args Parameter in Java
- What is the Args Parameter?
- How to Use the Args Parameter in Java
- Practical Applications of the Args Parameter
- Error Handling with Command-Line Arguments
- Conclusion
- FAQ Section
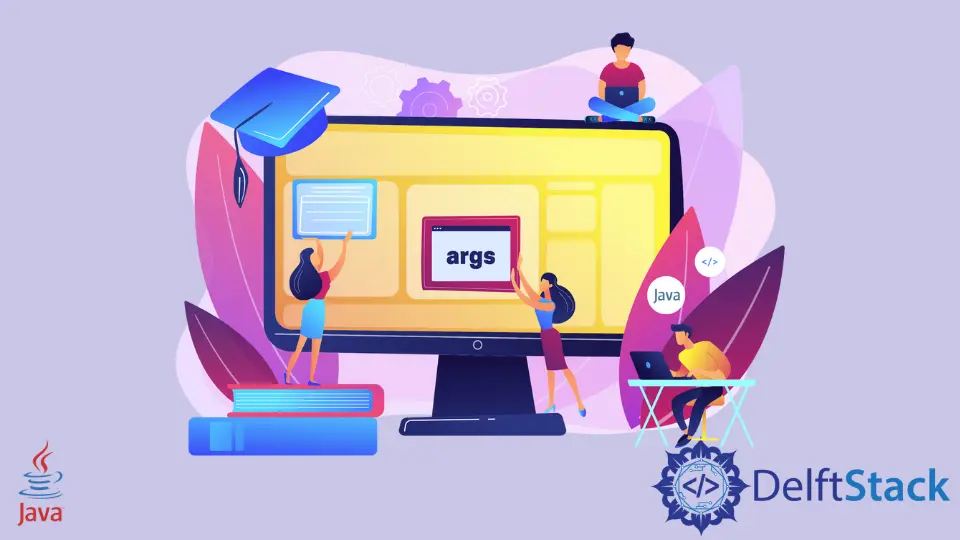
Java, a popular programming language, has a unique way of handling command-line arguments through the args
parameter in its main method.
This article will delve into the args
parameter, explaining its significance and how it can be utilized effectively in Java programs. Whether you are a beginner or an experienced developer, understanding how to work with command-line arguments can enhance your programming skills and improve the functionality of your applications. So, let’s explore the args
parameter, how to use it, and some practical examples to clarify its importance in Java.
What is the Args Parameter?
In Java, the args
parameter is an array of String
objects that stores the command-line arguments passed to the program when it is executed. The main method, which serves as the entry point for any Java application, is defined as follows:
public static void main(String[] args) {
// Your code here
}
When you run a Java program from the command line, you can provide additional information or parameters after the class name. These parameters are captured in the args
array. For instance, if you execute a program with the command java MyProgram arg1 arg2
, the args
array will contain two elements: args[0]
will be “arg1” and args[1]
will be “arg2”. This feature allows developers to create dynamic applications that can adapt based on user input.
How to Use the Args Parameter in Java
Let’s look at a practical example to understand how to utilize the args
parameter effectively. The following code demonstrates how to access and print command-line arguments in a Java program.
public class CommandLineArgs {
public static void main(String[] args) {
System.out.println("Number of arguments: " + args.length);
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
}
}
}
When you run this program with the command java CommandLineArgs Hello World
, the output will be as follows:
Output:
Number of arguments: 2
Argument 0: Hello
Argument 1: World
In this example, we first print the number of arguments received using args.length
. Then, we loop through the args
array and print each argument along with its index. This simple program illustrates how to read and utilize command-line arguments in Java, which can be useful for various applications, such as configuring settings or passing data to your program at runtime.
Practical Applications of the Args Parameter
The args
parameter can be employed in numerous practical scenarios. Here are a few examples:
-
Configuration Settings: You can pass configuration settings to your Java application via command-line arguments. This allows you to modify application behavior without changing the code.
-
File Handling: If your program processes files, you can pass file names as arguments, enabling users to specify which files to process.
-
User Input: Instead of prompting users for input during execution, you can accept command-line arguments to streamline the process.
Here’s an example demonstrating how to use command-line arguments for file processing:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileProcessor {
public static void main(String[] args) {
if (args.length < 1) {
System.out.println("Please provide a filename.");
return;
}
String filename = args[0];
try (BufferedReader br = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("An error occurred while reading the file: " + e.getMessage());
}
}
}
When executed with a filename, such as java FileProcessor myfile.txt
, the program will read and print the contents of myfile.txt
. The output will be the lines from the specified file.
Output:
Content of the file line 1
Content of the file line 2
...
This example shows how the args
parameter can be used to enhance user interaction and make your Java applications more flexible and powerful.
Error Handling with Command-Line Arguments
When working with command-line arguments, it’s essential to implement error handling to ensure your application runs smoothly. For instance, you should check if the required arguments are provided and handle potential exceptions gracefully.
Consider the following code snippet, which checks for the correct number of arguments before proceeding:
public class ArgumentChecker {
public static void main(String[] args) {
if (args.length != 2) {
System.out.println("Usage: java ArgumentChecker <arg1> <arg2>");
return;
}
String arg1 = args[0];
String arg2 = args[1];
System.out.println("Argument 1: " + arg1);
System.out.println("Argument 2: " + arg2);
}
}
If you run this program without the required two arguments, it will prompt the user with the correct usage.
Output:
Usage: java ArgumentChecker <arg1> <arg2>
This approach ensures that your program can handle unexpected input gracefully, improving the user experience and reducing potential errors.
Conclusion
Understanding the args
parameter in Java is crucial for creating dynamic and user-friendly applications. By effectively utilizing command-line arguments, developers can enhance the functionality of their programs, making them more versatile and adaptable to user needs. Whether you are passing configuration settings, processing files, or gathering user input, the args
parameter provides a powerful tool for Java developers. So, the next time you write a Java application, consider how the args
parameter can elevate your program’s capabilities.
FAQ Section
-
What is the purpose of the args parameter in Java?
Theargs
parameter allows you to pass command-line arguments to your Java program, enabling dynamic input at runtime. -
How do I access command-line arguments in Java?
Command-line arguments can be accessed using theargs
array in the main method, where each argument is stored as a string. -
Can I pass multiple arguments to a Java program?
Yes, you can pass multiple arguments separated by spaces when executing the program from the command line. -
What happens if I don’t provide any arguments?
If no arguments are provided,args.length
will be zero, and you can handle this scenario gracefully in your code. -
How can I validate command-line arguments in Java?
You can validate command-line arguments by checking the length of theargs
array and ensuring it meets your program’s requirements.