Partial Class in C#
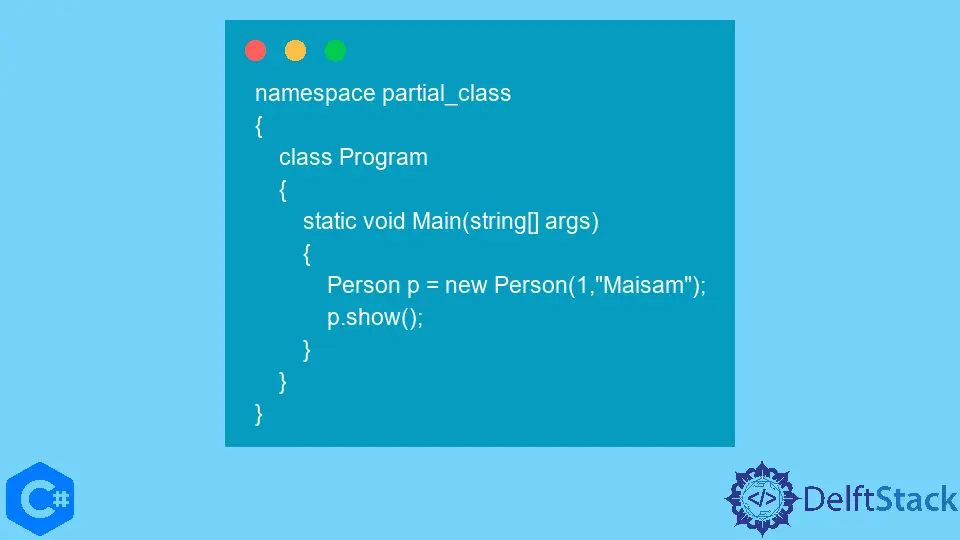
In this tutorial, we will discuss the partial class in C#.
the Partial Class in C#
The partial
keyword specifies a partial class in C#. If we have a class with multiple responsibilities, we should split it into two separator classes, each having only one purpose. One way of achieving this is to create a separate class with separate dependencies in C#. Another easier way to deal with this problem is to create one partial class for each responsibility. We can split the definition of one class across multiple files with the partial classes.
In this tutorial, we will split the definition of the Person
class across multiple files Person1.cs
and Person2.cs
.
Person.cs
:
using System;
namespace partial_class {
public partial class Person {
private int id;
private string name;
public Person(int id, string name) {
this.id = id;
this.name = name;
}
public void show() {
Console.WriteLine("Person id = {0}", id);
Console.WriteLine("Person Name = {0}", name);
}
}
}
We will split the definition of this class across multiple files with the help of the partial
keyword in C#. The content of the Person1.cs
and Person2.cs
classes is given below.
Person1.cs
:
namespace partial_class {
public partial class Person {
private int id;
private string name;
public Person(int id, string name) {
this.id = id;
this.name = name;
}
}
}
Person2.cs
:
using System;
namespace partial_class {
public partial class Person {
public void show() {
Console.WriteLine("Person id = {0}", id);
Console.WriteLine("Person Name = {0}", name);
}
}
}
The content of the Program.cs
containing the main()
function is given below.
Program.cs
:
namespace partial_class {
class Program {
static void Main(string[] args) {
Person p = new Person(1, "Maisam");
p.show();
}
}
}
Output:
Person id = 1
Person Name = Maisam
In the above code examples, we split the code of the Person.cs
class into two files - Person1.cs
and Person2.cs
with the partial
keyword in C#. There are multiple advantages of this approach. We can split large classes with many responsibilities into multiple smaller partial classes having one responsibility each. Multiple developers can work on the same class in 2 different separate files. We can separate front-end design from back-end business logic. Partial classes also provide us a method for efficiently maintaining our code. It also makes our code much cleaner and organized with partial classes.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn