How to Convert an Image to Base64 String in C#
- Understanding Base64 Encoding
- Method 1: Using MemoryStream
- Method 2: Using FileStream
- Method 3: Using Image Class
- Conclusion
- FAQ
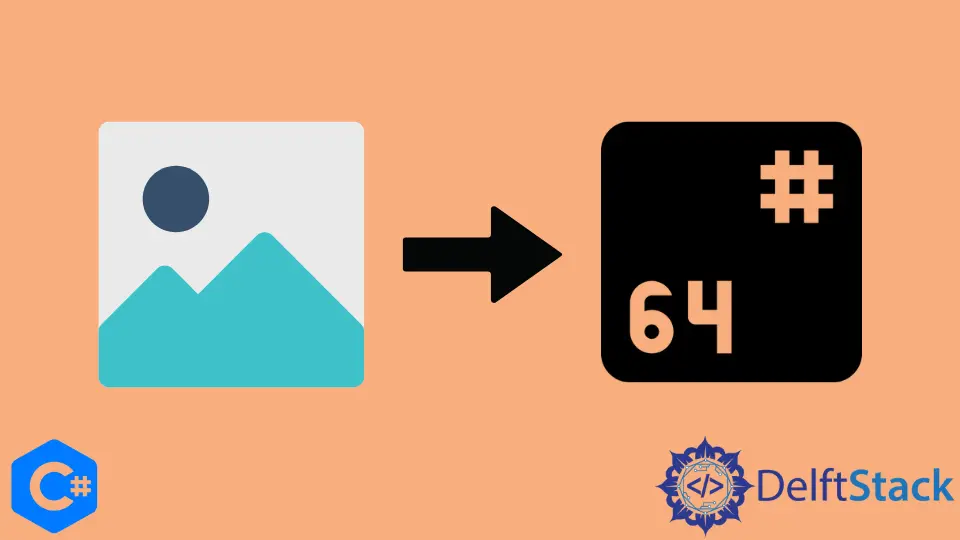
In the world of web development and programming, the need to convert images into a Base64 string is a common requirement. This process allows images to be embedded directly into HTML or CSS, which can be particularly useful for optimizing web applications. If you’re working with C#, converting an image to a Base64 string is straightforward and can be done in just a few steps.
This article will guide you through the process of converting an image into a Base64 string using C#, providing clear examples and explanations along the way. Whether you’re a seasoned developer or a beginner, you’ll find this guide helpful for your coding journey.
Understanding Base64 Encoding
Base64 encoding is a method of converting binary data into a text format, which makes it easier to transmit over media that are designed to handle textual data. Images, being binary files, can be encoded into Base64 strings and embedded in various formats. This is particularly useful for web applications, where you might want to include images directly in your HTML or CSS files without making additional HTTP requests.
When you convert an image to a Base64 string, you essentially represent the binary data of the image as a string of ASCII characters. This can help in reducing the number of requests to the server, thus improving loading times in some scenarios.
Method 1: Using MemoryStream
One of the simplest methods to convert an image to a Base64 string in C# is by using the MemoryStream
class. Below is a step-by-step example of how to achieve this.
using System;
using System.IO;
class Program
{
static void Main()
{
string imagePath = "path_to_your_image.jpg";
string base64String = ConvertImageToBase64(imagePath);
Console.WriteLine(base64String);
}
static string ConvertImageToBase64(string imagePath)
{
byte[] imageBytes = File.ReadAllBytes(imagePath);
return Convert.ToBase64String(imageBytes);
}
}
Output:
<base64_encoded_string>
In this code, we first specify the path to the image you want to convert. The ConvertImageToBase64
method reads the image file into a byte array using File.ReadAllBytes
. Once we have the byte array, we convert it to a Base64 string using the Convert.ToBase64String
method. Finally, we print the Base64 string to the console.
This method is efficient and easy to implement, especially for small to medium-sized images. However, keep in mind that very large images can lead to high memory usage, so it’s advisable to optimize image sizes for web applications.
Method 2: Using FileStream
Another effective way to convert an image to a Base64 string in C# is by utilizing the FileStream
class. This method is particularly useful for large images, as it allows you to read the image in chunks rather than loading the entire file into memory at once.
using System;
using System.IO;
class Program
{
static void Main()
{
string imagePath = "path_to_your_image.jpg";
string base64String = ConvertImageToBase64(imagePath);
Console.WriteLine(base64String);
}
static string ConvertImageToBase64(string imagePath)
{
using (FileStream fs = new FileStream(imagePath, FileMode.Open, FileAccess.Read))
{
byte[] imageBytes = new byte[fs.Length];
fs.Read(imageBytes, 0, (int)fs.Length);
return Convert.ToBase64String(imageBytes);
}
}
}
Output:
<base64_encoded_string>
In this example, we open the image file using FileStream
and read it into a byte array. The using
statement ensures that the file stream is properly disposed of after use, which is crucial for resource management. Once we have the byte array, we convert it to a Base64 string just like in the previous method.
This method is particularly beneficial when working with large images, as it minimizes memory consumption by not loading the entire file into memory at once. It is a good practice to consider this approach when dealing with images that are large in size.
Method 3: Using Image Class
For those working with images in a more graphical context, such as WinForms or WPF applications, you can use the Image
class from the System.Drawing
namespace. This method provides additional flexibility, allowing you to manipulate the image before converting it to a Base64 string.
using System;
using System.Drawing;
using System.IO;
class Program
{
static void Main()
{
string imagePath = "path_to_your_image.jpg";
string base64String = ConvertImageToBase64(imagePath);
Console.WriteLine(base64String);
}
static string ConvertImageToBase64(string imagePath)
{
using (Image image = Image.FromFile(imagePath))
{
using (MemoryStream ms = new MemoryStream())
{
image.Save(ms, System.Drawing.Imaging.ImageFormat.Jpeg);
byte[] imageBytes = ms.ToArray();
return Convert.ToBase64String(imageBytes);
}
}
}
}
Output:
<base64_encoded_string>
In this approach, we load the image using Image.FromFile
, which allows for additional manipulation if needed. We then save the image to a MemoryStream
in JPEG format. Finally, we convert the stream to a byte array and then to a Base64 string.
This method is particularly useful if you need to resize or alter the image before encoding it. It provides a more graphical approach to image processing in C# applications, making it suitable for desktop applications where image manipulation is common.
Conclusion
Converting an image to a Base64 string in C# is a straightforward process that can be accomplished using various methods. Whether you choose to use MemoryStream
, FileStream
, or the Image
class, each method has its own advantages depending on your specific needs. By understanding these techniques, you can efficiently embed images in your web applications or handle image data in your C# projects. Remember to consider the size of the images you are working with, as large images can affect performance and memory usage.
FAQ
-
What is Base64 encoding?
Base64 encoding is a method of converting binary data into a text format, making it easier to transmit over text-based media. -
Why would I convert an image to a Base64 string?
Converting an image to a Base64 string allows you to embed the image directly into HTML or CSS, which can reduce server requests and improve loading times. -
Can I convert other file types to Base64 in C#?
Yes, you can convert various file types to Base64 in C# using similar methods, as long as you read the file into a byte array. -
Are there any downsides to using Base64 encoding for images?
Yes, Base64 encoded images are larger in size than their binary counterparts, which can lead to increased data transfer sizes. -
How can I decode a Base64 string back to an image in C#?
You can decode a Base64 string back to an image by converting the string back to a byte array and then using theImage.FromStream
method.
#. Discover various methods, including using MemoryStream, FileStream, and the Image class for efficient image handling in your C# applications. Whether you’re a beginner or an experienced developer, this guide provides clear examples and explanations to help you understand the process of Base64 encoding images.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn