C#에서 콘솔 창 지우기
Aimen Fatima
2023년10월12일
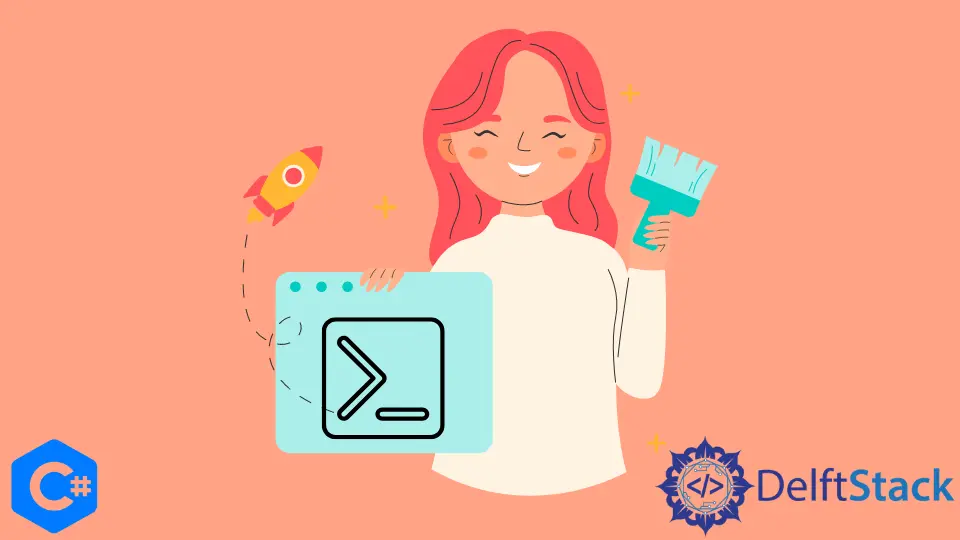
C# 프로젝트에서 콘솔을 지우는 방법을 시연합니다. 개념을 더 잘 이해할 수 있도록 코드 스니펫을 제공합니다.
Clear()
메서드를 사용하여 C#
에서 콘솔 창 지우기
때때로 실행하는 동안 콘솔 창을 지워야 합니다. C#의 Console
클래스는 Clear()
메서드를 제공합니다.
이 방법의 정의는 다음과 같습니다.
public static void Clear();
이 메서드는 정적이므로 이름으로 호출할 수 있습니다. I/O 오류가 발생한 경우 이 메소드는 IOException을 발생시킵니다.
Clear()
메서드는 콘솔 창의 모든 내용을 제거하고 화면과 콘솔 버퍼를 지웁니다.
Clear()
메서드를 설명하는 예제 프로젝트를 생성해 보겠습니다. 이 예에서는 for
루프를 만들어 열 번 반복한 다음 사용자에게 아무 키나 입력하도록 요청합니다.
사용자가 키보드에서 아무 키나 누르면 Console.Clear()
가 호출되고 화면이 지워지며 두 번째 for
루프가 실행됩니다.
프로그램 1:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearConsole {
class Program {
static void Main(string[] args) {
for (int i = 0; i < 10; i++) {
Console.WriteLine("count: " + i);
}
Console.WriteLine("Note: We are going to make the screen clear. Press any key to continue.");
Console.ReadKey();
Console.Clear();
for (int i = 10; i < 20; i++) {
Console.WriteLine("count: " + i);
}
Console.ReadKey();
}
}
}
출력:
count: 0
count: 1
count: 2
count: 3
count: 4
count: 5
count: 6
count: 7
count: 8
count: 9
Note: We are going to make the screen clear. Press any key to continue.
키보드에서 아무 키나 누르면 화면이 깨끗해지고 콘솔에 다음과 같이 출력됩니다.
count: 10
count: 11
count: 12
count: 13
count: 14
count: 15
count: 16
count: 17
count: 18
count: 19
다음은 Clear()
메서드를 설명하는 또 다른 예제 프로그램입니다. 이 코드 스니펫은 콘솔 창에 일부 텍스트를 표시하고 Console.Clear()
가 호출되고 콘솔이 1초 후에 지워집니다.
일부 텍스트는 나중에 콘솔 창에 표시됩니다.
프로그램 2:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearConsole {
class Program {
static void Main(string[] args) {
string welcome = "Hi, Welcome! \nI am ClearConsole. What's your name?";
foreach (char c in welcome) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
Console.WriteLine();
string name = Console.ReadLine();
string thanks = "Nice to see you " + name;
Console.WriteLine();
foreach (char c in thanks) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
string note = "You are going to experience Clear() method.";
Console.WriteLine();
foreach (char c in note) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
System.Threading.Thread.Sleep(1000);
Console.Clear();
string cleared = "Cleared the screen. \nHope you enjoyed this method. \nGood Bye!";
foreach (char c in cleared) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
Console.ReadKey();
}
}
}
출력:
Hi, Welcome!
I am ClearConsole. What's your name?
human
Nice to see you human
You are going to experience Clear() method.
첫째, 이 텍스트는 콘솔의 출력으로 표시됩니다. 1초 후 콘솔이 지워지고 후속 결과는 다음과 같습니다.
Cleared the screen.
Hope you enjoyed this method.
Good Bye!