How to Keep Console Open in C#
-
Keep Console Open With the
Console.ReadLine()
Method inC#
-
Keep Console Open With the
Console.Read()
Method inC#
-
Keep Console Open With the
Console.ReadKey()
Method inC#
-
Keep Console Open With the Ctrl + F5 Shortcut in
C#
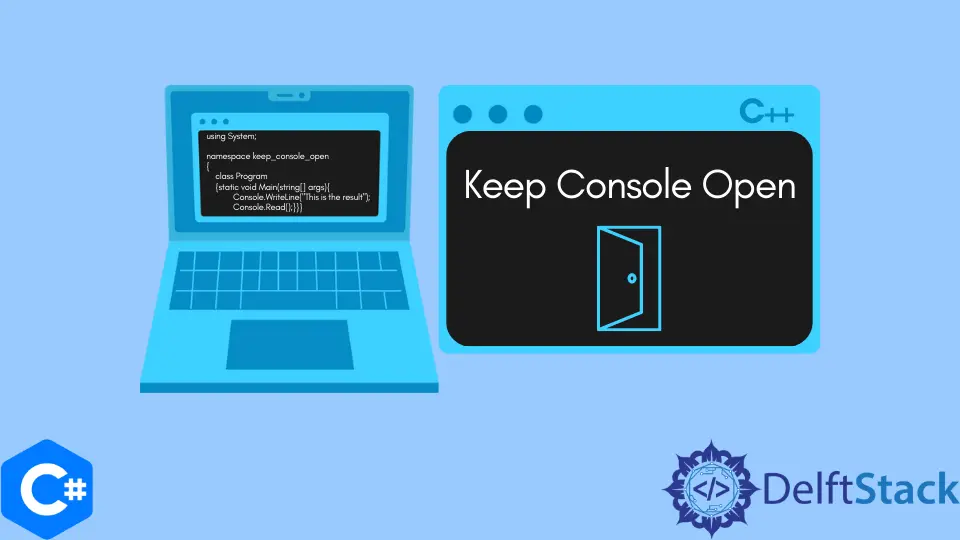
This tutorial will discuss methods to pause the console of Microsoft Visual Studio IDE in C#.
Keep Console Open With the Console.ReadLine()
Method in C#
The Console.ReadLine()
method gets the input as a string from the console in C#. The Console.ReadLine()
method reads a complete line or until the Enter key is pressed. The Console.ReadLine()
method can also be used to keep our console window open after displaying some output. We can write the Console.ReadLine()
method at the end of our code to keep our console window open.
using System;
namespace keep_console_open {
class Program {
static void Main(string[] args) {
Console.WriteLine("This is the result");
Console.ReadLine();
}
}
}
Output:
This is the result
In the above code, we keep our console window open by writing the Console.ReadLine()
method at the end of our code. The console window remains open until the Enter key is pressed. It is because the Console.ReadLine()
method reads a complete line from the console. We cannot close the console window by pressing any other key.
Keep Console Open With the Console.Read()
Method in C#
The Console.Read()
method is another method that can be used to keep our console window open after displaying some output. We can write the Console.Read()
method at the end of our code to keep our console window open.
using System;
namespace keep_console_open {
class Program {
static void Main(string[] args) {
Console.WriteLine("This is the result");
Console.Read();
}
}
}
Output:
This is the result
We keep our console open after displaying This is the result
by writing the Console.Read()
method at the end of our code. The console can only be resumed by pressing the Enter key, just like the previous example.
Keep Console Open With the Console.ReadKey()
Method in C#
The Console.ReadKey()
method) gets the input as a ConsoleKeyInfo
object from the console in C#. The Console.ReadKey()
method reads only a single key from the console. The Console.ReadKey()
method can also be used to keep our console window open after displaying some output. We can write the Console.ReadKey()
method at the end of our code to keep our console window open.
using System;
namespace keep_console_open {
class Program {
static void Main(string[] args) {
Console.WriteLine("This is the result");
Console.ReadKey();
}
}
}
Output:
This is the result
We keep our console open after displaying This is the result
by writing the Console.Read()
method at the end of our code. Unlike the previous two approaches, the console window can be closed by pressing any key this time.
Keep Console Open With the Ctrl + F5 Shortcut in C#
The disadvantage of using any of the approaches mentioned above is that we have to write a line of code at the end of our program. It can lead to many problems when extending our code in the future. The best approach for keeping our console window open after the execution of code is to run it with the Ctrl + F5 shortcut of the Microsoft Visual Studio IDE. Our program runs in debug mode when we run our code by clicking the start button in the Visual Studio IDE. If we want to run our code just like it does on languages like C and C++ after compilation, we have to also compile our code with the Ctrl + F5 shortcut.
using System;
namespace keep_console_open {
class Program {
static void Main(string[] args) {
Console.WriteLine("This is the result");
}
}
}
Output:
This is the result
We keep our console open after displaying This is the result
by compiling our code with the Ctrl + F5 shortcut of the Microsoft Visual Studio IDE. We do not have to write anything at the end of our code to keep our console window open.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn