Go での文字列補間
Musfirah Waseem
2023年6月20日
Go
Go String
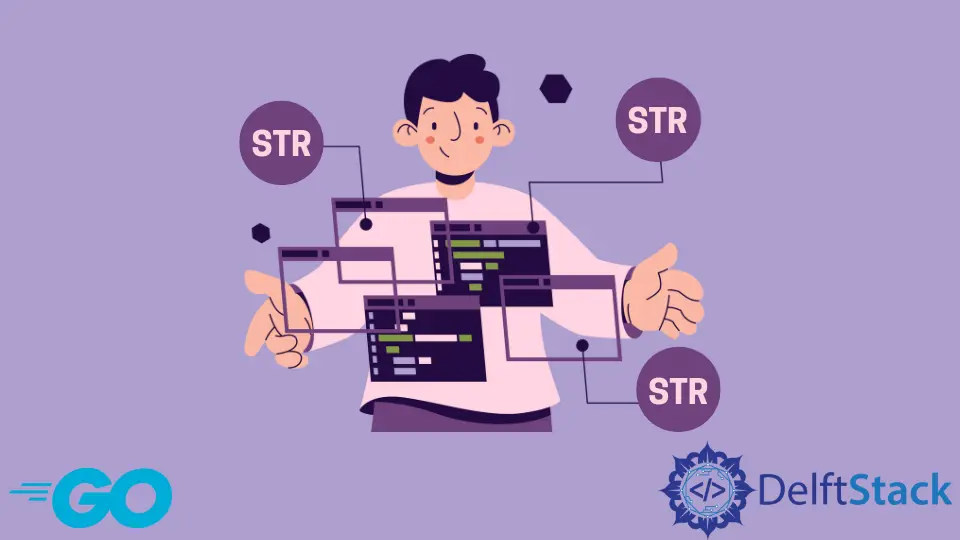
Go には、変数の値を文字列内のプレースホルダーに置き換える多くの文字列補間メソッドがあります。
Go の指定子
指定子 | 説明 |
---|---|
%s |
文字列を印刷する |
%d |
整数を出力する |
%v |
定義された構造体のすべての要素の値を出力します |
%+v |
構造体で指定されたすべての要素の名前と値を出力します |
fmt.Sprintf()
メソッドを使用する
package main
import (
"fmt"
"io"
"os"
)
func main() {
const string, val = "Hello,", "GoLang is Fun"
output := fmt.Sprintf("%s World! %s.\n", string, val)
io.WriteString(os.Stdout, output)
}
出力:
Hello, World! GoLang is Fun.
この方法を使用するには、fmt
パッケージをインポートする必要があることに注意してください。
Go 指定子テーブルを使用する
package main
import "fmt"
func main() {
string := "Go language was designed at %s in %d."
place := "Google"
year := 2007
output := fmt.Sprintf(string, place, year)
fmt.Println(output)
}
出力:
Go language was designed at Google in 2007.
上記のコード スニペットは、補間式を含む文字列リテラルを表示します。
fmt.Printf()
メソッドを使用する
package main
import "fmt"
func square(i int) int {
return i*i
}
func main() {
fmt.Printf("Square of 2 is %v\n", square(2))
}
出力:
Square of 2 is 4
上記のコードでは、出力行コマンドで文字列を直接補間しています。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn