How to Download Image in C#
-
Download Image With the
WebClient
Class inC#
-
Download Image Without Knowing the Format With the
Bitmap
Class inC#
-
Download Image Without Knowing Format With the
Image.FromStream()
Function inC#
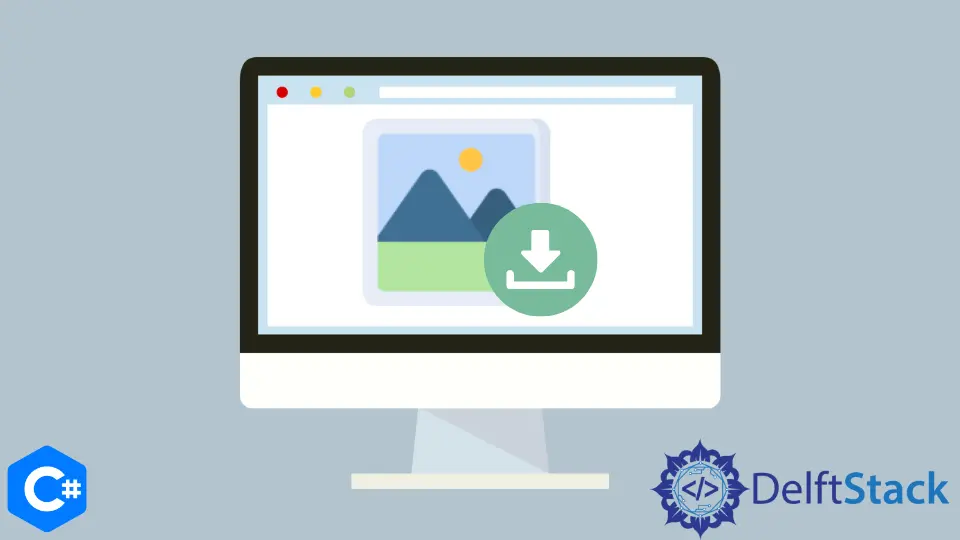
This tutorial will discuss the methods for downloading an image in C#.
Download Image With the WebClient
Class in C#
The WebClient
class provides functionality for sending data to and receiving data from a URL in C#. The WebClient.DownloadFile(url, path)
function downloads a file from a particular URL url
and save it to the path
. We can use the WebClient.DownloadFile()
function to download an image from a URL.
using System;
using System.Net;
using System.Runtime.InteropServices;
namespace download_image {
class Program {
static void download() {
string url =
"https://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Breathe-face-smile.svg/1200px-Breathe-face-smile.svg.png";
using (WebClient client = new WebClient()) {
client.DownloadFile(new Uri(url), "Image.png");
}
}
static void Main(string[] args) {
try {
download();
} catch (ExternalException e) {
Console.WriteLine(e.Message);
} catch (ArgumentNullException e) {
Console.WriteLine(e.Message);
}
}
}
}
In the above code, we downloaded an image from the URL url
and saved it to the path Image.png
with the client.DownloadFile(new Uri(url), "Image.png")
function in C#.
Download Image Without Knowing the Format With the Bitmap
Class in C#
In the above example, we have to know the image file format to be downloaded; then, we can download it from the URL and save it. But, if we do not know the image file format, we can use the Bitmap
class. The Bitmap
class provides methods for working with images in C#. This method will download and save files in all the formats that the Bitmap
class can handle. The Bitmap.Save(path, format)
function writes the contents of our bitmap to a file in the path
with the format format
. The ImageFormat
class can be used inside the Bitmap.Save()
function to manually specify the format of the image to be saved. The following code example shows us how we can download an image from a URL without knowing the format with the Bitmap.Save()
function in C#.
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Net;
using System.Runtime.InteropServices;
namespace download_image {
class Program {
static void SaveImage() {
string url =
"https://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Breathe-face-smile.svg/1200px-Breathe-face-smile.svg.png";
WebClient client = new WebClient();
Stream stream = client.OpenRead(url);
Bitmap bitmap = new Bitmap(stream);
if (bitmap != null) {
bitmap.Save("Image1.png", ImageFormat.Png);
}
stream.Flush();
stream.Close();
client.Dispose();
}
static void Main(string[] args) {
try {
SaveImage();
} catch (ExternalException e) {
Console.WriteLine(e.Message);
} catch (ArgumentNullException e) {
Console.WriteLine(e.Message);
}
}
}
}
We defined the SaveImage()
function that downloads and saves an image. We used the WebClient
class to make web requests to the url
. We used the Stream
class to read the data from the client.OpenRead(url)
function. We used the Bitmap
class to convert the stream to a bitmap format. We then used the bitmap.Save()
function to save the bitmap
in the path Image1.png
with the format being ImageFormat.Png
.
Download Image Without Knowing Format With the Image.FromStream()
Function in C#
We can also do the same thing as the previous example with the Image.FromStream()
function in C#. The Image.FromStream()
function reads an image file from a memory stream in C#. For this, we can first download all the data from our URL to an array of bytes. Then, we can load that array into an object of the MemoryStream
class. We can then use the Image.FromStream()
function to read an image from the object of the MemoryStream
class. We can then save this image to a path in a particular format with the Image.Save(path, format)
function in C#. The following code example shows us how to download an image from a URL without knowing its formatting with the Image.FromStream()
function in C#.
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Net;
using System.Runtime.InteropServices;
namespace download_image {
class Program {
static void SaveImage() {
string url =
"https://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Breathe-face-smile.svg/1200px-Breathe-face-smile.svg.png";
using (WebClient webClient = new WebClient()) {
byte[] data = webClient.DownloadData(url);
using (MemoryStream mem = new MemoryStream(data)) {
using (var yourImage = Image.FromStream(mem)) {
yourImage.Save("Image2.png", ImageFormat.Png);
}
}
}
}
static void Main(string[] args) {
try {
SaveImage();
} catch (ExternalException e) {
Console.WriteLine(e.Message);
} catch (ArgumentNullException e) {
Console.WriteLine(e.Message);
}
}
}
}
We defined the SaveImage()
function that downloads and saves an image. We used the array of bytes data
to store the data returned by the webClient.DownloadData(url)
function. We then initialized an instance mem
of the MemoryStream
class with data
. We then read the image yourImage
from the mem
with the Image.FromStream(mem)
function. In the end, we saved the image yourImage
to the Image2.png
path with the ImageFormat.Png
formatting by using the yourImage.Save("Image2.png", ImageFormat.Png)
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn