String Interpolation in Go
- Using fmt.Sprintf for String Interpolation
- Using String Concatenation
- Using Go Templates for String Interpolation
- Conclusion
- FAQ
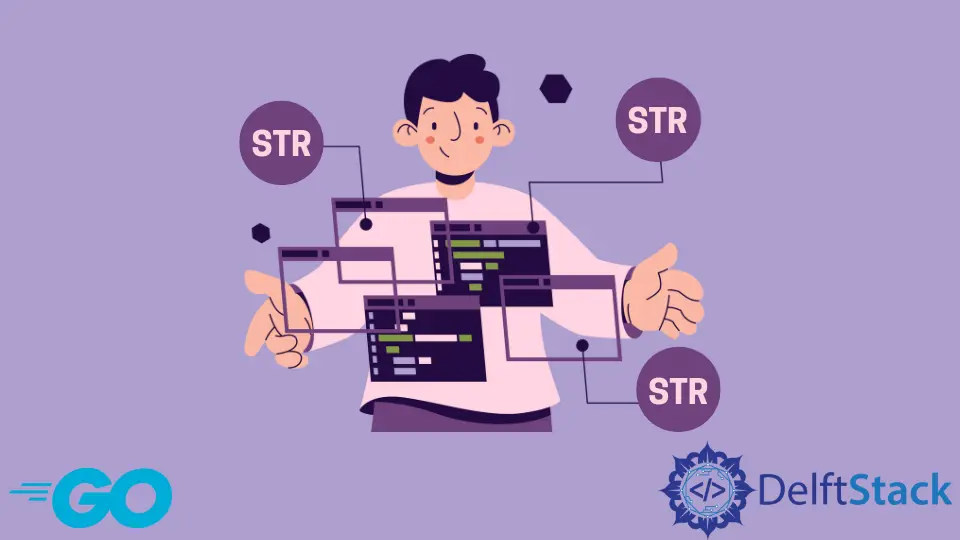
String interpolation is a powerful feature in programming that allows developers to insert variables into strings seamlessly. In Go, also known as GoLang, there are several methods to achieve this, each offering its own advantages in terms of readability and conciseness. Whether you’re working on a small project or a large application, understanding how to interpolate strings effectively can enhance your coding experience.
This article will explore the various ways you can perform string interpolation in Go, providing clear examples and explanations to help you grasp the concepts easily. By the end, you’ll be equipped with the knowledge to use these techniques in your own Go projects.
Using fmt.Sprintf for String Interpolation
One of the most common methods for string interpolation in Go is using the fmt.Sprintf
function from the fmt
package. This function allows you to format strings with placeholders, which can be replaced by the values of variables.
Here’s a simple example that demonstrates how to use fmt.Sprintf
:
package main
import (
"fmt"
)
func main() {
name := "Alice"
age := 30
greeting := fmt.Sprintf("Hello, my name is %s and I am %d years old.", name, age)
fmt.Println(greeting)
}
Output:
Hello, my name is Alice and I am 30 years old.
In this example, fmt.Sprintf
takes a format string and a list of variables. The %s
and %d
are placeholders for a string and an integer, respectively. When the function is called, it replaces these placeholders with the values of name
and age
. The result is a new string that is then printed to the console. This method is particularly useful when you need to construct complex strings dynamically, as it allows for clear and readable code.
Using String Concatenation
Another straightforward method for string interpolation in Go is string concatenation. This involves using the +
operator to combine strings and variables into a single string. While this method may not be as elegant as fmt.Sprintf
, it is simple and effective for basic use cases.
Here’s how you can concatenate strings in Go:
package main
import "fmt"
func main() {
name := "Bob"
age := 25
greeting := "Hello, my name is " + name + " and I am " + fmt.Sprint(age) + " years old."
fmt.Println(greeting)
}
Output:
Hello, my name is Bob and I am 25 years old.
In this example, we use the +
operator to concatenate the string literals with the variable name
and the string representation of age
. The fmt.Sprint
function is used to convert the integer age
into a string before concatenation. While this method is straightforward, it can become unwieldy with longer strings or multiple variables, making it less ideal for complex scenarios.
Using Go Templates for String Interpolation
Go also provides a robust templating system through the text/template
package, which can be extremely useful for string interpolation, especially in web applications. This method allows you to define a template with placeholders and then fill those placeholders with dynamic data.
Here’s a simple example using Go templates:
package main
import (
"os"
"text/template"
)
type Person struct {
Name string
Age int
}
func main() {
person := Person{Name: "Charlie", Age: 28}
tmpl, err := template.New("greeting").Parse("Hello, my name is {{.Name}} and I am {{.Age}} years old.")
if err != nil {
panic(err)
}
tmpl.Execute(os.Stdout, person)
}
Output:
Hello, my name is Charlie and I am 28 years old.
In this code, we define a Person
struct that holds the Name
and Age
fields. We then create a new template and parse a string containing placeholders in the format {{.FieldName}}
. The Execute
method is called to fill in the placeholders with the values from the person
struct. This method is particularly powerful because it separates the logic of your application from the presentation, making it easier to maintain and modify.
Conclusion
String interpolation in Go is essential for any developer looking to write clean and efficient code. Whether you choose to use fmt.Sprintf
, string concatenation, or Go templates, each method has its own strengths and can be applied depending on the complexity of your project. By mastering these techniques, you can significantly enhance your ability to generate dynamic strings in your Go applications. As you continue to explore Go, remember that clear and readable code is just as important as functionality.
FAQ
-
What is string interpolation in Go?
String interpolation in Go is the process of inserting variable values into string literals for easy and dynamic string generation. -
Is fmt.Sprintf the only way to interpolate strings in Go?
No, besides fmt.Sprintf, you can also use string concatenation and Go templates for string interpolation. -
When should I use Go templates for string interpolation?
Go templates are particularly useful when dealing with complex data structures or when you need to separate your application logic from presentation. -
Can I use string concatenation for complex strings?
While string concatenation is simple, it can become unwieldy with longer strings or multiple variables. In such cases, consider using fmt.Sprintf or Go templates. -
Are there performance differences between these methods?
Yes, performance can vary. Generally, fmt.Sprintf is slower than simple concatenation, but Go templates offer more flexibility and maintainability for complex scenarios.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn