How to Clear the Console Window in C#
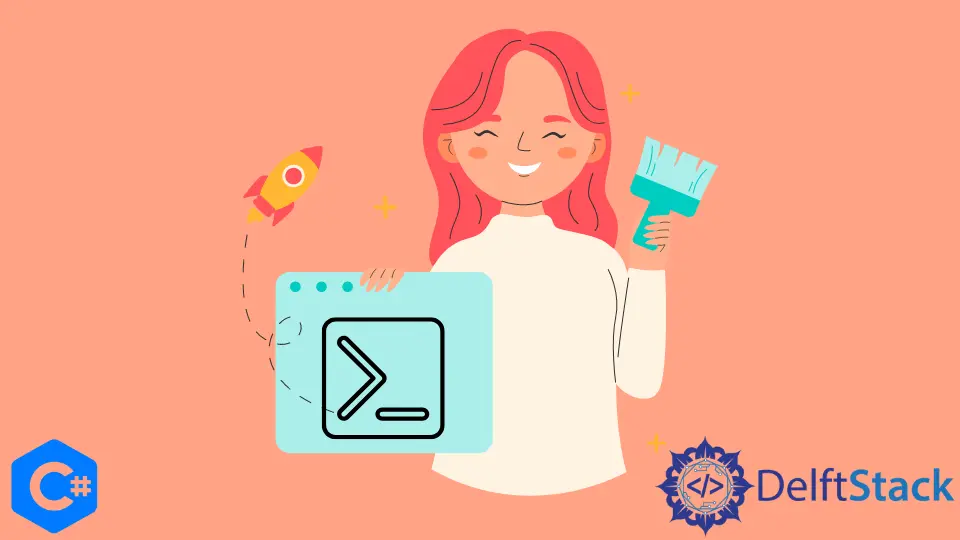
We will demonstrate how to clear the console in the C# project. We will provide the code snippets to understand the concept better.
Use the Clear()
Method to Clear the Console Window in C#
Sometimes you need to clear the console window while executing. The Console
class in C# provides a Clear()
method.
The definition of this method is as follows:
public static void Clear();
As this method is static, you can call it by its name. In case an I/O error occurs, this method throws the IOException.
The Clear()
method removes all the content on the console window and clears the screen and the console buffer.
Let us create an example project to depict the Clear()
method. In this example, we will create a for
loop to iterate ten times and then ask the user to enter any key.
As the user presses any key from the keyboard, Console.Clear()
will be called, the screen will be cleared, and the second for
loop will be executed.
Program 1:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearConsole {
class Program {
static void Main(string[] args) {
for (int i = 0; i < 10; i++) {
Console.WriteLine("count: " + i);
}
Console.WriteLine("Note: We are going to make the screen clear. Press any key to continue.");
Console.ReadKey();
Console.Clear();
for (int i = 10; i < 20; i++) {
Console.WriteLine("count: " + i);
}
Console.ReadKey();
}
}
}
Output:
count: 0
count: 1
count: 2
count: 3
count: 4
count: 5
count: 6
count: 7
count: 8
count: 9
Note: We are going to make the screen clear. Press any key to continue.
As you press any key from the keyboard, the screen will be made clear, and then the output on the console will be:
count: 10
count: 11
count: 12
count: 13
count: 14
count: 15
count: 16
count: 17
count: 18
count: 19
Following is another example program depicting the Clear()
method. This code snippet will show some text on the console window, Console.Clear()
will be invoked, and the console will be cleared after 1 second.
Some text will be shown later on the console window.
Program 2:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearConsole {
class Program {
static void Main(string[] args) {
string welcome = "Hi, Welcome! \nI am ClearConsole. What's your name?";
foreach (char c in welcome) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
Console.WriteLine();
string name = Console.ReadLine();
string thanks = "Nice to see you " + name;
Console.WriteLine();
foreach (char c in thanks) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
string note = "You are going to experience Clear() method.";
Console.WriteLine();
foreach (char c in note) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
System.Threading.Thread.Sleep(1000);
Console.Clear();
string cleared = "Cleared the screen. \nHope you enjoyed this method. \nGood Bye!";
foreach (char c in cleared) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
Console.ReadKey();
}
}
}
Output:
Hi, Welcome!
I am ClearConsole. What's your name?
human
Nice to see you human
You are going to experience Clear() method.
Firstly, this text will be shown as the output on your console. After 1 second, the console will be cleared, and the subsequent outcome will be:
Cleared the screen.
Hope you enjoyed this method.
Good Bye!