How to Open a PDF File in C#
-
Use the
Process.Start()
Method to Open a PDF File inC#
-
Use the
DynamicPDF
Viewer to Open a PDF File inC#
-
Use the
PdfDocument
to Open a PDF File inC#
-
Use the
System.IO.Stream
Instance to Open a PDF File inC#
-
Use the
iTextSharp
NuGet Package to Open a PDF File inC#
-
Use the
PdfViewer
From the Toolbox to Open a PDF File inC#
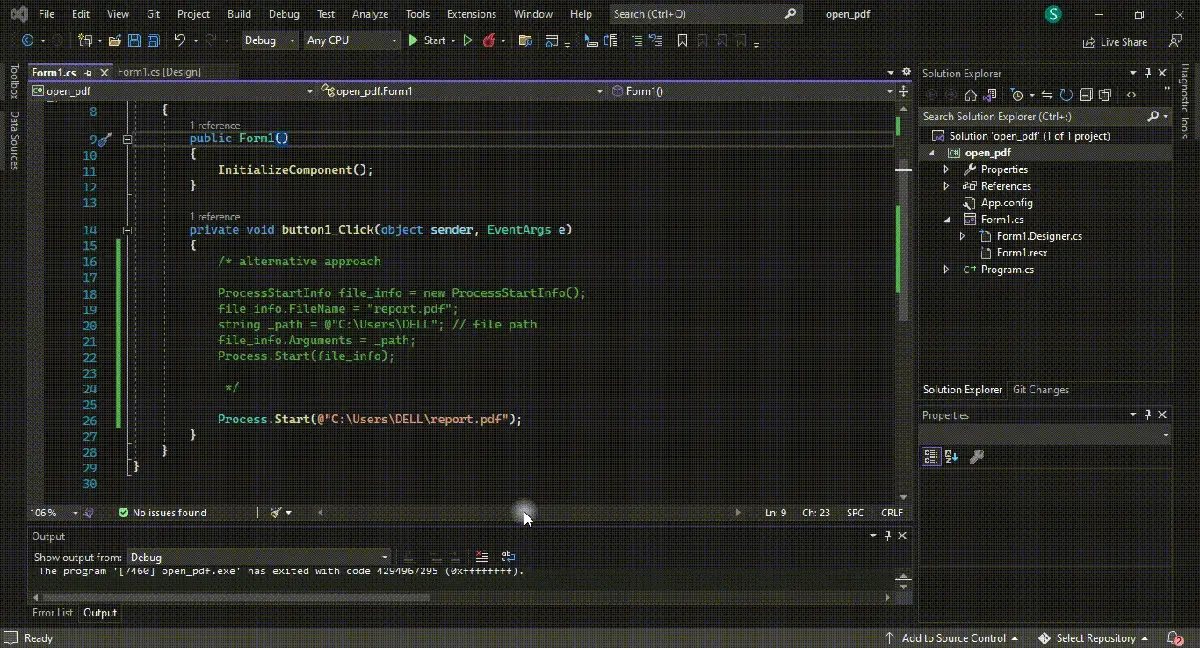
This tutorial will teach you how to open a PDF file inside the Windows Form using C#. There are six possible ways to access a Portable Document Format file with C# programming for your Windows Applications.
Each method is arranged based on the user’s basic knowledge of C# programming and GUI Controls. You can understand and learn these different ways without any programming background with the executable C# examples.
You have to know that there’s no direct support to generate a PDF file in C#; instead, you can use third-party libraries or tools to generate a file. The PDFSharp
is an example that enables your Windows Applications to use drawing routines known from GDI+
published under the MIT license.
Use the Process.Start()
Method to Open a PDF File in C#
The System.Diagnostics.Process.Start()
method in C# works as a system default PDF file or other document viewer. A common problem many beginners have with this approach is the file path.
If you need a relative path from the program’s .exe
file to a folder with resources, add Resources\ or ...\Resources\
to your file path. Otherwise, you can add your PDF files to a C# project as an embedded resource by using Path.GetTempPath()
by saving it to some temporary location and opening it.
using System;
using System.Diagnostics; // essential namespace
using System.Windows.Forms;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
/* alternative approach
ProcessStartInfo file_info = new ProcessStartInfo();
file_info.FileName = "report.pdf";
string _path = @"C:\Users\DELL"; // file path
file_info.Arguments = _path;
Process.Start(file_info);
*/
Process.Start(@"C:\Users\DELL\report.pdf");
}
}
}
Output:
It belongs to the System.Diagnostics
namespace, which also holds the definition of ProcessStartInfo
. It has different parameters, including: fileName
, arguments
, userName
, password
, and domain
to access files, including PDFs.
Use the DynamicPDF
Viewer to Open a PDF File in C#
The DynamicPDF
viewer is a powerful tool for .NET within Windows Applications to access a PDF using a file, byte array, or password-protected file or open a PDF using a Stream
. The examples from this method will explain how you can utilize the DynamicPDF
viewer to access or open .pdf
files.
Install this viewer using the MSI installer provided by the DynamicPDF
and add the DynamicPDFViewer
control to your Visual Studio toolbox after a successful installation. Now, it’s a valid option to access the toolbox to add a DynamicPDFViewer
control to your Windows Forms.
It’s a premium tool with customizable features to enhance the performance of your C# applications. You can fully embed this viewer into any .NET
WinForm application so that you no longer rely on an external viewer for displaying or interacting with your documents and can take advantage of its high efficiency, reliability, and compatibility.
using System;
using System.Windows.Forms;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
pdfViewer1.Open(@"C:\Users\DELL\report.pdf");
}
private void pdfViewer1_Click(object sender, EventArgs e) {}
}
}
Output:
You can install it as a NuGet package in Visual Studio by the name ceTe.DynamicPDF.Viewer.NET
. The x64 and x86 versions of this tool are available, install and put its reference to your C# project to open PDF files more efficiently.
Use the PdfDocument
to Open a PDF File in C#
The Open
method is extremely simple and famous when it comes to opening a PDF file in C# with something like pdfViewer.Open(pdf_path);
and in case you want to open a PDF directly from a byte array which requires you to create an instance of pdfDocument
to pass the byte array to a constructer, you can use the Open
method.
Pass the pdfDocument
instance to the Viewer
control you created using the Open
method like pdfDocument pdf_doc = new pdfDocument(pdf_file);
and can execute it using the Open
method pdfViewer.Open(pdf_doc);
.
Most importantly, for password-protected PDF files, you need to pass the password along with the file path to the constructor like pdfDocument pdf_doc = new pdfDocument(pdf_path, "my_password123");
and use the Open
method to pass this instance to your DynamicPDF
viewer control.
using System;
using System.Windows.Forms;
using ceTe.DynamicPDF.Viewer;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
PdfDocument file_pdf =
new PdfDocument(data_pdf); // `data_pdf` contains the byte array info of pdf file
pdfViewer1.Open(file_pdf);
}
private void pdfViewer1_Click(object sender, EventArgs e) {}
}
}
When you click the Open
button, the PDF file opens from the byte array in the DynamicPDF
Viewer embedded on the Windows Form.
Opening a password-protected PDF file is as simple as opening a PDF file from the byte array by creating an instance of the PdfDocument
and passing the file path and password to the constructor.
As the PDF is encrypted and password protected, you will pass the PdfDocument
instance to your pdfViewer1
using the Open()
method.
using System;
using System.Windows.Forms;
using ceTe.DynamicPDF.Viewer;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
PdfDocument file_pdf = new PdfDocument(
@"C:\Users\DELL\report.pdf", "password"); // first string is the path, and the second
// string is the password on that pdf file
pdfViewer1.Open(file_pdf);
}
private void pdfViewer1_Click(object sender, EventArgs e) {}
}
}
Output:
Use the System.IO.Stream
Instance to Open a PDF File in C#
It is possible to use the System.IO.Stream
instance directly to accomplish this task with the Open
method. It requires creating a pdfDocument
instance from the Stream
, something like pdfDocument pdf_doc = new pdfDocument(pdf_stream);
.
using System;
using System.Windows.Forms;
using ceTe.DynamicPDF.Viewer;
using System.IO;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
/* perform some stream operation to get `pdf_stream`
string dictionary_start = @"C:\Users\DELL\";
string dictionary_end = @"C:\Users\DELL\edvdd";
foreach (string info_file in Directory.EnumerateFiles(dictionary_start))
{
using (FileStream SourceStream = File.Open(info_file, FileMode.Open))
{
using (FileStream DestinationStream = File.Create(dictionary_end +
info_file.Substring(info_file.LastIndexOf('\\'))))
{
await SourceStream.CopyToAsync(DestinationStream);
}
}
}
*/
PdfDocument file_pdf =
new PdfDocument(pdf_stream); // `pdf_stream` contains the pdf path directly from the
// `System.IO.Strem` instance
pdfViewer1.Open(file_pdf);
}
private void pdfViewer1_Click(object sender, EventArgs e) {}
}
}
When you click the Open
button, the PDF file opens from the stream in the pdfViewer1
DynamicPDF
Viewer embedded on the Windows Form.
Use the iTextSharp
NuGet Package to Open a PDF File in C#
Create a new C# project (Windows Form C# Application) in Visual Studio and install the iTextSharp
NuGet package version v5.5.11
or updated version.
It is an important library because it offers functions like CREATE
, INSERT
, ADAPT
, and MAINTAIN
documents in PDF, enabling you to add all possible .pdf
functionalities to your C# project.
Design your form with a button and use the iTextSharp.text.pdf.parser
namespace to handle your form. As an advanced tool library, it will help you create complex PDF reports and enhance your application with PDF functionality.
using System;
using System.Windows.Forms;
using iTextSharp.text.pdf.parser; // essential namespace
using System.Text;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
using (OpenFileDialog dialog_pdffile =
new OpenFileDialog() { Filter = "PDF files|*.pdf", ValidateNames = true,
Multiselect = false }) {
if (dialog_pdffile.ShowDialog() == DialogResult.OK) {
try {
iTextSharp.text.pdf.PdfReader file_proc =
new iTextSharp.text.pdf.PdfReader(dialog_pdffile.FileName);
StringBuilder build_string = new StringBuilder();
for (int i = 1; i <= file_proc.NumberOfPages; i++) {
// read the pdf document
build_string.Append(PdfTextExtractor.GetTextFromPage(file_proc, i));
}
richTextBox1.Text = build_string.ToString();
file_proc.Close();
} catch (Exception ex) {
MessageBox.Show(ex.Message, "Caution, Error occurs!", MessageBoxButtons.OK,
MessageBoxIcon.Error);
}
}
}
}
private void richTextBox1_TextChanged(object sender, EventArgs e) {}
}
}
Output:
Use the PdfViewer
From the Toolbox to Open a PDF File in C#
In this method, you will learn how to open a PDF file using the WinForms PDF Viewer in C# and what assemblies are required to create a simple PDF viewer C# application.
The Patagames.pdf
is a primary assembly containing a class library to create, edit, and load PDF files, also the PDF rendering engine.
The Patagames.Pdf.WinForms
assembly contains the PdfViewer UserControl
, and other UI controls for PDF viewer, and these are the only two assemblies required for this WinForms .pdf
viewer.
Creating the control manually from the code is a complex process with many benefits. It enables you to create a PDF viewer by simply dragging the control from VS toolbox and dropping it in the designer window of your C# form. Navigate the Patagames Pdf.Net
SDK tab and drag the PdfViewer
toolbox item to the Designer window.
Remember, pdfViewer
does not contain any user interface elements and all the crucial functionalities are available through public methods.
After adding the instance to the designer, you can load the document in the pdfViewerControl
with something like pdfViewer1.LoadDocument("file_name.pdf");
.
using System;
using System.Windows.Forms;
namespace open_pdf {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
pdfViewer1.LoadDocument(@"C:\Users\DELL\Resumed.pdf");
}
private void pdfViewer1_Load(object sender, EventArgs e) {}
}
}
Output:
Another approach you can use with the help of this SDK tab is opening a PDF document using the properties by navigating the Patagames Pdf.Net SDK
tab and dragging the PdfToolStripMain
toolbox item to the designer window to add its instance to the designer .cs
file.
Afterward, click the PdfViewer
property in the property grid and select the pdfViewer1
from the drop-down list. Now you can load or add PDF documents by clicking the Open
icon.
As we are discussing button click events, you can manually add the controls from the C# code by adding namespace references of assemblies, creating a pdfViewer
instance, and loading the PDF file. In the button’s click event, initialize the PdfViewerControl
, load the document, and add it to the form.
The Spire
PDF viewer is a valid alternative to the Patagames
PDF viewer for simply opening the PDF files in C#. Download the Spire.PDFViewer
, add a tool script in your Windows form, and add Spire.PdfViewer.Forms
reference to your C# project to either directly load the PDF files from the system or choose a PDF file from a dialog box from your computer.
In this tutorial, you have learned how to open a PDF or any other file in C# using the most effective methods to maintain performance.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub