HTML to PDF in C#
- Getting Started with HtmlRenderer.PdfSharp
- Basic HTML to PDF Conversion
- Handling Complex HTML Structures
- Conclusion
- FAQ
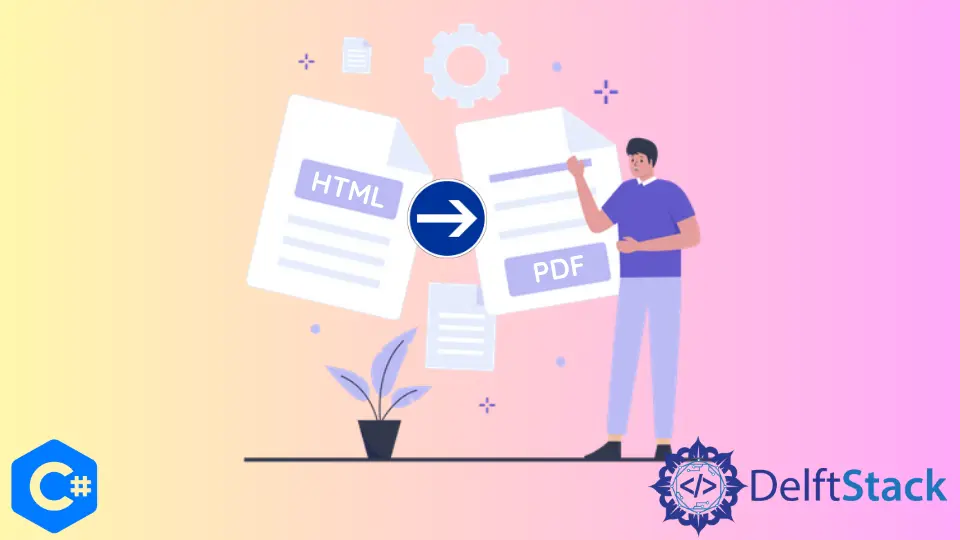
In today’s digital landscape, converting HTML to PDF is a common requirement for many developers. Whether you’re generating reports, invoices, or simply saving web content for offline use, having a reliable method to convert HTML to PDF in C# can streamline your workflow. One of the most effective ways to achieve this is through the HtmlRenderer.PdfSharp package. This package allows developers to convert HTML strings into PDF documents effortlessly.
In this article, we will explore how to use the HtmlRenderer.PdfSharp package to perform this conversion, providing you with clear code examples and explanations to help you implement this functionality in your own projects.
Getting Started with HtmlRenderer.PdfSharp
Before diving into the code, it’s essential to set up your environment. To begin, you need to install the HtmlRenderer.PdfSharp
package. You can do this using the NuGet Package Manager in Visual Studio or via the command line. Here’s how to install it using the NuGet Package Manager Console:
Install-Package HtmlRenderer.PdfSharp
or,
dotnet add package HtmlRenderer.PdfSharp-- version 1.5.0.6
After installation, you can start using the package in your C# projects. The HtmlRenderer.PdfSharp library is built on top of PdfSharp, which is a popular library for creating PDF documents in .NET. This combination allows you to render HTML content directly into a PDF format.
Basic HTML to PDF Conversion
Now that you have the necessary package installed, let’s look at a basic example of converting an HTML string into a PDF document. Below is a simple C# code snippet that demonstrates this process.
using System;
using System.IO;
using PdfSharp.Pdf;
using TheArtOfDev.HtmlRenderer.PdfSharp;
class Program
{
static void Main()
{
string html = "<h1>Document</h1> <p>This is an HTML document which is converted to a pdf file.</p>";
string pdfPath = "output.pdf";
using (PdfDocument pdf = new PdfDocument())
{
PdfGenerator.AddPdfPages(pdf, html, PageSize.A4);
pdf.Save(pdfPath);
}
Console.WriteLine("PDF generated successfully.");
}
}
The code begins by importing necessary namespaces. It initializes a string containing HTML content and specifies the output file path for the PDF. The PdfGenerator.AddPdfPages
method is called to convert the HTML into PDF pages, and finally, the document is saved to the specified path. This simple example effectively demonstrates how to create a PDF from an HTML string.
Output:
PDF generated successfully.
This straightforward approach allows you to convert basic HTML content into a PDF file. However, for more complex HTML structures, you may need to explore additional options and configurations that HtmlRenderer.PdfSharp offers.
Handling Complex HTML Structures
When dealing with more complex HTML, such as those containing CSS styles and images, you need to ensure that your HTML content is well-structured. The HtmlRenderer.PdfSharp package can handle CSS styles, but there are some limitations. Here’s how you can convert a more complex HTML string into a PDF.
using System;
using System.IO;
using PdfSharp.Pdf;
using TheArtOfDev.HtmlRenderer.PdfSharp;
class Program
{
static void Main()
{
string html = @"
<html>
<head>
<style>
h1 { color: blue; }
p { font-size: 14px; }
</style>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a sample PDF document with styles.</p>
<img src='https://example.com/image.png' alt='Sample Image' />
</body>
</html>";
string pdfPath = "complex_output.pdf";
using (PdfDocument pdf = new PdfDocument())
{
PdfGenerator.AddPdfPages(pdf, html, PageSize.A4);
pdf.Save(pdfPath);
}
Console.WriteLine("Complex PDF generated successfully.");
}
}
In this example, we define a more intricate HTML structure that includes styles and an image. The PdfGenerator.AddPdfPages
method still handles the conversion seamlessly. However, note that external images may not render correctly unless they are accessible at the time of conversion. Always ensure your HTML is valid and well-formed to avoid issues during PDF generation.
Output:
Complex PDF generated successfully.
This method gives you the flexibility to create visually appealing PDFs that retain the formatting and styles of your original HTML content. With HtmlRenderer.PdfSharp, you can effectively manage the conversion of HTML to PDF, even for more sophisticated designs.
Conclusion
Converting HTML to PDF in C# using the HtmlRenderer.PdfSharp package is a straightforward and efficient process. With just a few lines of code, you can generate PDFs from simple or complex HTML content, making it an invaluable tool for developers. Whether you are creating reports, invoices, or any other documentation, this method allows you to maintain the integrity of your HTML while providing users with a downloadable PDF format. Start integrating this functionality into your projects today and enhance your application’s capabilities.
FAQ
-
What is the HtmlRenderer.PdfSharp package?
HtmlRenderer.PdfSharp is a .NET library that allows developers to convert HTML strings into PDF documents using the PdfSharp library. -
Can I use CSS with HtmlRenderer.PdfSharp?
Yes, HtmlRenderer.PdfSharp supports basic CSS styles, allowing you to style your HTML content before converting it to PDF. -
How do I handle images in my HTML for PDF conversion?
Ensure that images are accessible via a URL or embedded in the HTML. External images must be reachable at the time of conversion. -
Is it possible to customize the PDF output?
Yes, you can customize the PDF output by modifying the HTML content, including styles and layout as needed. -
What are the limitations of HtmlRenderer.PdfSharp?
While it supports basic HTML and CSS, complex layouts and advanced CSS features may not render as expected. Always test your output.
using the HtmlRenderer.PdfSharp package. This article provides step-by-step instructions and code examples for generating PDFs from HTML strings, including handling complex structures and styles. Enhance your development skills with this essential guide.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn