La diferencia entre ArrayList y LinkedList en Java
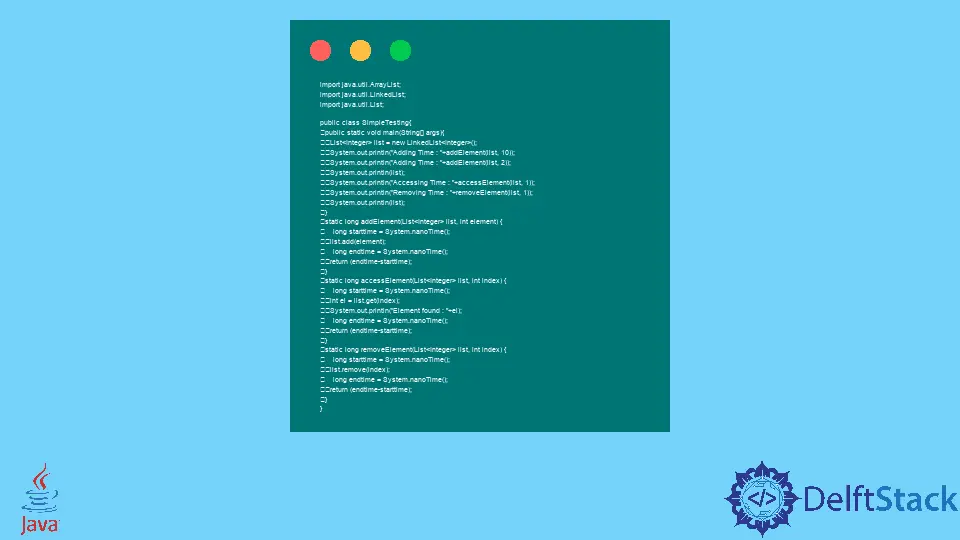
Este tutorial presenta cómo inicializar char y el valor inicial del tipo char en Java.
En Java, ArrayList y LinkedList son las dos clases que implementan la interfaz List. ArrayList es la implementación de matriz redimensionable de la interfaz List, mientras que LinkedList es la implementación de lista doblemente enlazada de la interfaz List en Java.
ArrayList y LinkedList se utilizan para almacenar datos, pero tienen varias diferencias debido al tipo de implementación. En este artículo, repasaremos algunas diferencias importantes entre estos con ejemplos.
Operación | ArrayList | LinkedList |
---|---|---|
Agregar elemento | Tómate más tiempo | Tómate menos tiempo |
Acceso a elemento | Tómate menos tiempo | Tómate más tiempo |
Eliminar elemento | Tómate más tiempo | Tómate menos tiempo |
Elementos iterativos | Tómate menos tiempo | Tómate más tiempo |
Elemento de búsqueda | Tómate menos tiempo | Tómate más tiempo |
- Problemas de rendimiento con LinkedList
Utiliza muchos objetos de memoria pequeños que son perjudiciales para la localidad de caché y crean problemas de rendimiento en todo el proceso.
Si bien LinkedList es rápido para agregar y eliminar elementos, es muy lento si accedemos a un elemento específico. En contraste, ArrayList es rápido para acceder a un elemento específico pero lento si agregamos y muy lento si eliminamos elementos del medio.
Ejemplo de ArrayList en Java
En este ejemplo, creamos una ArrayList y aplicamos varias operaciones como agregar, eliminar y acceder a elementos. Medimos el tiempo mientras realizamos operaciones para poder comprender la diferencia de rendimiento entre ArrayList y LinkedList. Vea el ejemplo a continuación.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
System.out.println("Adding Time : " + addElement(list, 10));
System.out.println("Adding Time : " + addElement(list, 2));
System.out.println(list);
System.out.println("Accessing Time : " + accessElement(list, 1));
System.out.println("Removing Time : " + removeElement(list, 1));
System.out.println(list);
}
static long addElement(List<Integer> list, int element) {
long starttime = System.nanoTime();
list.add(element);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long accessElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
int el = list.get(index);
System.out.println("Element found : " + el);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long removeElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
list.remove(index);
long endtime = System.nanoTime();
return (endtime - starttime);
}
}
Producción :
Adding Time : 48556
Adding Time : 2090
[10, 2]
Element found : 2
Accessing Time : 42301
Removing Time : 6471
[10]
Ejemplo de LinkedList en Java
En este ejemplo, creamos una LinkedList y aplicamos varias operaciones como agregar, eliminar y acceder a elementos. También medimos el tiempo mientras realizamos operaciones para que podamos comprender la diferencia de rendimiento entre ArrayList y LinkedList. Vea el ejemplo a continuación.
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = new LinkedList<Integer>();
System.out.println("Adding Time : " + addElement(list, 10));
System.out.println("Adding Time : " + addElement(list, 2));
System.out.println(list);
System.out.println("Accessing Time : " + accessElement(list, 1));
System.out.println("Removing Time : " + removeElement(list, 1));
System.out.println(list);
}
static long addElement(List<Integer> list, int element) {
long starttime = System.nanoTime();
list.add(element);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long accessElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
int el = list.get(index);
System.out.println("Element found : " + el);
long endtime = System.nanoTime();
return (endtime - starttime);
}
static long removeElement(List<Integer> list, int index) {
long starttime = System.nanoTime();
list.remove(index);
long endtime = System.nanoTime();
return (endtime - starttime);
}
}
Producción :
Adding Time : 82591
Adding Time : 4218
[10, 2]
Element found : 2
Accessing Time : 54516
Removing Time : 7572
[10]
Artículo relacionado - Java ArrayList
- Cómo ordenar objetos en ArrayList por fecha en Java
- Diferencias entre List y Arraylist en Java
- Encuentra valores únicos en Java ArrayList
- Vector y ArrayList en Java
- Comparar ArrayLists en Java
- Convertir ArrayList a Int Array en Java