How to Pass Object by Reference in C#
- Pass Variables by Value to a Function in C#
- Pass Variables by Reference to a Function in C#
- Pass Objects by Reference to a Function in C#
- Conclusion
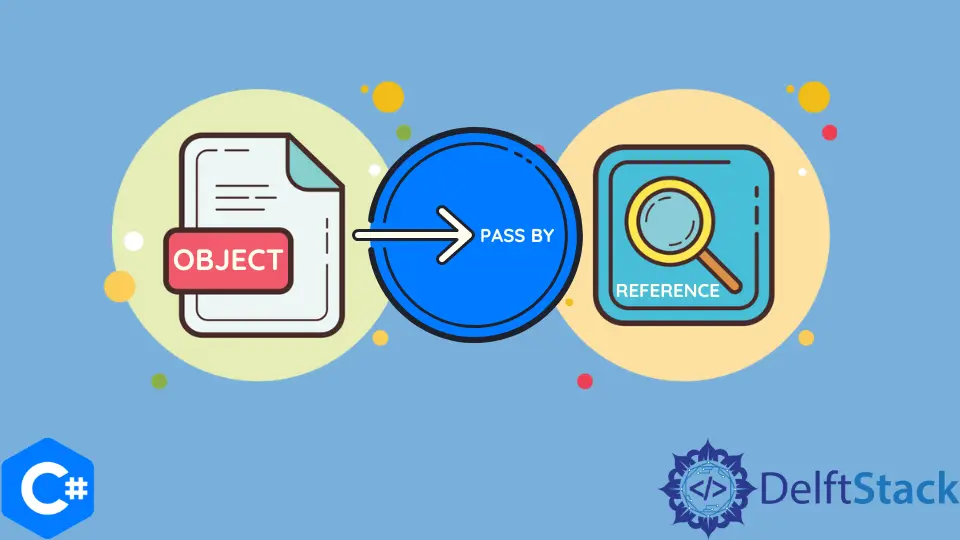
Navigating the intricacies of passing objects to functions in C# is a fundamental aspect of programming. This tutorial dives into the methods employed for this purpose, providing insights into the nuances of passing variables by value and reference.
Understanding how these mechanisms operate is crucial, influencing the behavior of objects within functions and their impact on the original variables. We explore examples showcasing the default pass-by-value behavior, using the ref
keyword to pass by reference, and the default pass-by-reference approach for reference types.
Pass Variables by Value to a Function in C#
By default, C# passes parameters by value, meaning a copy of the variable is sent to the method. However, there are instances where we may want to simulate passing an object by reference using variables by value.
In this context, modifications within a method should reflect on the original object outside the method.
Consider a scenario where we have a method named method1
that attempts to modify a string passed to it. We’ll explore how this affects the original string value in the calling method.
using System;
namespace pass_object_by_reference {
class Program {
static void method1(string value) {
// Modifying the value parameter
value = "something";
Console.WriteLine("New value = {0}", value);
}
static void Main(string[] args) {
// Creating a string variable
string valueType = "Nothing";
// Calling method1 with the string variable
method1(valueType);
// Displaying the original value of the string variable
Console.WriteLine("Original Value = {0}", valueType);
}
}
}
In this code snippet, we have a Program
class containing two methods: method1
and Main
. The goal is to understand how the string value, initially set to Nothing
, is affected when passed to method1
.
In the Main
method, we declare a string variable named valueType
and set its initial value to Nothing
.
The method1
method takes a string parameter named value
. Within this method, we attempt to modify the value of value
to something
.
After calling method1
in the Main
method, we then display the original value of the valueType
string.
Output:
New value = something
Original Value = Nothing
Pass Variables by Reference to a Function in C#
We can also pass a value type variable by reference with the ref
keyword in C#. The ref
keyword specifies that a value is passed by reference to a function. With this approach, there will only exist one copy of the variable in the memory, and a separate pointer will be passed to the function that points to the value of the variable.
We’ll explore how using the ref
keyword affects the original string value in the calling method.
using System;
namespace pass_object_by_reference {
class Program {
static void method1(ref string value) {
// Modifying the value parameter directly
value = "something";
Console.WriteLine("New value = {0}", value);
}
static void Main(string[] args) {
// Creating a string variable
string valueType = "Nothing";
// Calling method1 with the string variable by reference
method1(ref valueType);
// Displaying the original value of the string variable
Console.WriteLine("Original Value = {0}", valueType);
}
}
}
In this code snippet, we have a Program
class containing two methods: method1
and Main
. The goal is to understand how using the ref
keyword affects the original string value when passed to method1
.
In the Main
method, we declare a string variable named valueType
and set its initial value to Nothing
.
The method1
method takes a string parameter named value
but with the ref
keyword. This signifies that we are passing the variable by reference, allowing modifications inside the method to directly affect the original variable.
Within the method1
method, we modify the value of the value
parameter to something
.
After calling method1
in the Main
method, we then display the original value of the valueType
string.
Output:
New value = something
Original Value = something
Pass Objects by Reference to a Function in C#
There are situations where you might want to pass an object by reference, allowing the method to directly manipulate the original object. This is particularly useful when dealing with large objects or when modifications should persist beyond the method’s scope.
In this section, we’ll explore how to pass an object by reference in C# using the natural behavior of reference types.
Consider a scenario where we have a Sample
class with a string property. We want to understand how passing an instance of this class to a method affects the original object.
using System;
namespace pass_object_by_reference {
public class Sample {
public string s { get; set; }
}
class Program {
static void dosomething(Sample obj) {
// Displaying the initial value of the 's' property
Console.WriteLine("Initial passed value = {0}", obj.s);
// Creating a new instance of Sample and assigning a value to the 's' property
obj = new Sample();
obj.s = "something";
// Displaying the new value of the 's' property
Console.WriteLine("New value = {0}", obj.s);
}
static void Main(string[] args) {
// Creating an instance of Sample
Sample sam = new Sample();
sam.s = "Nothing";
// Calling the dosomething method with the Sample object
dosomething(sam);
// Displaying the original value of the 's' property
Console.WriteLine("Original Value = {0}", sam.s);
}
}
}
In this code snippet, we have a Sample
class with a string property named s
. The Program
class contains two methods: dosomething
and Main
.
In the Main
method, we create an instance of the Sample
class named sam
and set its s
property to Nothing
.
The dosomething
method takes a Sample
object (obj
) as a parameter. Within this method, we display the initial value of the s
property of the passed object.
Next, we create a new instance of Sample
and assign a value to its s
property. Finally, we display the new value of the s
property.
After calling the dosomething
method in the Main
method, we display the original value of the s
property of the sam
object.
Output:
Initial passed value = Nothing
New value = something
Original Value = Nothing
Conclusion
Mastering the art of passing objects to functions in C# involves grasping the subtleties of pass-by-value and pass-by-reference methodologies. The showcased examples elucidate how variables and objects behave within functions, emphasizing the critical differences between reference and value types.
Developers are equipped with the knowledge to choose the appropriate approach for their specific scenarios, ensuring effective manipulation of objects and preventing unintended consequences. Whether it’s leveraging the default behavior, employing the ref
keyword for explicit pass-by-reference, or understanding the intricacies of reference types, this tutorial provides a comprehensive guide to navigating object passing in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn