How to Crop an Image in C#
-
Use the
Graphics.DrawImage()
Method to Draw and Crop an Image inC#
-
Use
DrawImage()
With Five Parameters to Crop an Image inC#
-
Use
DrawImage()
With Specified Portion, Location, and Size to Crop an Image inC#
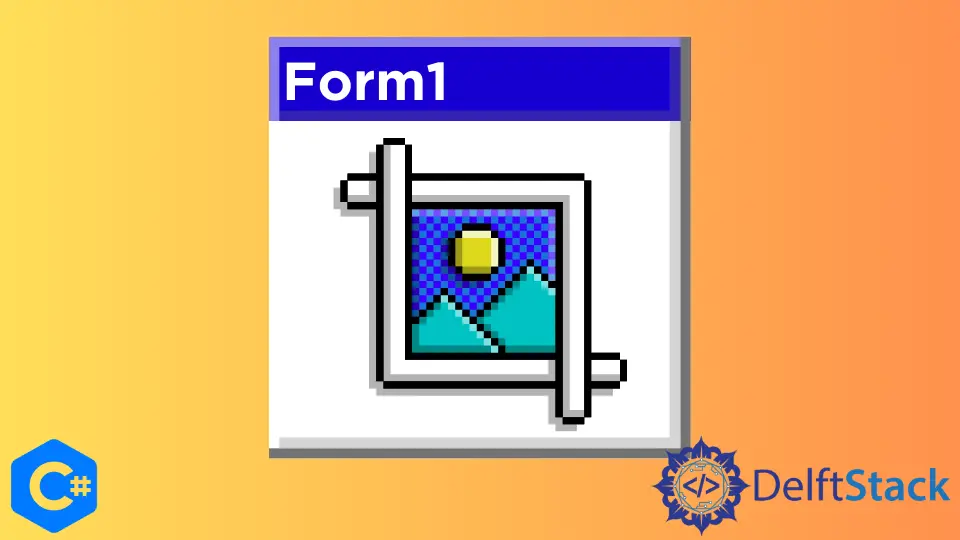
This article will introduce how to draw and crop a specific image at a specified location with the original size using a C# code.
Use the Graphics.DrawImage()
Method to Draw and Crop an Image in C#
Graphics can be found in the System.Drawing
namespace and are primarily used to display or draw graphics or images in .Net-based
Windows Applications. .Net
provides multiple classes to deal with graphics objects and shapes such as pens, brushes, rectangles, circles, etc.
The Graphics and Image .Net
classes can render an image on a Windows Form. The DrawImage()
function draws a portion of an image at a specified location.
Below is a code snippet for the DrawImage
function.
public void DrawImage(System.Drawing.Image image, float x, float y,
System.Drawing.RectangleF srcRect, System.Drawing.GraphicsUnit srcUnit);
The parameters are explained below:
image
- specifies the image to draw.x
- specifies the x-coordinate of the image.y
- specifies the y-coordinate of the image.srcRect
- a RectangleF structure that specifies the portion of the image to draw.srcUnit
- a member of the GraphicsUnit enumeration that specifies the units of measure used bysrcRect
.
The following code example is implemented using Windows Forms and the Paint
event handler with PaintEventArgs e
as a parameter.
Below are the enumerated actions performed:
- An image was created from a JPEG file
Koala.jpg
located in the folderC:\Users\lolaa\Downloads
. - The coordinates to draw the image were created.
- A source rectangle was created to extract a portion of the image.
- The unit of measure was set to pixels.
- The image was drawn to the screen.
The specified source rectangle size determined the portion of the original unscaled image drawn on the screen.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace CreatingImageObject {
public partial class Form1 : Form {
Image img = null;
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
if (img == null) {
img = Image.FromFile(@"C:\Users\lolaa\Downloads\Koala.jpg");
}
}
private void Form1_Paint(object sender, PaintEventArgs e) {
if (img != null) {
// Graphics g = e.Graphics;
Graphics g = this.CreateGraphics();
g.DrawImage(img, 0, 0, this.Width, this.Height);
g.Dispose();
}
}
}
}
Output:
When you click on the button, the image below is displayed:
Output:
Rectangle cropRect = new Rectangle(...);
Bitmap src = Image.FromFile(fileName) as Bitmap;
Bitmap target = new Bitmap(cropRect.Width, cropRect.Height);
using (Graphics g = Graphics.FromImage(target)) {
g.DrawImage(src, new Rectangle(0, 0, target.Width, target.Height), cropRect, GraphicsUnit.Pixel);
}
Use DrawImage()
With Five Parameters to Crop an Image in C#
To edit or crop the image we drew earlier, we can use the DrawImage(Image, Single, Single, RectangleF, GraphicsUnit)
function. You can see here that we used five parameters inside the function.
Updating the above code would give a different output. Below is the updated code example.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace CreatingImageObject {
public partial class Form1 : Form {
Image img = null;
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
if (img == null) {
// Create image.
img = Image.FromFile(@"C:\Users\lolaa\Downloads\Koala.jpg");
}
}
private void Form1_Paint(object sender, PaintEventArgs e) {
if (img != null) {
Graphics g = this.CreateGraphics();
// specify the coordinates for upper-left corner of image.
float x = 100.0F;
float y = 100.0F;
// rectangle is created for the source image with custom dimensions.
RectangleF srcRect = new RectangleF(50.0F, 50.0F, 150.0F, 150.0F);
GraphicsUnit dim = GraphicsUnit.Pixel;
// image is drawn to the screen.
g.DrawImage(img, x, y, srcRect, dim);
g.Dispose();
}
}
}
}
Output:
Use DrawImage()
With Specified Portion, Location, and Size to Crop an Image in C#
We can also use the DrawImage()
function to draw a specified portion of the selected image at the specified location and with the specified size.
Syntax:
public void DrawImage(System.Drawing.Image image, System.Drawing.Rectangle destRect, float srcX,
float srcY, float srcWidth, float srcHeight,
System.Drawing.GraphicsUnit srcUnit,
System.Drawing.Imaging.ImageAttributes? imageAttrs,
System.Drawing.Graphics.DrawImageAbort? callback, IntPtr callbackData);
Updating the above code would give a different output. Below is the updated code example.
The parameters are explained below:
image
- specifies the image to draw.destRect
- specifies the location and size of the image on the screen. It scales the drawn image to fit the rectangle.srcX
andsrcY
- the x- and y-coordinates of the upper-left corner of the image.srcWidth
andsrcHeight
- specify the width and height of the image, respectively.srcUnit
- belongs to theGraphicsUnit
enumeration. It is the units of measurement used by thesrcRect
parameter.imageAttrs
- anImageAttributes
instance that specifies recoloring and gamma information for image objects.callback
- a delegate function of theGraphics.DrawImageAbort
. It specifies theDrawImage()
overload to call when drawing the image. The application’s specification determines the number of times this method will be called.callbackData
- a value specifies additional data for theGraphics.DrawImageAbort
delegate to use when checking whether to stop the execution of theDrawImage
method.
The example of code below was also implemented using Windows Forms and the Paint
event handler with PaintEventArgs e
as a parameter.
The code performs the following actions:
- An instance of the
Graphics.DrawImageAbort
call-back method was created. - A
Koala.jpg
image located in folderC:\Users\lolaa\Downloads
was created. - Points were created for the destination rectangle to draw the image.
- A rectangle container was created to specify the portion of the image to draw.
- The graphics drawing unit was set to pixel.
- The original image was drawn to the screen.
- An additional rectangle container to draw an adjusted image.
- Sets the attributes of the adjusted image to a larger gamma value.
- The adjusted image was drawn to the screen.
The Graphics.DrawImageAbort
call-back returns a bool which determines the execution of the DrawImage
method.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Imaging;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace CreatingImageObject {
public partial class Form1 : Form {
Image img = null;
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
// Define DrawImageAbort callback method.
private bool DrawImageCallback8(IntPtr callBackData) {
// Test for call that passes callBackData parameter.
if (callBackData == IntPtr.Zero) {
// If no callBackData passed, abort the DrawImage method.
return true;
} else {
// If callBackData passed, continue DrawImage method.
return false;
}
}
private void button1_Click(object sender, EventArgs e) {
if (img == null) {
// Create image.
img = Image.FromFile(@"C:\Users\lolaa\Downloads\Koala.jpg");
}
}
private void Form1_Paint(object sender, PaintEventArgs e) {
if (img != null) {
Graphics g = this.CreateGraphics();
// Create a call-back method.
Graphics.DrawImageAbort imageCallback = new Graphics.DrawImageAbort(DrawImageCallback8);
IntPtr imageCallbackData = new IntPtr(1);
// Create image.
// Image newImage = Image.FromFile(@"C:\Users\lolaa\Downloads\Koala.jpg");
// img = Image.FromFile(@"C:\Users\lolaa\Downloads\Koala.jpg");
// rectangle for the original image.
Rectangle destRectOriginal = new Rectangle(100, 25, 450, 150);
// coordinates of the rectangle.
float x = 50.0F;
float y = 50.0F;
float width = 150.0F;
float height = 150.0F;
GraphicsUnit dim = GraphicsUnit.Pixel;
// Draw original image to screen.
g.DrawImage(img, destRectOriginal, x, y, width, height, dim);
// rectangle for the adjusted image.
Rectangle destRectAdjusted = new Rectangle(100, 175, 450, 150);
// image attributes and sets gamma.
ImageAttributes imageAttribute = new ImageAttributes();
imageAttribute.SetGamma(4.0F);
// Draw adjusted image to screen.
try {
checked {
// Draw adjusted image to screen.
g.DrawImage(img, destRectAdjusted, x, y, width, height, dim, imageAttribute,
imageCallback, imageCallbackData);
}
} catch (Exception ex) {
g.DrawString(ex.ToString(), new Font("Arial", 8), Brushes.Black, new PointF(0, 0));
}
}
}
public void DrawImageRect4FloatAttribAbortData(PaintEventArgs e) {}
}
}
Output:
Further discussion on other overloaded DrawImage()
methods is available in this reference.