The Difference Between ArrayList and LinkedList in Java
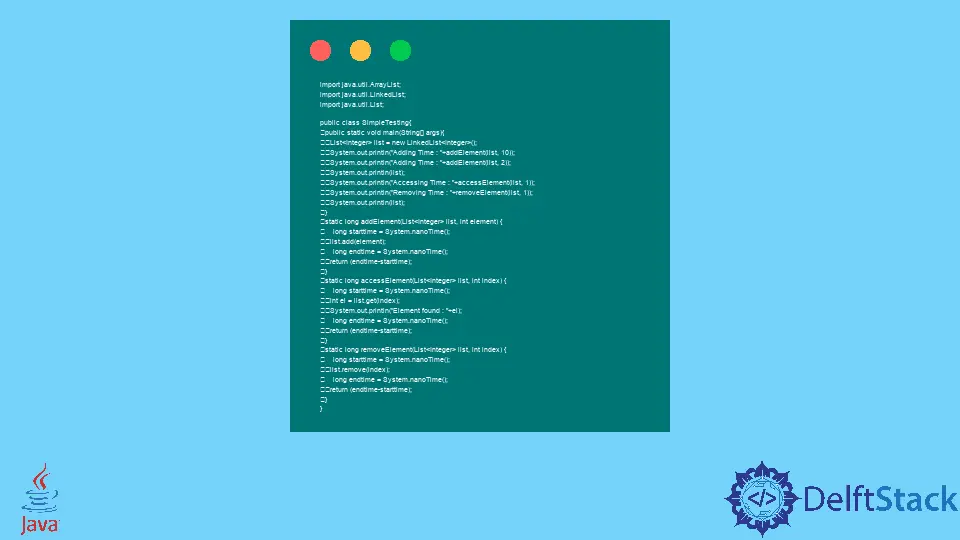
This tutorial introduces how to initialize char and the initial value of char type in Java.
In Java, ArrayList and LinkedList are both the classes that implement the List interface. The ArrayList is the resizable array implementation of the List interface, whereas LinkedList is the Doubly-linked list implementation of the List interface in Java.
ArrayList and LinkedList are both used to store data but have several differences due to implementation type. In this article, we will loop up some major differences between these with examples.
Operation | ArrayList | LinkedList |
---|---|---|
Adding Element | Take More Time | Take Less Time |
Accessing Element | Take Less Time | Take More Time |
Removing Element | Take More Time | Take Less Time |
Iterating Elements | Take Less Time | Take More Time |
Searching Element | Take Less Time | Take More Time |
- Performance Issues with LinkedList
It uses lots of small memory objects that are bad for cache-locality and create performance issues across the process.
Although LinkedList is fast for adding and deleting elements, it is very slow if we access a specific element. In contrast, ArrayList is fast for accessing a specific element but slow if we add and very slow if we delete elements from the middle.
ArrayList Example in Java
In this example, we created an ArrayList and applied several operations such as adding, removing and accessing elements. We measure the time while performing operations so that we can understand the performance difference between ArrayList and LinkedList. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
System.out.println("Adding Time : " + addElement(list, 10));
System.out.println("Adding Time : " + addElement(list, 2));
System.out.println(list);
System.out.println("Accessing Time : " + accessElement(list, 1));
System.out.println("Removing Time : " + removeElement(list, 1));
System.out.println(list);
}
static long addElement(List<Integer> list, int element) {
long starttime = System.nanoTime();
list.add(element);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long accessElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
int el = list.get(index);
System.out.println("Element found : " + el);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long removeElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
list.remove(index);
long endtime = System.nanoTime();
return (endtime - starttime);
}
}
Output:
Adding Time : 48556
Adding Time : 2090
[10, 2]
Element found : 2
Accessing Time : 42301
Removing Time : 6471
[10]
LinkedList Example in Java
In this example, we created a LinkedList and applied several operations such as adding, removing, and accessing elements. We measure the time as well while performing operations so that we can understand the performance difference between ArrayList and LinkedList. See the example below.
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = new LinkedList<Integer>();
System.out.println("Adding Time : " + addElement(list, 10));
System.out.println("Adding Time : " + addElement(list, 2));
System.out.println(list);
System.out.println("Accessing Time : " + accessElement(list, 1));
System.out.println("Removing Time : " + removeElement(list, 1));
System.out.println(list);
}
static long addElement(List<Integer> list, int element) {
long starttime = System.nanoTime();
list.add(element);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long accessElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
int el = list.get(index);
System.out.println("Element found : " + el);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long removeElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
list.remove(index);
long endtime = System.nanoTime();
return (endtime - starttime);
}
}
Output:
Adding Time : 82591
Adding Time : 4218
[10, 2]
Element found : 2
Accessing Time : 54516
Removing Time : 7572
[10]
Related Article - Java ArrayList
- How to Sort Objects in ArrayList by Date in Java
- How to Find Unique Values in Java ArrayList
- Vector and ArrayList in Java
- Differences Between List and Arraylist in Java
- How to Compare ArrayLists in Java
- Merge Sort Using ArrayList in Java