How to Sort Manual Linked List With Bubble Sort Algorithm in Java
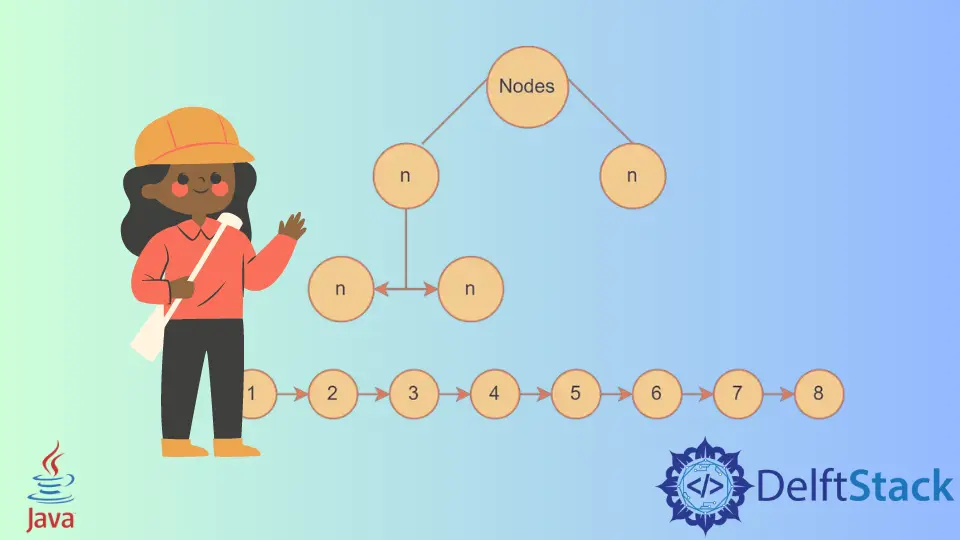
Bubble sort is the most common data structure algorithm used to sort data collection. It functions by iterating and swapping the adjacent elements in the wrong order until they are correct.
We will first show you the basic sorting demo. Then we will implement two bubble sort algorithms to sort linked lists in Java.
Bubble Sort in Java
Let’s keep things essential without getting into the arithmetic aspect of sorting. We will traverse an array from the start to the final index in the bubble sort.
Then only it can compare the current index to the following index. The core concept of this formula is when the present elements get greater by size than the next element.
Syntax:
for (int A = 0; A < sort - 1; A++)
for (int B = 0; B < sort - A - 1; B++)
if (DEMO[B] > DEMO[B + 1]) {
// Swapping of array
int temp = DEMO[B];
DEMO[B] = DEMO[B + 1];
DEMO[B + 1] = temp;
}
Now let’s execute a simple bubble sort algorithm in Java. But first, have a look at the initial state of the array’s unsorted index(s).
Array: {43, 65, 21, 64, 12, 6, 1}
The following code block will perform bubble sort on this array index by applying this basic sorting algorithm.
It is worth mentioning that we can always modify this formula depending on our requirements. However, the core should remain basic to form clarity at this moment.
Code:
// In this program, we will sort an array DEMO using the bubble sort algorithm
// Main class
public class BubbleSortLinkListExample1 {
// Main function
private void bubbleSort(int DEMO[]) {
// Using .length to determine entire length of array's index
int sort = DEMO.length;
// If array's length is less than int sort, increase it by 1
for (int A = 0; A < sort - 1; A++)
// Formula 1
for (int B = 0; B < sort - A - 1; B++)
if (DEMO[B] > DEMO[B + 1]) {
// Swapping of array
int temp = DEMO[B];
DEMO[B] = DEMO[B + 1];
DEMO[B + 1] = temp;
}
}
/* Now we are going to print DEMO array*/
void printArray(int DEMO[]) {
int sort = DEMO.length;
for (int A = 0; A < sort; ++A) System.out.print(DEMO[A] + " ");
System.out.println();
}
// We are going to implement a driver algorithm for sorting our DEMO array
public static void main(String args[]) {
BubbleSortLinkListExample1 ob = new BubbleSortLinkListExample1();
int DEMO[] = {43, 65, 21, 64, 12, 6, 1};
ob.bubbleSort(DEMO);
System.out.println("After the array has been sorted!");
ob.printArray(DEMO);
}
}
After sorting this array in ascending order, we get Java’s output.
