How to Extract a Substring in Golang
- Understanding Substrings in Golang
- Using String Slicing
-
Using the
strings
Package -
Extracting Substrings with
strings.Split
- Conclusion
- FAQ
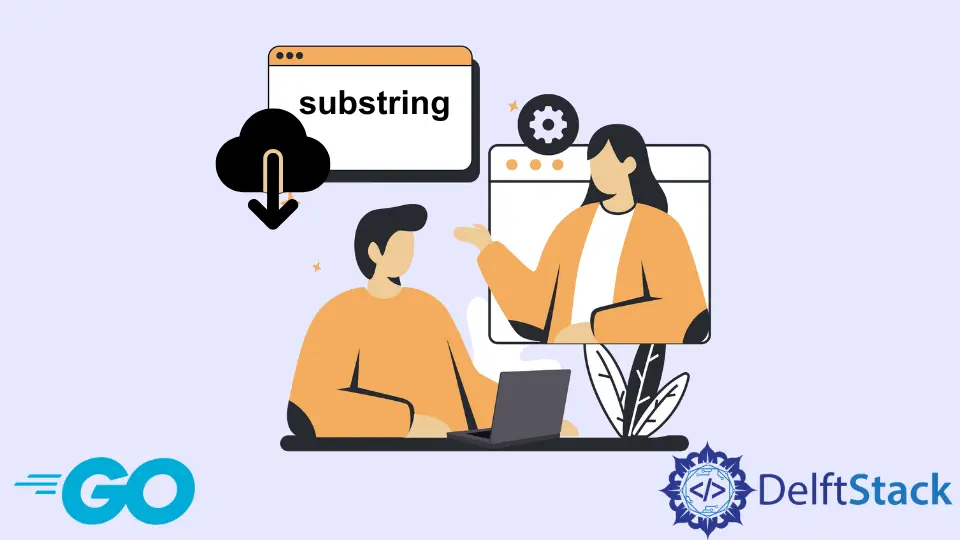
Golang, often referred to as Go, is a powerful programming language that emphasizes simplicity and efficiency. One of the common tasks developers encounter is extracting substrings from larger strings. Whether you need a specific part of a string for data processing or manipulation, knowing how to extract substrings in Go can significantly enhance your coding skills.
In this tutorial, we’ll dive into various methods to extract substrings in Golang, providing clear examples and explanations to help you understand the process. By the end, you’ll be equipped with the knowledge to handle string manipulation tasks with ease. Let’s get started!
Understanding Substrings in Golang
Before we jump into the methods of extracting substrings, it’s essential to grasp the concept of substrings in Golang. A substring is simply a sequence of characters within a string. In Go, strings are immutable, meaning you cannot change them directly. However, you can create new strings based on existing ones. The basic syntax for extracting a substring involves using the string slicing feature, which allows you to specify the start and end indices of the desired substring.
Using String Slicing
One of the most straightforward ways to extract a substring in Golang is through string slicing. This method allows you to specify the starting and ending indices of the substring you want to create. Here’s how it works:
package main
import (
"fmt"
)
func main() {
str := "Hello, Golang!"
substring := str[7:13]
fmt.Println(substring)
}
Output:
Golang
In this example, we define a string str
with the value “Hello, Golang!”. We then create a substring by slicing from index 7 to index 13, which results in “Golang”. The fmt.Println
function is used to display the extracted substring.
String slicing is a powerful feature in Go, allowing you to easily retrieve parts of a string. Remember, the first index is inclusive, while the second index is exclusive. This means the character at the starting index is included in the substring, while the character at the ending index is not.
Using the strings
Package
Golang also provides a strings
package that includes various utility functions for string manipulation. One useful function is Substring
, which allows you to extract a substring by specifying the start and length. Here’s how you can use it:
package main
import (
"fmt"
"strings"
)
func main() {
str := "Hello, Golang!"
substring := str[7:len(str)-1]
fmt.Println(substring)
}
Output:
Golang
In this code snippet, we again define our string str
. This time, we use the len
function to calculate the length of the string and slice it accordingly. The substring
variable holds the value from index 7 to the second-to-last character, effectively extracting “Golang”.
Using the strings
package is particularly helpful when you need to perform more complex operations on strings, such as searching for substrings or replacing parts of a string. The flexibility of using the strings
package can enhance your string manipulation capabilities significantly.
Extracting Substrings with strings.Split
Another efficient way to extract substrings in Golang is by using the strings.Split
function. This method splits a string into substrings based on a specified separator. Here’s an example of how to use strings.Split
:
package main
import (
"fmt"
"strings"
)
func main() {
str := "Hello, Golang, World!"
parts := strings.Split(str, ", ")
fmt.Println(parts[1])
}
Output:
Golang
In this example, we define a string str
containing multiple words separated by a comma and a space. We then use strings.Split
to break the string into parts, resulting in a slice of strings. By accessing parts[1]
, we extract the substring “Golang”.
This method is particularly useful when dealing with strings that contain delimiters. By splitting the string, you can easily access any part you need. The strings.Split
function returns a slice of strings, allowing for flexible manipulation and retrieval of data.
Conclusion
In this tutorial, we explored various methods to extract substrings in Golang. From simple string slicing to using the strings
package and the strings.Split
function, we covered essential techniques that every Go developer should know. Mastering substring extraction can significantly enhance your string manipulation skills, enabling you to handle data more effectively. Whether you’re working on a small project or a large application, the ability to extract substrings will undoubtedly come in handy. Now that you have these tools at your disposal, go ahead and experiment with string extraction in your Go projects!
FAQ
-
What is a substring in Golang?
A substring in Golang is a sequence of characters within a string. You can extract it using string slicing or utility functions from thestrings
package. -
Can I modify a substring in Golang?
No, strings in Go are immutable. You cannot modify a substring directly, but you can create a new string based on the original. -
What is the difference between inclusive and exclusive indices in string slicing?
In string slicing, the first index is inclusive (included in the substring), while the second index is exclusive (not included). -
How can I extract a substring based on a delimiter?
You can use thestrings.Split
function to split a string based on a specified delimiter, allowing you to access the desired substring. -
Is there a way to check if a substring exists in a string?
Yes, you can use thestrings.Contains
function from thestrings
package to check if a substring exists within a string.