How to Sort a List by a Field in C#
- Import the Required Libraries
- Define a Sample Class
- Create a Sample Data
-
Use the
Sort()
Method to Sort a List by Field in C# -
Use the
OrderBy()
Method to Sort a List by Field inC#
-
Using a Custom
IComparer<T>
Implementation to Sort a List by Field in C# -
Use the
Comparison<T>
With theSort()
Method to Sort a List by Field in C# - Conclusion
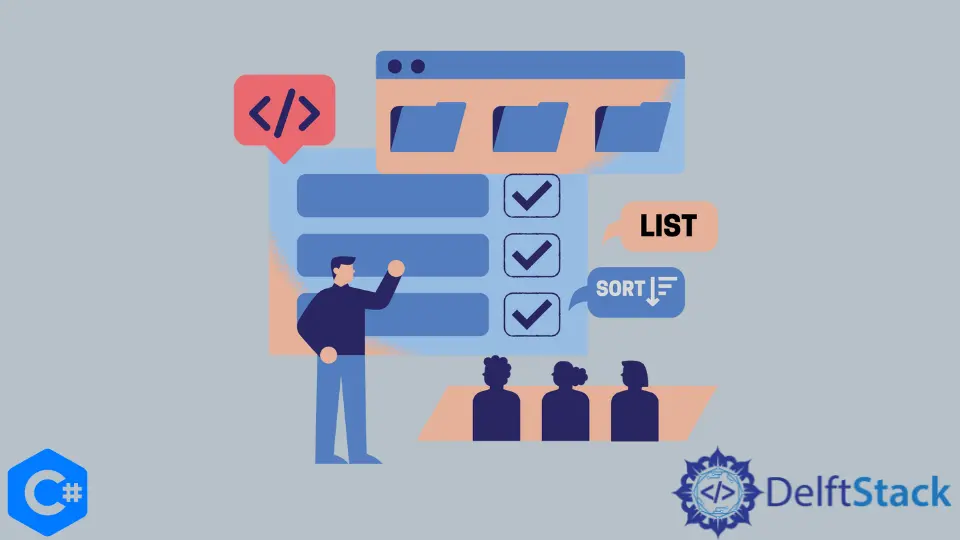
Sorting data is a common task in programming, and C# provides various ways to do it. In this guide, we’ll explore two primary methods for sorting lists in C#: using the Sort()
function and LINQ’s OrderBy()
keyword.
Additionally, we’ll discuss more advanced techniques involving custom comparers and delegates.
Import the Required Libraries
First, we need to import the necessary libraries to use the functions we’ll be using in our example:
using System;
using System.Linq;
using System.Collections.Generic;
Define a Sample Class
We then create a class called PersonData
. This class has defined a variable called name
, with the datatype string with its constructor and a method that will convert an input into a string value and return it:
public class PersonData {
public string name;
public PersonData(string name) {
this.name = name;
}
public override string ToString() {
return name;
}
}
Create a Sample Data
Now, let’s declare five variables of the PersonData
datatype and assign them some string values:
PersonData tom = new PersonData("Tom");
PersonData roger = new PersonData("Roger");
PersonData fred = new PersonData("Fred");
PersonData jack = new PersonData("Jack");
PersonData anna = new PersonData("Anna");
To work with this data, we’ll put them into a list called mergedList
:
List<PersonData> mergedList = new List<PersonData>() { tom, roger, fred, jack, anna };
Use the Sort()
Method to Sort a List by Field in C#
The simplest way to perform sorting in C# is by using the Sort()
method. To use it, we call this method on the list, as defined in the code block above, and provide a comparison function that determines how the values should be compared and sorted in ascending order.
The syntax sorts the mergedList
based on the name
property of the objects in ascending order.
Syntax:
mergedList.Sort((x, y) => {
int ret = String.Compare(x.name, y.name);
return ret;
});
The syntax mergedList.Sort((x, y) =>
sorts the mergedList
using a custom comparison logic that compares the name
property of two objects, x
and y
.
The ret
will be:
- Negative if
'x.name'
should come before'y.name'
in the sorted order. - Positive if
'x.name'
should come after'y.name'
in the sorted order. - Zero if
'x.name'
and'y.name'
have the same value in terms of sorting.
Let’s have an example to demonstrate how the method works.
Code:
using System;
using System.Linq;
using System.Collections.Generic;
public class PersonData {
public string name;
public PersonData(string name) {
this.name = name;
}
public override string ToString() {
return name;
}
}
public class SortList {
public static void Main() {
PersonData tom = new PersonData("Tom");
PersonData roger = new PersonData("Roger");
PersonData fred = new PersonData("Fred");
PersonData jack = new PersonData("Jack");
PersonData anna = new PersonData("Anna");
List<PersonData> mergedList = new List<PersonData>() { tom, roger, fred, jack, anna };
mergedList.Sort((x, y) => {
int ret = String.Compare(x.name, y.name);
return ret;
});
Console.WriteLine(String.Join(Environment.NewLine, mergedList));
}
}
First, we’ll create a constructor to initialize the 'name'
property of PersonData
objects. Next, we override the ToString()
method to provide a custom string representation for PersonData
objects.
We then create instances of PersonData
with different names (Tom, Roger, Fred, Jack, Anna
), create a list (mergedList
) and initialize it with PersonData
objects.
We sort the mergedList
based on the name
property using a custom comparison logic (mergedList.Sort((x, y)
). Lastly, we compare the name
property of two PersonData
objects, x
and y
.
The ret
will be:
- Negative if
'x.name'
should come before'y.name'
in the sorted order. - Positive if
'x.name'
should come after'y.name'
in the sorted order. - Zero if
'x.name'
and'y.name'
have the same value in terms of sorting.
This line of code return ret;
will return the result of the comparison to the Sort()
method for sorting. Lastly, we output the sorted list, joining elements with new lines (Console.WriteLine(String.Join(Environment.NewLine, mergedList));
).
Output:
Anna
Fred
Jack
Roger
Tom
After we’ve sorted the list in ascending order, we call the Reverse()
method to sort it in descending order.
mergedList.Reverse();
Output:
Tom
Roger
Jack
Fred
Anna
Now, the list is sorted in descending order.
Use the OrderBy()
Method to Sort a List by Field in C#
This is the alternate method for sorting a list in ascending order. We’ll call the list name created in the preceding code block by using the method OrderBy()
and giving an argument against which the values will be checked to sort it in ascending order.
Syntax:
List<PersonData> sortedList = mergedList.OrderBy(x => x.name).ToList();
The syntax above takes the mergedList
of PersonData
objects, sorts them in ascending order based on the name
property (OrderBy(x => x.name)
), and stores the sorted result in a new list called sortedList
. After this line of code executes, sortedList
will contain the PersonData
objects sorted by their name
property.
Let’s have an example to demonstrate how the method works.
Code:
using System;
using System.Linq;
using System.Collections.Generic;
public class PersonData {
public string name;
public PersonData(string name) {
this.name = name;
}
public override string ToString() {
return name;
}
}
public class SortList {
public static void Main() {
PersonData tom = new PersonData("Tom");
PersonData roger = new PersonData("Roger");
PersonData fred = new PersonData("Fred");
PersonData jack = new PersonData("Jack");
PersonData anna = new PersonData("Anna");
List<PersonData> mergedList = new List<PersonData>() { tom, roger, fred, jack, anna };
List<PersonData> sortedList = mergedList.OrderBy(x => x.name).ToList();
Console.WriteLine(String.Join(Environment.NewLine, sortedList));
}
}
First, we’ll create a constructor to initialize the 'name'
property of PersonData
objects. Next, we override the ToString()
method to provide a custom string representation for PersonData
objects.
Then, we define a class called SortList
and create instances of PersonData
with different names (Tom, Roger, Fred, Jack, Anna
), create a list (mergedList
) and initialize it with PersonData
objects. We sort the mergedList
based on the name
property using LINQ’s OrderBy()
method (mergedList.OrderBy(x => x.name).ToList();
), the lambda expression (x => x.name)
specifies the sorting criterion.
Lastly, we output the sorted list, joining elements with new lines ( Console.WriteLine(String.Join(Environment.NewLine, sortedList));
.
Output:
Anna
Fred
Jack
Roger
Tom
To sort this list in descending order, we call the Reverse()
function:
List<PersonData> sortedList = mergedList.OrderBy(x => x.name).ToList();
sortedList.Reverse();
Output:
Tom
Roger
Jack
Fred
Anna
Now, the list is sorted in descending order.
