How to Read Integer From Console in C#
-
Read Integer Values From Console in C# Using the
Console.ReadLine()
andint.Parse()
Methods -
Read Integer Values From Console in C# Using the
Console.ReadLine()
andint.TryParse()
Methods -
Read Integer Values From Console in C# Using the
Console.ReadLine()
andConvert.ToInt32()
Methods -
Read Integer Values From Console in C# Using the
Console.Read()
Method -
Read Integer Values From Console in C# Using the
Console.ReadKey()
Method - Conclusion
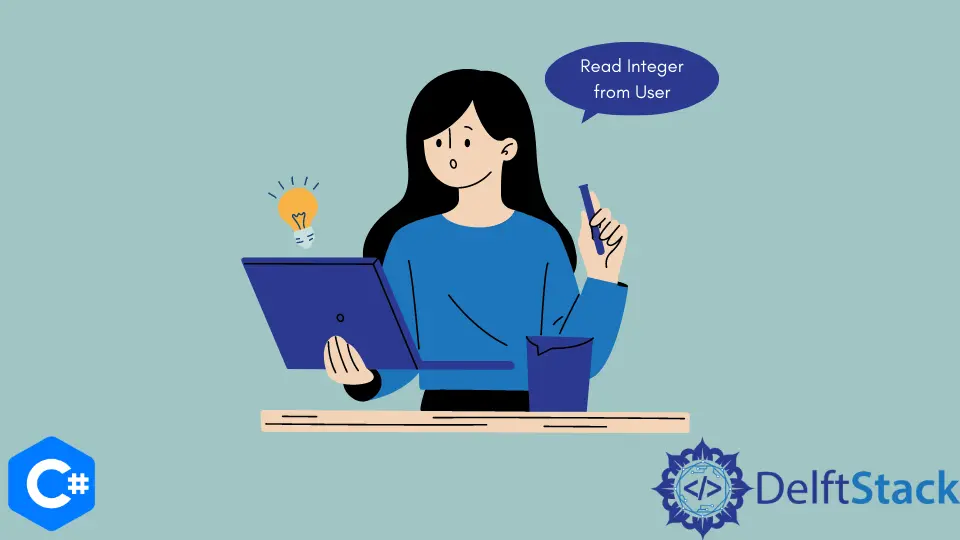
In C#, interacting with the console to obtain user input is a fundamental skill. This is particularly true when working with integer values, as efficient handling of user input is essential for robust and user-friendly applications.
This article explores various methods for reading integer values from the console in C#, providing insights into when and how to use each approach.
Read Integer Values From Console in C# Using the Console.ReadLine()
and int.Parse()
Methods
The Console.ReadLine()
method reads a line of text from the standard input, typically the console. By default, it returns the input as a string.
However, when we need to obtain an integer from the user, we use the int.Parse()
method. This method is part of the System
namespace in C# and is specifically designed for converting string representations of numbers to their integer equivalents.
The basic syntax is as follows:
int result = int.Parse(inputString);
Here, inputString
is the string we obtain from the console, and result
is the variable that will store the parsed integer value. If the string cannot be converted to an integer, a FormatException
will be thrown.
Let’s dive into a complete working example:
using System;
namespace ReadIntegerExample {
class Program {
static void Main(string[] args) {
Console.Write("Enter an integer: ");
string input = Console.ReadLine();
try {
int parsedValue = int.Parse(input);
Console.WriteLine($"You entered: {parsedValue}");
} catch (FormatException) {
Console.WriteLine("Invalid input. Please enter a valid integer.");
}
}
}
}
In this example, we start by prompting the user to enter an integer using Console.Write("Enter an integer: ")
. The input is then read as a string using Console.ReadLine()
and stored in the input
variable.
Inside the try
block, we use int.Parse(input)
to convert the string to an integer. If the conversion is successful, the parsed value is displayed using the Console.WriteLine($"You entered: {parsedValue}")
.
However, if the input string is not a valid integer, a FormatException
is caught in the catch
block, and an error message is displayed.
Let’s consider an example where the user enters a valid integer:
Enter an integer: 42
You entered: 42
In this scenario, the program successfully parses the input string 42
to the integer value 42
and displays the result.
Keep in mind that int.Parse()
does not handle invalid input gracefully on its own. Including exception handling, as demonstrated in the provided example, ensures a more robust and user-friendly application.
Read Integer Values From Console in C# Using the Console.ReadLine()
and int.TryParse()
Methods
Another method we can use for reading integers from the console is the int.TryParse()
method.
Unlike int.Parse()
, this method provides a more forgiving approach, allowing us to check if the input is a valid integer without throwing exceptions. This is particularly useful when user input might be unpredictable.
The int.TryParse()
method attempts to convert a string representation of a number to its integer equivalent. Its syntax looks like this:
bool success = int.TryParse(inputString, out int result);
Here, inputString
is the string we obtain from the console, result
is the variable that will store the parsed integer value, and success
is a boolean indicating whether the conversion was successful. If successful, result
holds the parsed value; otherwise, it remains 0
.
Let’s explore a complete working example:
using System;
namespace ReadIntegerExample {
class Program {
static void Main(string[] args) {
Console.Write("Enter an integer: ");
string input = Console.ReadLine();
if (int.TryParse(input, out int parsedValue)) {
Console.WriteLine($"You entered: {parsedValue}");
} else {
Console.WriteLine("Invalid input. Please enter a valid integer.");
}
}
}
}
Similar to the previous example, we prompt the user to enter an integer using Console.Write("Enter an integer: ")
and read the input as a string with Console.ReadLine()
. However, this time, we use int.TryParse(input, out int parsedValue)
to attempt the conversion.
If the conversion is successful, the code inside the if
block executes, displaying the parsed value using Console.WriteLine($"You entered: {parsedValue}")
. If the input is not a valid integer, the code within the else
block is executed, providing an error message.
Let’s consider an example where the user enters a valid integer:
Enter an integer: 56
You entered: 56
In this scenario, the program successfully parses the input string 56
to the integer value 56
and displays the result.
Read Integer Values From Console in C# Using the Console.ReadLine()
and Convert.ToInt32()
Methods
Continuing our exploration of reading integer values from the console in C#, we now turn our attention to another method – Convert.ToInt32()
. This method is part of the Convert
class in C# and provides a convenient way to convert a specified string representation of a number to an equivalent 32-bit signed integer.
The syntax is as follows:
int result = Convert.ToInt32(inputString);
Here, inputString
is the string obtained from the console, and result
is the variable that will store the parsed integer value. If the string cannot be converted to an integer, a FormatException
will be thrown, similar to the behavior of int.Parse()
.
Let’s delve into a complete working example:
using System;
namespace ReadIntegerExample {
class Program {
static void Main(string[] args) {
Console.Write("Enter an integer: ");
string input = Console.ReadLine();
try {
int parsedValue = Convert.ToInt32(input);
Console.WriteLine($"You entered: {parsedValue}");
} catch (FormatException) {
Console.WriteLine("Invalid input. Please enter a valid integer.");
}
}
}
}
In this example, the user is prompted to enter an integer using Console.Write("Enter an integer: ")
, and the input is read as a string with Console.ReadLine()
. The Convert.ToInt32(input)
method is then used to attempt the conversion.
Inside the try
block, if the conversion is successful, the parsed value is displayed using Console.WriteLine($"You entered: {parsedValue}")
. On the other hand, if the input string is not a valid integer, a FormatException
is caught in the catch
block, and an error message is displayed.
Consider an example where the user enters a valid integer:
Enter an integer: 73
You entered: 73
In this scenario, the program successfully parses the input string 73
to the integer value 73
and displays the result.
Read Integer Values From Console in C# Using the Console.Read()
Method
Let’s see a different approach for reading integer values from the console in C# – using Console.Read()
. Unlike the previous methods, Console.Read()
reads a single character from the console, making it ideal when we expect single-digit integer input.
The Console.Read()
method reads the next character from the standard input stream (console) and returns its ASCII value as an integer. To use it for reading an integer, we need to convert the character to its corresponding numeric value.
The basic syntax involves storing the result in an integer variable:
int inputValue = Console.Read();
Here, inputValue
is the variable that will store the ASCII value of the character read. To obtain the actual numeric value, we can subtract the ASCII value of 0
, as digits are represented in a contiguous block in ASCII.
Let’s explore a complete working example:
using System;
namespace ReadIntegerExample {
class Program {
static void Main(string[] args) {
Console.Write("Enter a single-digit integer: ");
int inputValue = Console.Read();
int numericValue = inputValue - '0';
Console.WriteLine($"You entered: {numericValue}");
}
}
}
In this example, the user is prompted to enter a single-digit integer using the line Console.Write("Enter a single-digit integer: ")
. The Console.Read()
method is then used to read a single character from the console.
To convert the ASCII value of the character to its numeric value, we subtract the ASCII value of 0
. This is possible because digits are represented in a contiguous block in ASCII.
The resulting numeric value is stored in the numericValue
variable, and it is then displayed using Console.WriteLine($"You entered: {numericValue}")
.
Consider an example where the user enters the digit 5
:
Enter a single-digit integer: 5
You entered: 5
In this scenario, the program successfully reads the single-digit integer 5
and displays the result after converting it to a numeric value.
While Console.Read()
is limited to reading single characters, it can be a concise option for scenarios where single-digit integer input is expected. Understanding the ASCII representation and converting it to its numeric value enables us to work with this method effectively.
However, it’s crucial to note its limitations and use cases to ensure appropriate application in different scenarios.
Read Integer Values From Console in C# Using the Console.ReadKey()
Method
Similar to Console.Read()
, the Console.ReadKey()
method allows us to capture a single key press from the console. Unlike Console.Read()
, Console.ReadKey()
provides more information about the pressed key, allowing us to handle non-digit inputs more gracefully.
The Console.ReadKey()
method reads the next key press from the standard input stream (console) and returns a ConsoleKeyInfo
object. This object contains information about the key pressed, including its character representation.
To use it for reading an integer, we can access the KeyChar
property of the ConsoleKeyInfo
object and convert it to its corresponding numeric value.
Basic syntax involves:
ConsoleKeyInfo key = Console.ReadKey();
char inputChar = key.KeyChar;
Here, key
is the ConsoleKeyInfo
object, and inputChar
is the character representation of the pressed key. To convert it to an integer, we can use int.TryParse()
or directly convert the character to its numeric value.
Let’s explore a complete working example:
using System;
namespace ReadIntegerExample {
class Program {
static void Main(string[] args) {
Console.Write("Enter a digit: ");
ConsoleKeyInfo key = Console.ReadKey();
char inputChar = key.KeyChar;
if (int.TryParse(inputChar.ToString(), out int numericValue)) {
Console.WriteLine($"\nYou entered: {numericValue}");
} else {
Console.WriteLine("\nInvalid input. Please enter a valid digit.");
}
}
}
}
In this example, the user is prompted to enter a digit using Console.Write("Enter a digit: ")
. The Console.ReadKey()
method is then used to capture the key press, and the character representation is obtained using key.KeyChar
.
To convert the character to an integer, we use int.TryParse(inputChar.ToString(), out int numericValue)
.
Inside the if
block, if the conversion is successful, the program displays the parsed numeric value using Console.WriteLine($"\nYou entered: {numericValue}")
. If the input is not a valid digit, the else
block executes, providing an error message.
Consider an example where the user enters the digit 7
:
Enter a digit: 7
You entered: 7
In this scenario, the program successfully captures the key press 7
and displays the result after converting it to a numeric value.
Conclusion
In C# programming, reading integer values from the console involves choosing the right method for the specific requirements of the application. Whether it’s the precise but exception-prone int.Parse()
, the forgiving int.TryParse()
, or alternative methods like Convert.ToInt32()
, Console.Read()
, and Console.ReadKey()
, each approach offers unique advantages.
By understanding the nuances of these methods, developers can create robust, interactive applications that efficiently handle user input.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn