How to Convert PDF File to Images in C#
- PDF to Image Converter
- Convert PDF File to Images in C# Using the PDFium Package
- Convert PDF File to Images in C# Using the Syncfusion Package
- Convert PDF File to Images in C# Using PDFRasterizer
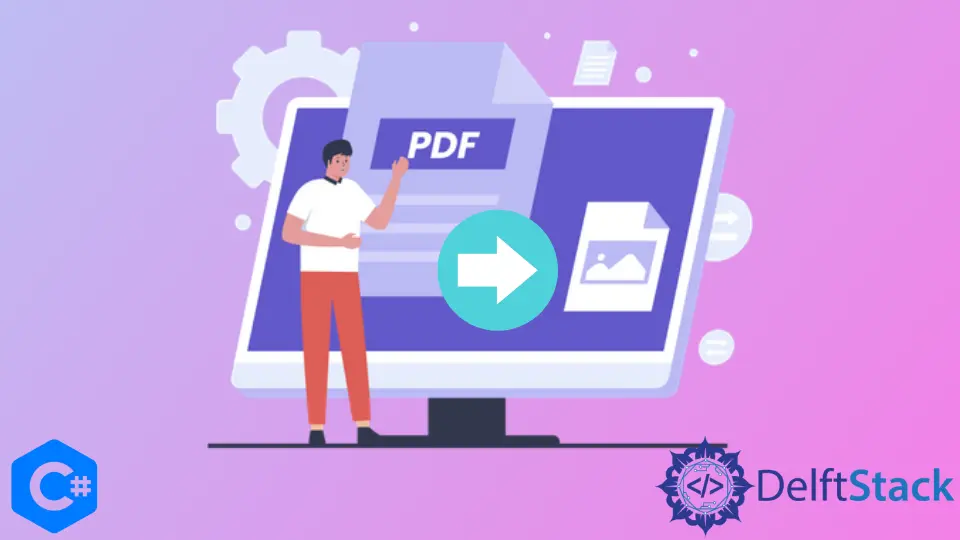
This article demonstrates the conversion of PDF files to images in C#.
We will explore two methods to convert PDF files into images. To elaborate thoroughly, we will create C# projects step by step and provide all the code snippets.
PDF to Image Converter
There are multiple ways to convert PDF files to images using C#. You can use third-party assemblies to create a converter or create your own.
We will make a converter to convert PDF files to images using two different assemblies. These are:
- PDFium
- Syncfusion
These two NuGet packages can be installed from nuget.org into your projects. Let’s create a C# project and guide to install these packages step by step.
Convert PDF File to Images in C# Using the PDFium Package
-
Create a
Console Application
in Visual Studio. We recommend Visual Studio 2019 or later to install and work with these packages. -
Click on the
Project
tab and selectManage NuGet Packages
, or click on theTools
tab, selectNuGet Package Manager
, and selectManage NuGet Packages for Solution
. -
Select the
Browse
tab and set thePackage Source
asnuget.org
. -
Suppose you do not find
nuget.org
in thePackage Source
window; add it by clicking on theSettings
tab. Click the+
icon and type the name and source shown in the image. -
Type
PDFium
in the search bar. Install PdfiumViewer, PdfiumViewer.Native.x86.v8-xfa, and PDFium.x86. -
After you install these packages, a reference to PdfiumViewer will be available in the
References
window. Create a folder in your project directory namedSamples
and place the PDF file you want to convert in this folder. -
Add a reference to
System.Drawing
to import this assembly into your project. Right-click on theReferences
tab in theSolution Explorer
window, selectAdd Reference
, navigate toFramework
, and selectSystem.Drawing
. -
Add the following code snippet to the
Program.cs
file. You can customize the input and output file name as per your requirement. -
This code snippet will convert single-page and multi-page documents. After executing the code, your PDF file should be converted to images (each PDF file page as an image).
using PdfiumViewer; using System; using System.Drawing.Imaging; using System.IO; using System.Linq; namespace PdfToImage { class Program { static void Main(string[] args) { var projectDirectory = Directory.GetParent(Directory.GetCurrentDirectory()).Parent.FullName; try { using (var document = PdfDocument.Load(projectDirectory + @"\Samples\document.pdf")) { var pageCount = document.PageCount; for (int i = 0; i < pageCount; i++) { var dpi = 300; using (var image = document.Render(i, dpi, dpi, PdfRenderFlags.CorrectFromDpi)) { var encoder = ImageCodecInfo.GetImageEncoders().First(c => c.FormatID == ImageFormat.Jpeg.Guid); var encoderParams = new EncoderParameters(1); encoderParams.Param[0] = new EncoderParameter(System.Drawing.Imaging.Encoder.Quality, 100L); image.Save(projectDirectory + @"\Samples\image_" + i + ".jpg", encoder, encoderParams); } } } } catch (Exception ex) { Console.WriteLine(ex.Message); } Console.WriteLine("Pdf converted to images. Press any key to exit..."); Console.ReadKey(); } } }
-
If you face the exception
Unable to find an entry point named 'FPDF_AddRef' in DLL 'pdfium.dll.'
, you need to change the target platform in your build setting. -
Navigate to the
Build
tab and select the platform target according to your system, i.e., x86 or x64. If you do not find the platform target underBuild
, select theProject
tab, select project properties, and find the platform target underBuild
.
Convert PDF File to Images in C# Using the Syncfusion Package
-
Create a
Console Application
in Visual Studio 2019 or a higher version by following step 1 as discussed above. -
Follow steps 2 and 3, described in the above section, to browse the Syncfusion package from NuGet.org.
-
Search for the
Syncfusion.pdfViewer.Windows
package and install it. -
Add references to
System.Drawing
andSystem.Windows.Forms
by following step 6 mentioned above. -
Copy the following code snippet into your
Program.cs
file to convert a single page of a PDF file to an image. You can customize it for multipage conversion using thefor
loop. -
Customize the input and output file names as per your requirement. You can select the specific page to convert by passing the appropriate parameter in the
ExportAsImage()
method.using Syncfusion.Pdf.Parsing; using Syncfusion.Windows.Forms.PdfViewer; using System.Drawing; using System.Drawing.Imaging; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PDFToJPEG { class Program { static void Main(string[] args) { // Initialize the PdfViewer Control PdfViewerControl pdfViewer = new PdfViewerControl(); // Load the input PDF file PdfLoadedDocument document = new PdfLoadedDocument("../../Data/document.pdf"); pdfViewer.Load(document); Bitmap image = pdfViewer.ExportAsImage(0); // Save the image. image.Save("output.jpg", ImageFormat.Jpeg); } } }
Navigate to the project directory->bin->Debug to find the output image file.
Convert PDF File to Images in C# Using PDFRasterizer
PDFRasterizer is a tool to convert PDF files to images developed in C#. It provides the GUI using Windows form.
You can download the source code and can use it without any errors. Navigate to PDFRasterizer to download the component.