Another effective way to identify driver classes is by using Java code to list all available JDBC drivers. This method can be particularly useful if you are unsure which drivers are installed in your environment. Here’s how you can do it:
import java.sql.Driver;
import java.sql.DriverManager;
import java.util.Enumeration;
public class ListDrivers {
public static void main(String[] args) {
Enumeration<Driver> drivers = DriverManager.getDrivers();
while (drivers.hasMoreElements()) {
Driver driver = drivers.nextElement();
System.out.println("Driver: " + driver.getClass().getName());
}
}
}
When you run this code, it will print out the names of all the JDBC drivers that are currently registered with the DriverManager.
Output:
Driver: com.mysql.cj.jdbc.Driver
Driver: org.postgresql.Driver
This code snippet imports the necessary JDBC classes and uses the DriverManager
to retrieve an enumeration of the available drivers. By iterating through this enumeration, you can display the class names of each driver, giving you a clear understanding of what is available in your environment.
Conclusion
In summary, understanding the driver class in Java is vital for effective database interaction. By knowing how to identify and implement the appropriate driver class, you can ensure smooth communication between your Java applications and databases. Whether you check the JDBC documentation or use Java code to list available drivers, having this knowledge will enhance your programming skills and improve your application’s performance.
FAQ
-
What is the purpose of a driver class in Java?
A driver class in Java facilitates communication between a Java application and a database, allowing for the execution of SQL queries. -
How many types of JDBC drivers are there?
There are four types of JDBC drivers: Type 1 (JDBC-ODBC Bridge), Type 2 (Native-API Driver), Type 3 (Network Protocol Driver), and Type 4 (Thin Driver).
-
Can I use multiple driver classes in a single Java application?
Yes, you can use multiple driver classes in a single Java application, especially if your application interacts with different types of databases. -
How do I know which driver class to use for my database?
You can check the official documentation of your database vendor to find the recommended JDBC driver class for your specific database version. -
What happens if I use the wrong driver class?
Using the wrong driver class can lead to runtime exceptions, such as ClassNotFoundException or SQLException, preventing your application from connecting to the database.