Anonymous Inner Class in Java
- Anonymous Inner Class in Java
- Variables and Members of the Anonymous Inner Class in Java
- Creation of Anonymous Inner Classes in Java
- Different Types of Anonymous Inner Class in Java
- Advantages of the Anonymous Inner Class in Java
- Conclusion
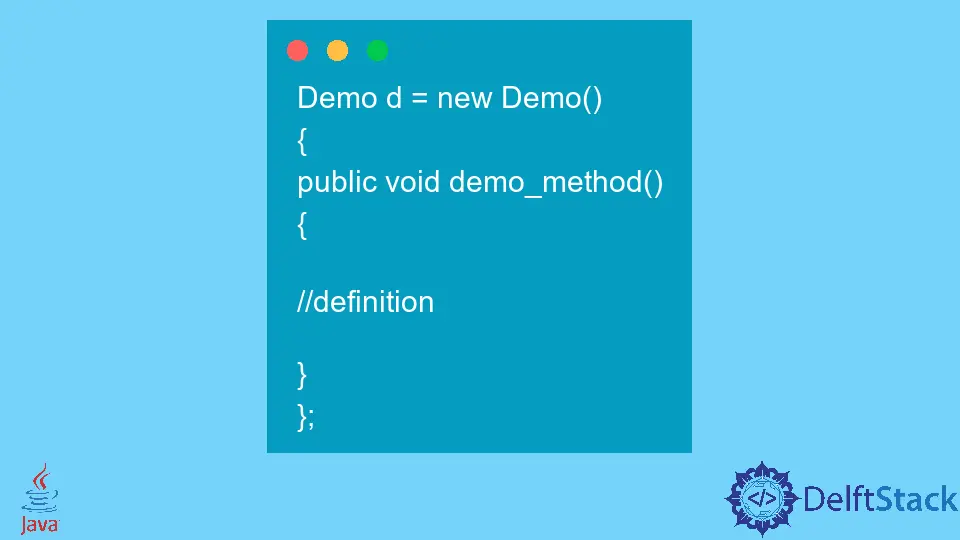
This article talks about the Anonymous Inner class in Java and its usefulness.
Anonymous Inner Class in Java
The word anonymous means - of unknown name. The same is true for the Java anonymous inner class.
An inner class without a name and for which we only need to create a single object is known as the Java anonymous inner class. It is useful when we have to make an instance of the object when we have to do some additional things like overloading methods from a class or an interface.
We can do this by using the Java anonymous Inner Class without making the subclass of the class.
In other words, a class without a name is called the Java anonymous inner class. Usually, whenever we create a new class, it has a name.
Therefore, the Java anonymous inner class is not used to create new classes. Rather, we use for overriding methods of a class or interface.
They are also useful in graphics programming, where we write implementation classes for listener interfaces.
The syntax of the Java anonymous inner class resembles the calling of a constructor except that the class definition is also present inside the block of code.
Syntax:
Demo d = new Demo() {
public void demo_method() {
// definition
}
};
Here, demo
refers to an abstract/concrete class or an interface. To better understand the concept of an anonymous inner class, let us see how it is different from a regular Java class.
- We can implement any number of interfaces using a normal class, but the anonymous inner class implements only one interface at a time.
- A regular class can extend another class and simultaneously implement several interfaces. While on the other hand, an anonymous inner class can do only one of these things at a time.
- The constructor’s name is the same as the name of a class. It implies that we can write any number of constructors in a regular class, but this is not true for an anonymous inner class.
To know more about inner classes in Java, refer to this documentation.
Variables and Members of the Anonymous Inner Class in Java
Anonymous inner classes also capture variables just like any local class in Java. The access to local variables is the same as an enclosing class.
The members of the enclosing class are accessible by the anonymous class. However, it cannot access those local variables (not even those present in its enclosing scope) that are not final.
Moreover, if we declare any type in an anonymous class, it shadows other declarations that have the same name in that scope. The anonymous inner class has some restrictions as well.
- The anonymous inner class does not allow the declaration of static initializers or member interfaces.
- Static members can be a part of the anonymous class if they are constant variables.
It concludes that we can declare a field, extra methods, instance initializers, and local classes inside an anonymous class.
Creation of Anonymous Inner Classes in Java
There are two main ways that we can use to create an anonymous inner class. These include the use of:
- A class (abstract or concrete)
- An interface
Let us look at them one by one.
Use a Class to Create an Anonymous Inner Class in Java
First, look at the example below.
abstract class Demo {
abstract void demo();
}
class AnonymousDemo {
public static void main(String args[]) {
Demo d = new Demo() {
void demo() {
System.out.println("This is a demo of an anonymous inner class using class.");
}
};
d.demo();
}
}
Output:
This is a demo of an anonymous inner class using class.
Here, a class is created whose name is decided by the compiler. This class extends the Demo
class and implements the demo()
method.
The object of an anonymous class is d
. It is the anonymous type Demo
class’ reference variable. Note that we save this as AnonymousDemo.java
for compilation.
The code below is how the compiler generates the internal class.
import java.io.PrintStream;
static class AnonymousDemo$1 extends Demo {
AnonymousDemo$1() {}
void demo() {
System.out.println("This is a demo of an anonymous inner class using class.");
}
}
Use an Interface to Create an Anonymous Inner Class in Java
In the above section, we use a class. But here, we will use an interface for the anonymous inner class. Let us understand this using an example.
interface Demonstration {
void demo();
}
class AnonymousDemo {
public static void main(String args[]) {
Demonstration d = new Demonstration() {
public void demo() {
System.out.println("This is an example of an anonymous inner class using an interface.");
}
};
d.demo();
}
}
Output:
This is an example of an Anonymous inner class using an interface.
Here, a class is created whose name is decided by the compiler. This class implements the interface Demonstration and gives the implementation of the demo()
method.
The object of an anonymous class is d
. It is the reference variable of the Demonstration type. Note that we save this as AnonymousDemo.java
for compilation.
The code below is how the compiler generates the internal class.
import java.io.PrintStream;
static class AnonymousDemo$1 implements Demonstration {
AnonymousDemo$1() {}
void demo() {
System.out.println("This is an example of an anonymous inner class using an interface.");
}
}
Different Types of Anonymous Inner Class in Java
The anonymous inner classes are classified into 3 types based on declaration and behavior.
- Anonymous Inner class that extends a class.
- Anonymous Inner class that implements an interface.
- Anonymous Inner class is defined inside the method/constructor argument.
Let us discuss all these three types one by one.
Anonymous Inner Class That Extends a Class in Java
We can create a thread by extending a Thread class, and we can also have an anonymous inner class that extends a class.
We use the new
keyword here to create an object that references the parent class type. Look at the example below.
class Demo {
public void example() {
System.out.println("Types of Anonymous classes");
}
}
public class AnonymousDemo {
public static void main(String args[]) {
Demo d1 = new Demo();
d1.example();
Demo d2 = new Demo() {
@Override
public void example() {
System.out.println("Type - 1");
}
};
d2.example();
}
}
Output:
Types of Anonymous classes
Type - 1
Anonymous Inner Class That Implements an Interface in Java
We can create a thread by implementing the Runnable interface, and the anonymous inner class can also implement an interface. We use the new
keyword to create an anonymous inner class object that references an interface type.
interface Demo {
public void demo();
}
public class AnonymousDemo {
public static void main(String args[]) {
Demo d = new Demo() {
@Override
public void demo() {
System.out.println("Type - 2");
}
};
d.demo();
System.out.println(d.getClass().getName());
}
}
Output:
Type - 2
AnonymousDemo$1
Anonymous Inner Class Inside a Method/Constructor Argument in Java
The anonymous inner class inside a method or constructor argument finds use in GUI
(Graphical user interface) applications. Here, we use the anonymous inner class as an argument and pass it to methods or constructors.
Here is an example.
abstract class Demo {
public abstract void demo();
}
class Example {
public void example(Demo d) {
d.demo();
}
}
public class AnonymousDemo {
public static void main(String args[]) {
Example e = new Example();
e.example(new Demo() {
@Override
public void demo() {
System.out.println("Type - 3");
}
});
}
}
Output:
Type - 3
Advantages of the Anonymous Inner Class in Java
The anonymous inner class closes over the method’s local variables, an added advantage over inner classes. Moreover, these classes reduce verbosity and provide reusability.
Also, these classes help developers as they don’t have to find a name for every class on their own. We can declare and instantiate a class using the anonymous inner classes simultaneously.
On the whole, the anonymous inner class is one of the advantages of Java.
To learn more about the anonymous inner class in Java, refer to this documentation.
Conclusion
In this article, we discussed the Java anonymous inner class. We saw the difference between regular classes and anonymous inner classes.
We also discussed the usage and advantages of the Java anonymous inner class. Moreover, we understood the implementation and syntax through codes and examples. We even learned about the various types of Java anonymous inner classes.
In conclusion, the anonymous inner class in Java adds to the functionality and versatility of the language by providing reusability and reducing wordiness.