How to Fix the Bad Operand Types Error in Java
-
Bad Operand Types for the
&
Operator in Java -
Bad Operand Types for the
&&
Operator in Java -
Bad Operand Types for the
==
Operator in Java -
Bad Operand Types for the
<=
Operator in Java - Conclusion
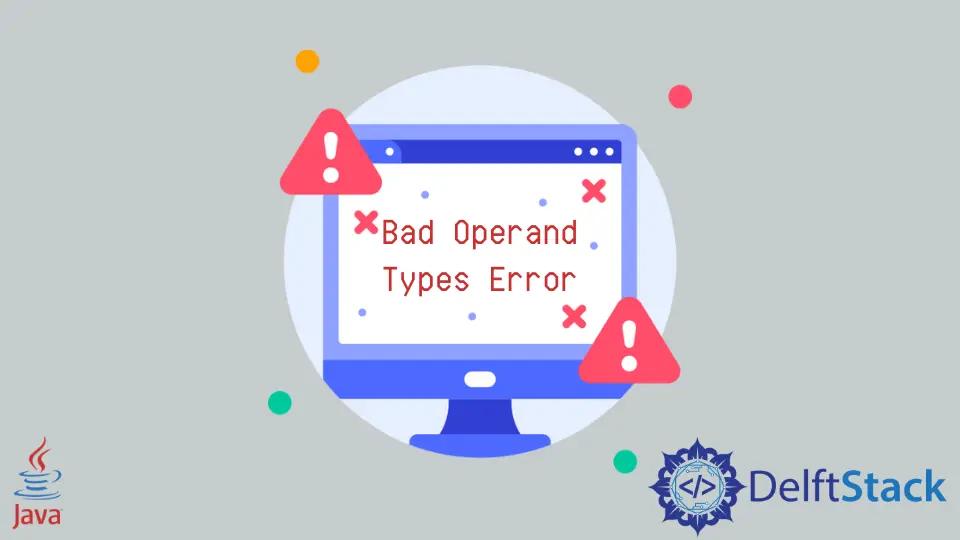
This tutorial introduces the bad operand types
error for binary operators in Java. Operators such as arithmetic operators and relational operators are called binary operators.
In Java, the bad operand types
error typically occurs when there is a mismatch between the types of operands used with a binary operator. The specific methods that may be associated with this error can vary depending on the context in which it occurs.
The bad operand types
error is a compile-time error when both types of operands are incompatible. Sometimes, the order of precedence of operators can also lead to incompatible operator types and result in an error to the console.
In this article, we will look at different examples of how this error occurs and how to resolve it.
Bad Operand Types for the &
Operator in Java
In Java, the message bad operand types for the '&' operator
typically indicates a compilation error. This error can occur when the &
bitwise AND
operator is applied to incompatible operand types.
The &
operator is designed for integral types like int
, long
, etc. If used with non-integral types or incompatible data types, such as strings or objects, it results in a compilation error.
Case 1: Incompatible Types
The &
operator in Java is a bitwise AND
operator, and it can be used with integer types. Here’s an example of using the &
operator with incompatible types:
public class BadOperandExample {
public static void main(String[] args) {
int number = 5;
boolean flag = true;
int result = number & flag;
System.out.println(result);
}
}
In this example, you’re attempting to perform a bitwise AND
operation (&
) between an int
(number
) and a boolean
(flag
). This will lead to a compilation error similar to:
Output:
error: bad operand types for binary operator '&'
if (x & 21 == 1) {
^
first type: int
second type: boolean
1 error
The error message indicates that there are bad operand types for the binary operator '&'
and specifies that the first type is int
and the second type is boolean
.
To solve this issue, you need to make sure that the operands used with the &
operator are of compatible types. In this case, you might want to convert the boolean
to an integer or use a logical AND
(&&
) if that fits the intended logic.
Here’s an example using a logical AND
:
public class FixedOperandExample {
public static void main(String[] args) {
int number = 5;
boolean flag = true;
// Using logical AND (&&) instead of bitwise AND (&)
boolean result = (number > 0) && flag;
System.out.println(result);
}
}
In this corrected example, we use the logical AND
(&&
) operator, which is suitable for boolean operands. The expression (number > 0) && flag
will be evaluated as true
if both conditions are true.
Output:
true
Case 2: Order of Precedence
There’s an issue with the usage of the bitwise AND
(&
) operator in the condition of the if
statement. The problem is that the equality
operator (==
) has higher precedence than the bitwise AND
operator, so the expression is evaluated as (x & 21) == 1
.
This may result in a bad operand types
error since the types are not compatible for the equality check.
public class BadOperandExample2 {
public static void main(String args[]) {
int x = 43;
if (x & 21 == 1) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
This will lead to a compilation error similar to:
error: bad operand types for binary operator '&'
if (x & 21 == 1) {
^
first type: int
second type: boolean
1 error
To solve this issue, add parentheses around the bitwise AND
operation (x & 13)
to ensure it is evaluated first before the equality check. This resolves the bad operand types
issue and ensures that the types are compatible for the bitwise AND
operation and the subsequent equality check.
Here’s the corrected version of the code:
public class FixedOperandExample2 {
public static void main(String args[]) {
int x = 43;
// Corrected: Use parentheses to ensure the bitwise AND operation is performed first.
if ((x & 21) == 1) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
Output:
if block executing
Bad Operand Types for the &&
Operator in Java
The bad operand types for the '&&' operator
error occurs when the logical AND
operator (&&
) is applied to incompatible operand types during compilation. The &&
operator is meant for boolean values, so if it is used with non-boolean types or expressions that do not result in boolean values, a compilation error is triggered.
Case 1: Incompatible Types
Let’s consider an example where you use the &&
operator with incompatible types:
public class BadOperandExample {
public static void main(String[] args) {
boolean flag1 = true;
int number = 5;
boolean result = flag1 && number;
System.out.println(result);
}
}
In this example, you’re attempting to use the logical AND
operator (&&
) between a boolean
(flag1
) and an int
(number
). This will lead to a compilation error similar to:
error: bad operand types for binary operator '&&'
boolean result = flag1 && number;
^
first type: boolean
second type: int
1 error
The error message indicates that there are bad operand types for the binary operator '&&'
and specifies that the first type is boolean
and the second type is int
.
To solve this issue, you need to make sure that both operands used with the &&
operator are of type boolean
. If you want to perform a logical AND
operation involving the int
value, you can use a relational operator to compare the int
value and then use &&
.
Here’s an example:
public class FixedOperandExample {
public static void main(String[] args) {
boolean flag1 = true;
int number = 5;
// Using a relational operator to compare the int value
boolean result = (number > 0) && flag1;
System.out.println(result);
}
}
In this corrected example, we use the greater than (>
) relational operator to compare the int
value (number > 0
). The result of this comparison is a boolean
value, and then we use the logical AND
(&&
) operator with the flag1
boolean
.
The expression (number > 0) && flag1
will be evaluated as true
if both conditions are true.
Output:
true
Case 2: Using &&
with Different Operand Types
Here is an instance where we’re trying to use the logical AND
operator (&&
) with operands of different types
public class BadOperandExample2 {
public static void main(String args[]) {
int x = 23;
if ((x > 13) && (x * 3)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
The expression (x > 13) && (x * 3)
involves an integer multiplication, and you can’t directly use the result of the multiplication as a boolean
condition. This will lead to a compilation error similar to:
error: bad operand types for binary operator '&&'
if ((x > 13) && (x * 3)) {
^
first type: boolean
second type: int
1 error
Here’s the corrected code:
public class FixedOperandExample2 {
public static void main(String args[]) {
int x = 23;
// Corrected the condition by comparing the result of (x * 3) with a value
if ((x > 13) && (x * 3 > 0)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
In this corrected code, a modification of the condition (x > 13) && (x * 3)
to (x > 13) && (x * 3 > 0)
was made. This ensures that the result of the multiplication is compared with a value (in this case, 0
).
This way, the condition is evaluated to a boolean
value, and it can be used with the logical AND
operator. The expression (x > 13) && (x * 3 > 0)
will be true
if both conditions are true.
Output:
if block executing
Bad Operand Types for the ==
Operator in Java
The bad operand types for the '==' operator
error arises when the equality operator (==
) is used with incompatible operand types during compilation. This typically occurs when trying to compare objects of different classes or incompatible data types.
The ==
operator is designed for comparing primitive types or checking object references, and using it with inappropriate types leads to a compilation error.
Let’s consider an example where you use the ==
operator with incompatible types:
public class BadOperandExample2 {
public static void main(String args[]) {
int x = 23;
String y = "23";
if (x == y) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
In the provided code, it is trying to use the equality operator (==
) to compare an int
(x
) and a String
(y
). This will result in a compilation error because the ==
operator in Java cannot be directly used to compare values of different types, such as int
and String
.
This will lead to a compilation error similar to:
error: bad operand types for binary operator '=='
if (x == y) {
^
first type: int
second type: String
1 error
To correct this issue, you need to convert the String
to an int
before using the ==
operator. You can use Integer.parseInt()
for this purpose:
public class FixedOperandExample2 {
public static void main(String args[]) {
int x = 23;
String y = "23";
// Convert the String to an int and then compare
if (x == Integer.parseInt(y)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
In this corrected code, Integer.parseInt(y)
is used to convert the String
to an int
before performing the comparison. This ensures that both operands have compatible types for the ==
operator.
The expression x == Integer.parseInt(y)
will evaluate to true
if the values are equal.
Output:
if block executing
Bad Operand Types for the <=
Operator in Java
The bad operand types for the
<= operator
error occurs when the less than or equal to (<=
) operator is used with incompatible operand types during compilation. This error indicates that the operands involved in the comparison are not of types that support the less than or equal to operation.
Like the previous case example, the <=
(less than equals to) operator can also give a bad operator types
error when both the operands are of different types. Look at the example below:
public class BadOperandExample {
public static void main(String[] args) {
int number = 5;
String text = "10";
if (number <= text) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
In this example, you’re attempting to use the less than or equal to operator (<=
) between an int
(number
) and a String
(text
). This will lead to a compilation error similar to:
error: bad operand types for binary operator '<='
if (number <= text) {
^
first type: int
second type: String
1 error
The error message indicates that there are bad operand types for the binary operator '<='
and specifies that the first type is int
and the second type is String
.
To solve this issue, you need to make sure that both operands used with the <=
operator are of compatible numeric types. If you want to compare the numeric value of the String
to the int
, you can convert the String
to an int
using Integer.parseInt()
:
public class FixedOperandExample {
public static void main(String[] args) {
int number = 5;
String text = "10";
// Convert the String to an int and then compare
if (number <= Integer.parseInt(text)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
In this corrected example, Integer.parseInt(text)
is used to convert the String
to an int
, and then the less than or equal to operator (<=
) is used to compare the numeric values. The expression number <= Integer.parseInt(text)
will be evaluated as true
if the number
is less than or equal to the value obtained from converting the String
.
Output:
if block executing
Conclusion
bad operand types
errors occur when Java encounters incompatible data types in operations, violating type safety. It ensures that operations are meaningful and prevents unintended consequences that may arise from mixing incompatible types, promoting code reliability and reducing potential runtime errors.
When encountering bad operand types
errors, it’s crucial to ensure that the operands used with the operators are of compatible types based on the operator’s requirements. If necessary, use type conversion or adjust the logic to ensure consistent types for proper operations.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack