修復 Java 中的錯誤運算元型別錯誤
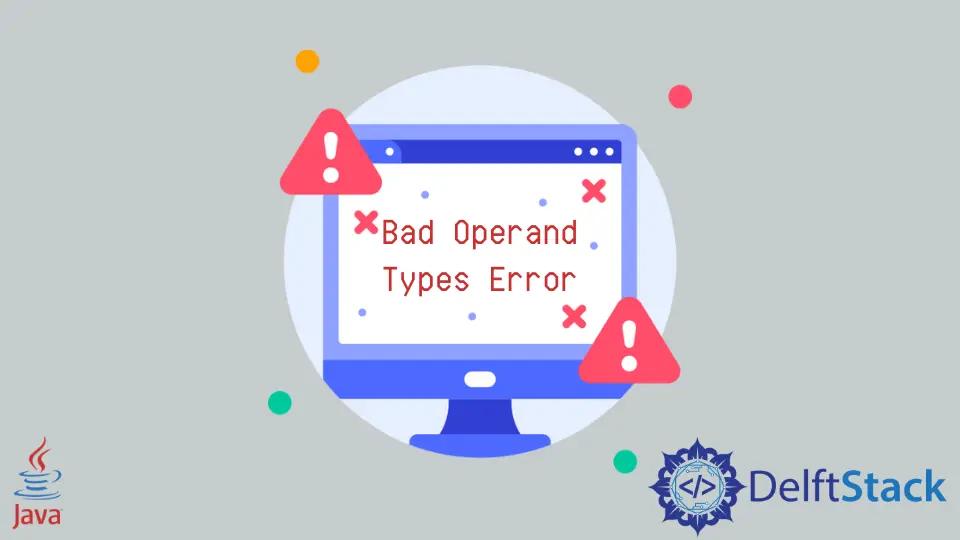
本教程介紹了 Java 中二元運算子的 bad operand types
錯誤。
二元運算子是需要兩個運算元的運算子。算術運算子和關係運算子等運算子稱為二元運算子。
運算子在程式設計中起著至關重要的作用,有時,由於使用不當,二元運算子會給出錯誤的運算元型別
錯誤。當兩種型別的運算元不相容時,bad operand types
錯誤是編譯時錯誤。
例如,我們將字串與整數進行比較時會出現此錯誤。本文將介紹此錯誤如何發生以及如何解決的不同示例。
有時,運算子的優先順序也可能導致運算子型別不相容並導致控制檯出錯。
Java 中&
運算子的錯誤運算元型別
讓我們首先了解 Java 程式碼中可能出現 bad operator error
的最常見情況。看下面的示例程式碼:
public class MyClass {
public static void main(String args[]) {
int x = 43;
if (x & 21 == 1) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
MyClass.java:4: error: bad operand types for binary operator '&'
if( x & 21 == 1){
^
first type: int
second type: boolean
1 error
發生此錯誤是因為 ==
(等於)運算子的優先順序高於&
運算子的優先順序。這導致了 21 == 1
評估,它給了我們一個布林值。
現在,請注意 &
有一個整數運算元和一個布林值。由於兩個運算子的型別不同,&
運算子不能工作,所以我們得到一個錯誤。
我們將使用括號表示需要首先評估 x & 21
以解決此錯誤。看下面修改後的程式碼:
public class MyClass {
public static void main(String args[]) {
int x = 43;
if ((x & 21) == 1) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
if block executing
Java 中&&
運算子的錯誤運算元型別
同樣,如果你正在使用邏輯&&
(和)運算子,那麼在某些情況下你可能會遇到 bad operand types
錯誤,例如以下示例程式碼:
public class MyClass {
public static void main(String args[]) {
int x = 43;
if ((x > 10) && (x * 5)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
MyClass.java:4: error: bad operand types for binary operator '&&'
if((x > 10) && (x*5)){
^
first type: boolean
second type: int
1 error
發生此錯誤是因為 &&
運算元需要兩個布林運算元。
這裡,表示式 x * 5
給出一個整數值。因此,這裡的&&
運算子有一個整數運算元,並給我們帶來 bad operand types
錯誤。
為了解決這個錯誤,我們將修改這個程式碼,使得 x * 5==21
返回一個布林值。看下面修改後的程式碼:
public class MyClass {
public static void main(String args[]) {
int x = 43;
if ((x > 10) && (x * 5 == 21)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
else block executing
Java 中 ==
運算子的錯誤運算元型別
使用 ==
等於運算子時,你可能會遇到相同的錯誤。如果傳遞的兩個運算元都是不同的型別,它會給出一個錯誤的操作符錯誤。
看下面的例子:
public class MyClass {
public static void main(String args[]) {
int x = 43;
String y = "43";
if (x == y) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
MyClass.java:5: error: bad operand types for binary operator '=='
if(x == y){
^
first type: int
second type: String
1 error
發生此錯誤是因為 ==
等於運算子的運算元屬於不同型別。一個是字串,另一個是整數。
要解決此錯誤,我們必須轉換其中一個以獲得相同的資料型別。如果我們將整數轉換為字串,則比較將按詞彙順序進行。
因此,我們將字串轉換為 int。看下面修改後的程式碼:
public class MyClass {
public static void main(String args[]) {
int x = 43;
String y = "43";
if (x == Integer.parseInt(y)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
if block executing
Java 中 <=
運算子的錯誤運算元型別
與前面的案例示例一樣,當兩個運算元的型別不同時,<=
(小於等於)運算子也可能給出 bad operator types
錯誤。看下面的例子:
public class MyClass {
public static void main(String args[]) {
int x = 43;
String y = "43";
if (x <= y) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
MyClass.java:5: error: bad operand types for binary operator '<='
if(x <= y){
^
first type: int
second type: String
1 error
要解決此錯誤,我們必須轉換其中一個以獲得相同的資料型別。看下面修改後的程式碼:
public class MyClass {
public static void main(String args[]) {
int x = 43;
String y = "43";
if (x <= Integer.parseInt(y)) {
System.out.println("if block executing");
} else {
System.out.println("else block executing");
}
}
}
輸出:
if block executing