For more advanced sorting, such as sorting objects based on specific criteria, we can utilize custom comparers and delegates. These techniques provide more control over the sorting process.
In the next parts, we will see examples of using a custom IComparer<T>
implementation and the Comparison<T>
delegate for sorting.
Using a Custom IComparer<T>
Implementation to Sort a List by Field in C#
We can use a custom IComparer<T>
implementation to sort a list of objects by a field or criteria that we specify. This approach offers flexibility and control without altering the original class structure.
Code:
using System;
using System.Collections.Generic;
public class Person {
public string Name { get; set; }
public int Age { get; set; }
}
public class PersonAgeComparer : IComparer<Person> {
public int Compare(Person x, Person y) {
return x.Age.CompareTo(y.Age);
}
}
public class Program {
public static void Main() {
List<Person> people = new List<Person> {
new Person { Name = "Alice", Age = 30 },
new Person { Name = "Bob", Age = 25 },
new Person { Name = "Charlie", Age = 35 },
};
IComparer<Person> customComparer = new PersonAgeComparer();
people.Sort(customComparer);
foreach (var person in people) {
Console.WriteLine($"Name: {person.Name}, Age: {person.Age}", Environment.NewLine);
}
}
}
We define a class called Person
with properties Name
and Age
. We create a custom comparer class for comparing Person
objects based on their Age
property.
Then, we implement the Compare()
method required by the IComparer<T>
interface. Inside the Compare()
method, we compare the Age
property of two Person
objects (Compare(Person x, Person y)
).
Inside the main()
method, we create a list of Person
objects (List<Person> people = new List<Person>
) and populate with three Person
instances, each with a name
and age
. Then, we create an instance of the custom PersonAgeComparer
(IComparer<Person> customComparer = new PersonAgeComparer();
).
Next, we sort the people
list using the custom comparer (customComparer
). This means the list will be sorted based on the Age
property of each Person
object.
Finally, we utilize a foreach
loop to iterate through the sorted people
list and print the names and ages of each person.
Output:
Name: Bob, Age: 25
Name: Alice, Age: 30
Name: Charlie, Age: 35
The output shows the people
list sorted by age in ascending order, with the names and ages of each person displayed.
Use the Comparison<T>
With the Sort()
Method to Sort a List by Field in C#
We can use the Comparison<T>
delegate with the List.Sort()
method to sort a list by a specific field. This approach allows us to provide custom comparison logic without creating a separate comparer class.
Example:
using System;
using System.Collections.Generic;
public class Person {
public string Name { get; set; }
public int Age { get; set; }
}
public class Program {
public static void Main() {
List<Person> people = new List<Person> {
new Person { Name = "Alice", Age = 35 },
new Person { Name = "Bob", Age = 20 },
new Person { Name = "Charlie", Age = 30 },
};
Comparison<Person> ageComparison = (p1, p2) => p1.Age.CompareTo(p2.Age);
people.Sort(ageComparison);
foreach (var person in people) {
Console.WriteLine($"Name: {person.Name}, Age: {person.Age}", Environment.NewLine);
}
}
}
In this code, a Person
class is defined with Name
and Age
properties. In the Main()
method, a list of Person
objects named people
is created and populated with three Person
instances, each with a name
and age
.
A custom comparison delegate ageComparison
is defined using a lambda expression(Comparison<Person> ageComparison = (p1, p2) => p1.Age.CompareTo(p2.Age);
). This delegate compares two Person
objects, p1
and p2
, based on their Age
property using the CompareTo()
method, and this lambda expression effectively defines a custom sorting criterion based on age.
The Sort()
method is used to sort the people
list. The ageComparison
delegate is passed as an argument to specify the custom sorting criterion so that the list will be sorted by age in ascending order.
Finally, we create a foreach
loop to iterate through the sorted people
list and print the names and ages of each person.
Output:
Name: Bob, Age: 20
Name: Charlie, Age: 30
Name: Alice, Age: 35
The output displays the people
list sorted by age in ascending order, with the names and ages of each person displayed.
Conclusion
Sorting is a fundamental operation in programming, and mastering these techniques will enhance your ability to work with data effectively in C#. Whether you need a simple ascending sort or more complex sorting logic, C# provides the tools you need to get the job done.
This article should help you get started with sorting lists in C# more easily.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn