How to Convert ArrayList to ObservableList in JavaFX
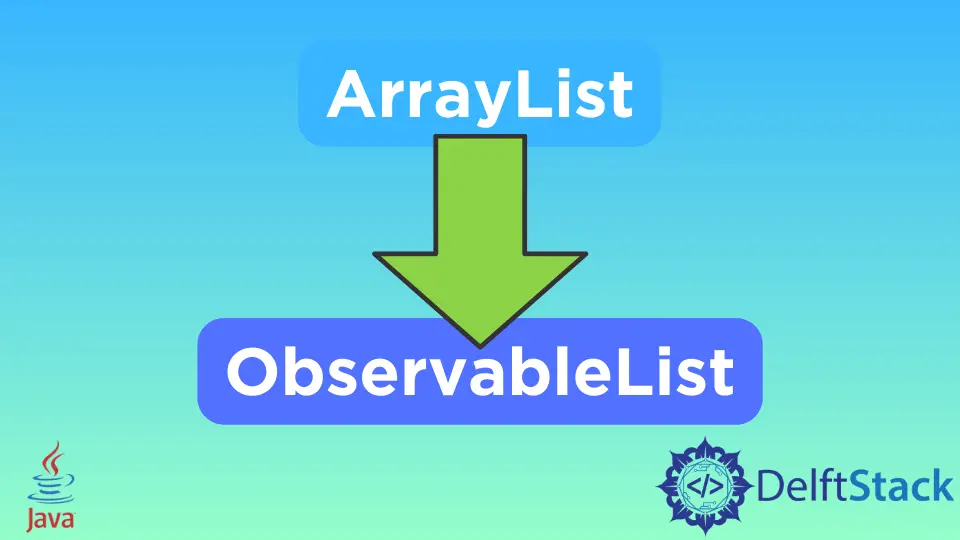
Sometimes we work with a list on our program. A list is a type of array.
There are two types of lists we can work with. The first one is the ArrayList, and the second one is the ObservableList.
The ArrayList is a class of resizable arrays where ObservableList allows a program to listen to and track occurring changes. Sometimes we need to convert an ArrayList to ObservableList for various purposes.
This article will explain how we can convert ArrayList to ObservableList. Also, we will see an example with output to make it easier to understand.
Convert ArrayList to ObservableList in JavaFX
So in our example, we will convert an ArrayList to an Observable list. We will start by including the necessary package files for the components we will use in our program.
Our example code will look like the following:
// Importing necessary packages
import java.util.ArrayList; // Package for the ArrayList
import java.util.List; // Package for the list
import javafx.collections.FXCollections; // Package for FxCollections
import javafx.collections.ListChangeListener; // Package for the listener for lists
import javafx.collections.ObservableList; // Package for the ObservableList
public class observableList {
// Our main method
public static void main(String[] args) {
List list = new ArrayList(); // create an array list of integer type
ObservableList ObList =
FXCollections.observableList(list); // create an observable list from array
ObList.addListener(new ListChangeListener() { // add an event listerer for the observable list
@Override
public void onChanged(
ListChangeListener.Change c) { // Method that will execute when any changes occured
System.out.println("Changes found ... "); // Show a message that a change occured
}
});
// add items to the observable List and check for size.
ObList.add(22);
System.out.println("The ObservableList size is: " + ObList.size());
list.add(44);
System.out.println("The ObservableList size is: " + ObList.size());
ObList.add(66);
System.out.println("The ObservableList size is: " + ObList.size());
}
}
The purpose of each line is commanded. Now, we will talk about the steps we follow to convert the ArrayList list
to the ObservableList ObList
.
The data type of both of these lists is an integer. First, we created an integer type ArrayList
named list
.
After that, we have created an ObservableList
name ObList
with the array list list
. We created the below event listener that detects changes made on the ObList
.
As an action, we showed a message Changes found...
on the console to inform the user that a change was found in ObList
.
public void onChanged(ListChangeListener.Change c) {
System.out.println("Changes found . . ");
}
This is a list change listener with ListChangeListener.Change
. You can add your preferred action to it based on your needs.
Lastly, we provided some data to the array to check whether our system works. After a successful compilation and run, you will get an output like the following.
Output:
Changes found...
The ObservableList size is: 1
The ObservableList size is: 2
Changes found...
The ObservableList size is: 3
Remember, if your IDE doesn’t support the automatic inclusion of libraries. Then you may need to include necessary library files manually before compiling; otherwise, it will show an error.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn