在 C# 中从控制台读取整数
-
使用 C# 中的
int.Parse()
方法从控制台读取整数 -
使用 C# 中的
int.TryParse()
方法从控制台读取整数 -
使用 C# 中的
Convert.ToInt32()
方法从控制台读取整数
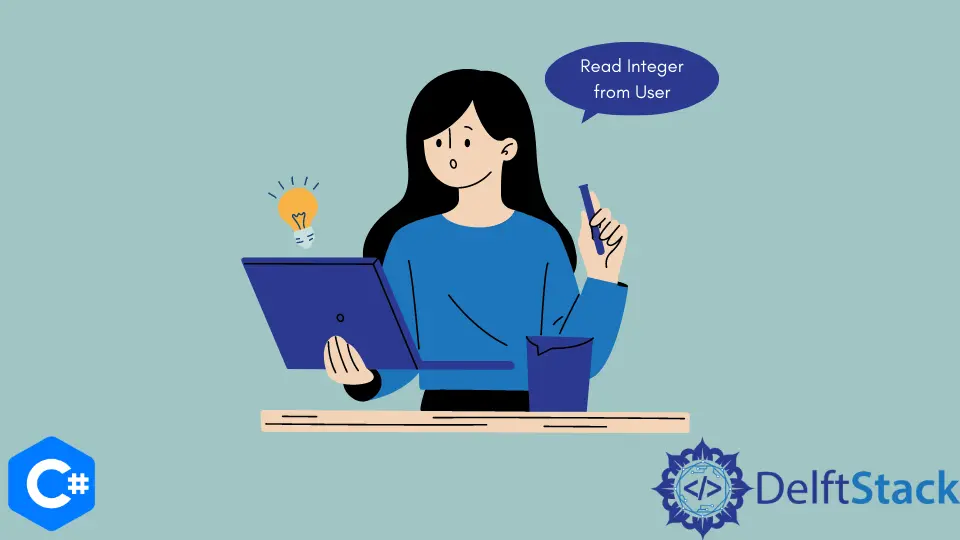
本教程将讨论从 C# 控制台读取整数值的方法。
使用 C# 中的 int.Parse()
方法从控制台读取整数
默认情况下,C# 中的 Console.ReadLine()
方法从控制台读取字符串值。如果要从控制台读取整数值,则必须首先在字符串中输入整数值,然后将其转换为整数。然后,使用 int.Parse()
方法将字符串转换为 C# 中的整数值。
using System;
namespace read_integer {
class Program {
static void method1() {}
static void Main(string[] args) {
Console.WriteLine("Enter a number");
int num = int.Parse(Console.ReadLine());
Console.WriteLine("You entered {0}", num);
}
}
}
输出:
Enter a number
11
You entered 11
在上面的代码中,我们使用 C# 中的 int.Parse()
方法从控制台读取了整数变量 num
。我们首先使用 Console.ReadLine()
方法以字符串形式获取输入,然后使用 int.Parse()
方法将其转换为整数。
使用 C# 中的 int.TryParse()
方法从控制台读取整数
C# 中的 [int.TryParse()
方法)还可以将字符串值转换为整数值。唯一的区别是 int.TryParse()
方法具有布尔返回类型,并且如果字符串中没有整数值,则返回 false。int.TryParse()
方法检查输入是否为有效整数。如果有人输入任何无效值,我们的程序将不会引发异常。
using System;
namespace read_integer {
class Program {
static void method2() {}
static void Main(string[] args) {
Console.WriteLine("Enter a number");
if (!int.TryParse(Console.ReadLine(), out int num)) {
Console.WriteLine("Invalid value entered");
} else {
Console.WriteLine("You entered {0}", num);
}
}
}
}
输出:
Enter a number
12
You entered 12
在上面的代码中,我们使用 C# 中的 int.TryParse()
方法从控制台读取了整数变量 num
。我们使用 int.TryParse()
方法检查用户是否输入了有效的整数。如果该值无效,程序将显示一条错误消息。如果该值有效,则程序将该值存储在整数变量 num
中,并将其显示在输出中。这种方法比以前的方法更好,因为它为我们提供了一种处理意外用户值的方法。当我们不确定用户输入时,应首选 int.TryParse()
方法而不是 int.TryParse()
方法。
使用 C# 中的 Convert.ToInt32()
方法从控制台读取整数
Convert
类用于在 C# 中的不同基础数据类型之间进行转换。由于字符串和整数都是基本数据类型,因此可以使用 Convert
类将字符串变量转换为整数变量。Convert.ToInt32()
方法是在 C# 中将字符串值转换为整数值的另一种方法。我们可以使用 Console.ReadLine()
方法从控制台读取字符串,然后使用 Convert.ToInt32()
方法将其转换为整数值。
using System;
namespace read_integer {
class Program {
static void method3() {}
static void Main(string[] args) {
Console.WriteLine("Enter a number");
int num = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("You entered {0}", num);
}
}
}
输出:
Enter a number
44
You entered 44
在上面的代码中,我们使用 C# 中的 Convert.ToInt32()
方法从控制台读取了整数变量 num
。我们首先使用 Console.ReadLine()
方法以字符串形式获取输入,然后使用 Convert.ToInt32()
方法将其转换为整数。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn