How to Exit Console Application in C#
-
Use the
return
Statement to Exit a Console Application in C# -
Use the
Environment.Exit()
Method to Exit a Console Application in C# - Throw an Exception to Exit a Console Application in C#
- Use Ctrl+C (Keyboard Interrupt) to Exit a Console Application in C#
- Use a Loop Break to Exit a Console Application in C#
- Conclusion
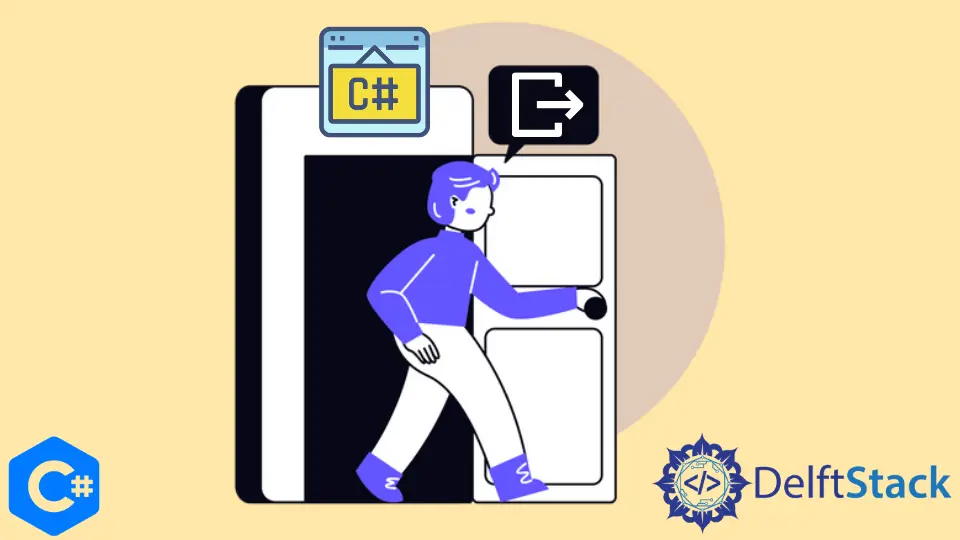
Exiting a console application in C# is a fundamental aspect of program control flow. Whether your application completes its task successfully or encounters an error condition, knowing how to exit properly ensures that your program behaves as expected.
This tutorial will discuss methods to exit a console application in C#. Each method has its purpose and considerations, allowing you to choose the most appropriate approach based on your specific requirements.
Use the return
Statement to Exit a Console Application in C#
If we want to exit our application, we can use the return
statement in C#. The return
statement ends the execution of a method and returns the control to the calling or the main
method.
Syntax:
return [expression];
return
: This is the keyword that indicates the intention to return a value from a method. It is used to exit the method and, optionally, pass a value back to the caller.expression
(optional): This is the value that is returned from the method. It must be of the same type as the return type specified in the method signature. If the method has a return type ofvoid
, then no value needs to be provided.
The return
statement allows for a controlled exit and can be useful when you want to indicate a specific exit code. We can use the return
statement inside the main()
function to end our console application’s execution.
The following code example shows us how to exit a console application with the return
statement in C#.
using System;
class Program {
static int Main() {
Console.WriteLine("Before Return");
return 0;
Console.WriteLine("After Return"); // This line will not be executed
}
}
The Main
method begins execution with Console.WriteLine("Before Return");
.
The line return 0;
instructs the program to exit with an exit code of 0
. Any code after this statement will not be executed.
Therefore, the line "After Return"
will not be printed to the console.
Output:
Before Return
The only disadvantage of using this method is that we cannot exit the application from any other function.
Use the Environment.Exit()
Method to Exit a Console Application in C#
The Environment.Exit()
method belongs to the System
namespace in C#. It allows you to instantly terminate a running application.
This method takes an integer parameter representing the exit code (where 0
typically indicates a successful exit). It can be used to convey information about the program’s termination status to the calling process.
Syntax:
Environment.Exit(exitCode);
exitCode
(optional): An integer that specifies the exit code. It is an indicator sent back to the calling process to inform it about the outcome of the program execution.
We can also use the Environment.Exit()
method to exit a console application in C#. This method immediately terminates the program, bypassing any cleanup or finalization code.
Example 1: Immediate Exit
using System;
class Program {
static void Main() {
Console.WriteLine("Before Exit");
Environment.Exit(0);
Console.WriteLine("After Exit"); // This line will not be executed
}
}
Output:
Before Exit
The program starts execution in the Main
method.
"Before Exit"
is printed on the console. Environment.Exit(0)
is called, specifying an exit code of 0
.
The program terminates instantly. As a result, the line Console.WriteLine("After Exit")
is skipped and not executed.
Example 2: Specifying an Exit Code
using System;
class Program {
static void Main() {
Console.WriteLine("An error occurred. Exiting...");
Environment.Exit(1);
}
}
Output:
An error occurred. Exiting...
The program begins execution in the Main
method. It displays a message indicating an error.
Environment.Exit(1)
is called with an exit code of 1
, indicating an error status. The program terminates, returning the exit code of 1
to the calling process.
The advantage of this method over the previous method is that we can exit the application from any function.
Throw an Exception to Exit a Console Application in C#
In C#, exceptions are objects that represent abnormal conditions that occur during the execution of a program. They provide a structured way to handle errors and other exceptional situations.
To throw an exception in C#, the throw
statement is used. This statement is followed by an instance of an exception class.
Syntax:
throw exception;
throw
: This is a keyword in C# that signals the occurrence of an exceptional condition or error in the program. Whenthrow
is used, it interrupts the normal flow of execution and transfers control to the nearest enclosing exception handler.exception
: This refers to an instance of an exception class. In C#, exceptions are represented as objects instantiated from classes that derive from theSystem.Exception
base class. These exception classes contain information about the type of error, a message describing the error, and, optionally, inner exceptions (exceptions that caused the current exception).
One effective way to exit a console application is by using exceptions. This method allows you to handle specific conditions or errors and then gracefully exit the program.
Example Code:
using System;
class Program {
static void Main() {
try {
// Check for exit condition
throw new ApplicationException("Exiting application.");
} catch (ApplicationException ex) {
Console.WriteLine(ex.Message);
}
}
}
Output:
Exiting application.
The program starts execution in the Main
method.
Within a try
block, it checks for a specific exit condition. If the condition is met, it throws an ApplicationException
with a descriptive message.
The exception is caught in the catch
block, where it prints the message and exits the program using Environment.Exit(1)
, indicating an error status.
Use Ctrl+C (Keyboard Interrupt) to Exit a Console Application in C#
Ctrl+C is a universally recognized keyboard shortcut for interrupting or terminating a running process in a console environment. When pressed, it sends an interrupt signal to the process currently running in the console, prompting it to exit gracefully.
In C#, you can handle the Ctrl+C signal using an event. Specifically, the Console.CancelKeyPress
event allows you to intercept the Ctrl+C signal and take appropriate action before the program exits.
Example Code:
using System;
class Program {
static bool exitFlag = false;
static void Main() {
Console.CancelKeyPress += new ConsoleCancelEventHandler(OnCancelKeyPress);
while (!exitFlag) {
// Perform program tasks
}
}
static void OnCancelKeyPress(object sender, ConsoleCancelEventArgs e) {
e.Cancel = true;
exitFlag = true;
}
}
This code sets up a console application to respond to a Ctrl+C keyboard interrupt. It declares a Boolean variable exitFlag
and sets it to false
.
The Main
method subscribes to the Console.CancelKeyPress
event, which is triggered when the user presses Ctrl+C. The program enters a while
loop, which continues executing as long as exitFlag
is false
.
When Ctrl+C is pressed, the OnCancelKeyPress
method is called. This method sets e.Cancel
to true
to prevent the default behavior of immediate termination, and it sets exitFlag
to true
. This indicates that the program should exit gracefully.
The program will continue running until the user presses Ctrl+C, at which point it will terminate.
Use a Loop Break to Exit a Console Application in C#
In C#, a loop break statement (break
) is used to immediately exit a loop, regardless of whether the loop’s condition has been met or not. It provides a way to prematurely terminate the loop and continue with the execution of the program.
In scenarios where you need to exit the application based on a condition, you can use a loop and break out of it.
Example Code:
using System;
class Program {
static void Main() {
Console.WriteLine("Press 'Q' to exit.");
while (true) {
ConsoleKeyInfo keyInfo = Console.ReadKey();
if (keyInfo.Key == ConsoleKey.Q) {
break; // Exit the loop if 'Q' is pressed
}
}
Console.WriteLine("Program has exited.");
}
}
In this example, the program displays a message instructing the user to press Q
to exit. It then enters an infinite loop using while(true)
.
Within the loop, it waits for a key press using Console.ReadKey()
. If the pressed key is Q
, the break
statement is executed, which immediately exits the loop.
The program then continues with the statement after the loop, printing "Program has exited"
. This demonstrates how a loop break can be used to exit a console application based on a specific condition.
Conclusion
Exiting a console application in C# is a critical aspect of program control flow. Knowing how to do it ensures your program behaves as expected.
In this tutorial, we explored various methods like the return
statement, the Environment.Exit()
method, throwing an exception, using keyboard interrupt (Ctrl + C) and loop break.
Understanding these techniques empowers you to manage program termination effectively, creating more robust and user-friendly console applications in C#. Choose the method that best fits your application’s needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn