How to Format a String Without Printing in Golang
Jay Singh
Feb 02, 2024
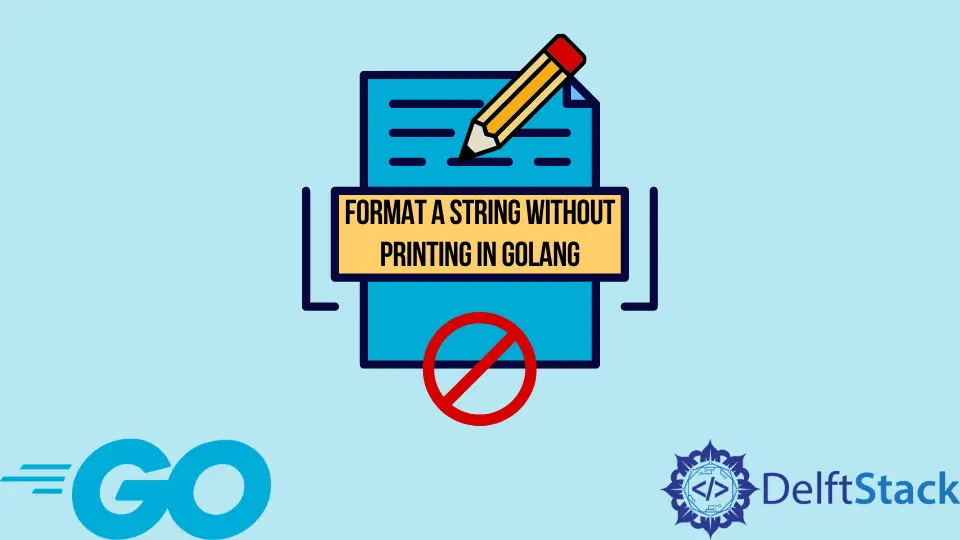
The Printf
is arguably the most common implementation of any variable formatted as a string. Golang has a simple technique of formatting a string without printing it.
The Sprintf()
function of the fmt
package in Go can be used to do this. The sole difference between Printf()
& Sprintf()
is Printf()
formats and prints the message, whereas Sprintf()
merely formats the message.
Use the Sprintf()
Function to Format a String in Golang
The formatting functions format the string based on the format specifiers sent to them.
package main
import (
"fmt"
)
func main() {
name := "Jay"
age := 23
res := fmt.Sprintf("%s is %d years old", name, age)
fmt.Println(res)
}
Output:
Jay is 23 years old
Another example is the sprintf()
method in the fmt
package formats a string and returns the output string.
package main
import (
"fmt"
)
func main() {
name := "Jay"
country := "India"
str := fmt.Sprintf("%s is from %s\n", name, country)
fmt.Println(str)
name = "Mike"
age := 20
str = fmt.Sprintf("%s is %d\n", name, age)
fmt.Println(str)
}
Output:
Jay is from India
Mike is 20
This last example demonstrates how to rearrange the format specifiers.
package main
import (
"fmt"
)
func main() {
a := 2
b := 3
c := 4
res := fmt.Sprintf("There are %d grapes, %d bananas, and %d strawberries.", a, b, c)
fmt.Println(res)
}
Output:
There are 2 grapes, 3 bananas, and 4 strawberries.