Output:
After the array has been sorted!
1 6 12 21 43 64 65
Bubble Sort Manual Linked List in Java
The linked list manual sorting is also a straightforward method in bubble sort.
Have we discussed traversing data previously? Now, we will practice it.
Nodes allow us to traverse data from one node to the next.
Look at our demo model below. Since we did perform sorting in the previous example, it is significant to emphasize nodes here.
Class Sort Linked List in Java
As we understood, we apply this class to form nodes in Java.
Code:
class SortLL {
public static class Mynode {
int indx;
Mynode fwdMynode;
public Mynode(int indx) {
this.indx = indx;
this.fwdMynode = null;
}
public int getindx() {
return this.indx;
}
}
.setNextNode()
function that accepts a node and sets the next instance variable accordingly.You can use these classes separately and call their objects, but that is one lengthy and complicated way. Hence, we have kept all the classes in one file.
Likewise, we will use the .getNextNode()
function, it retrieves the next class element with no arguments. You must be familiar with the concept, so let’s execute the manual bubble sort algorithm without further ado.
-
Linked list before sorting:
Code:
class SortLL { public static class Mynode { int indx; Mynode fwdMynode; public Mynode(int indx) { this.indx = indx; this.fwdMynode = null; } public int getindx() { return this.indx; } } // My node class private Mynode head; private int size; public SortLL() { this.head = null; this.size = 0; } public void add(int indx) { Mynode Mynode = new Mynode(indx); if (head == null) { head = Mynode; } else { Mynode CN = head; while (CN.fwdMynode != null) { CN = CN.fwdMynode; } CN.fwdMynode = Mynode; } size++; } public void sort() { if (size > 1) { boolean dtr; do { Mynode thisMynode = head; Mynode ladtMynode = null; Mynode fwd = head.fwdMynode; dtr = false; while (fwd != null) { if (thisMynode.indx > fwd.indx) { dtr = true; if (ladtMynode != null) { Mynode sig = fwd.fwdMynode; ladtMynode.fwdMynode = fwd; fwd.fwdMynode = thisMynode; thisMynode.fwdMynode = sig; } else { Mynode sig = fwd.fwdMynode; head = fwd; fwd.fwdMynode = thisMynode; thisMynode.fwdMynode = sig; } ladtMynode = fwd; fwd = thisMynode.fwdMynode; } else { ladtMynode = thisMynode; thisMynode = fwd; fwd = fwd.fwdMynode; } } } while (dtr); } } public int listSize() { return size; } public void printindx() { Mynode CN = head; while (CN != null) { int indx = CN.getindx(); System.out.print(indx + " "); CN = CN.fwdMynode; } System.out.println(); } public boolean isEmpty() { return size == 0; } } // indxInterface class class SrtBubb { public static void main(String[] args) { SortLL s = new SortLL(); s.add(12); s.add(2); s.add(7); s.add(19); s.add(23); s.add(9); System.out.println("Before Performing Bubble Sort"); s.printindx(); s.sort(); System.out.println("After Performing Bubble Sort"); s.printindx(); System.out.println("Size of the linked list is: " + s.listSize()); } }
-
After performing the manual bubble sort:
Output:
Before Performing Bubble Sort 12 2 7 19 23 9 After Performing Bubble Sort 2 7 9 12 19 23 Size of the linked list is: 6
You can modify this program based on your requirements. But this is the most practical method for beginners to understand the manual sorting of linked lists using bubble sort.
Suppose you are still confused about this topic. We have also provided the code in the file directory for you.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn