How to Iterate Through a List in C#
-
Use the
for
Loop to Iterate Through a List in C# -
Use the
foreach
Loop to Iterate Through a List in C# -
Use the
ForEach
Method and Lambda Expressions to Iterate Through a List in C# -
Use the
while
Loop and theMoveNext()
Method to Iterate Through a List in C# - Conclusion
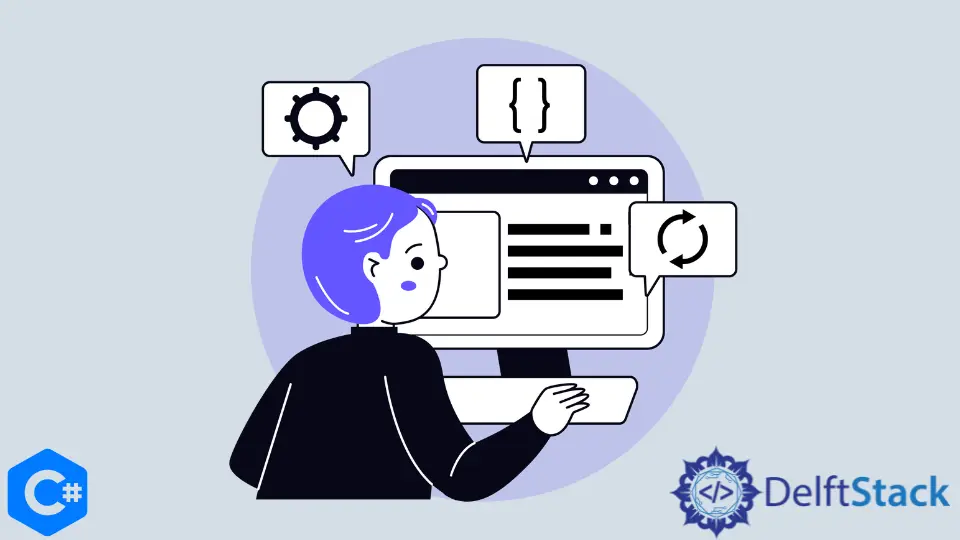
Iterating through a list is a common task in programming, and understanding different approaches can significantly impact code efficiency and readability.
In this article, we will delve into various methods to effectively iterate through a list in C#.
Use the for
Loop to Iterate Through a List in C#
The for
loop is a fundamental construct in C# that repeats a block of code a specified number of times. It is a great choice for iterating through a list.
It follows a specific syntax that allows you to initialize a counter, define a condition for continuation, and specify how the counter should be updated after each iteration.
The general syntax of a for
loop in C# is as follows:
for (initialization; condition; increment) {
// Code to be executed during each iteration
}
Parameters:
- Initialization: This is where you initialize the loop control variable (often referred to as the counter). It’s typically set to an initial value.
- Condition: This is the condition that is checked before each iteration. If the condition evaluates to true, the loop continues; otherwise, it exits.
- Increment: This is the operation that updates the loop control variable after each iteration. It’s usually an increment or decrement operation.
The code snippet below demonstrates how to use the for
loop to iterate through a list in C#:
using System;
using System.Collections.Generic;
namespace iterate_through_a_list {
class Program {
static void Main(string[] args) {
List<string> values = new List<string> { "value1", "value2", "value3" };
for (int i = 0; i < values.Count; i++) {
Console.WriteLine("Element#{0} = {1}", i, values[i]);
}
}
}
}
Output:
Element#0 = value1
Element#1 = value2
Element#2 = value3
In this example, we create a list, values
, containing string elements and iterate through it using the for
loop. The loop uses the values.Count
property as the upper limit, ensuring we iterate over all elements in the list.
Use the foreach
Loop to Iterate Through a List in C#
The foreach
loop is another powerful tool in C# for iterating through a data structure, including lists. It iterates over each element in the data structure without requiring an explicit index or upper limit, allowing you to focus on processing each element within the collection.
Here is the basic syntax of the foreach
loop in C#:
foreach (var item in collection) {
// Code to process each item
}
Parameters:
item
is a variable that represents the current element being processed in the iteration.collection
is the enumerable collection you want to iterate through.
Let’s see how to use the foreach
loop to iterate through a list:
using System;
using System.Collections.Generic;
namespace iterate_through_a_list {
class Program {
static void Main(string[] args) {
List<string> values = new List<string> { "value1", "value2", "value3" };
foreach (var v in values) {
Console.WriteLine("Element = {0}", v);
}
}
}
}
Output:
Element = value1
Element = value2
Element = value3
In this snippet, we utilize the foreach
loop to iterate through the values
list. The loop automatically iterates over each element, simplifying the iteration process.
Use the ForEach
Method and Lambda Expressions to Iterate Through a List in C#
Lambda expressions in C# also offer a concise way to create anonymous functions. They are handy for simplifying and reducing code for repetitive tasks, including iterating through a list.
On the other hand, the ForEach
method is a member of the List<T>
class in C#, used to iterate over all elements in a list and perform a specified action on each element. The method accepts a delegate, typically implemented as a lambda expression or a method, that defines the action to be performed on each element.
The syntax for using the ForEach
method is as follows:
list.ForEach(action);
Here, list
is the instance of the List<T>
class, and action
is the delegate that defines the action to be performed on each element.
Let’s have an example of using the ForEach
method and lambda expressions to iterate through a list:
using System;
using System.Collections.Generic;
namespace iterate_through_a_list {
class Program {
static void Main(string[] args) {
List<string> values = new List<string> { "value1", "value2", "value3" };
values.ForEach((v) => Console.WriteLine("Element = {0}", v));
}
}
}
Output:
Element = value1
Element = value2
Element = value3
In this code, the lambda expression takes an element v
(representing each string in the list) and prints it in a specific format using Console.WriteLine
.
The ForEach
method iterates through each element in the list and applies the action defined by the lambda expression, which, in this case, is printing each element.
Use the while
Loop and the MoveNext()
Method to Iterate Through a List in C#
The MoveNext()
method is commonly used to iterate through a list using an enumerator. An enumerator provides a way to access elements of a collection sequentially without exposing the underlying structure of the collection.
Here, we’ll demonstrate how to use a while
loop in conjunction with the MoveNext()
method to iterate through a list in C#.
using System;
using System.Collections;
using System.Collections.Generic;
namespace IterateWithMoveNext {
class Program {
static void Main(string[] args) {
List<string> values = new List<string> { "value1", "value2", "value3" };
// Get the enumerator for the list
IEnumerator<string> enumerator = values.GetEnumerator();
// Iterate using a while loop and MoveNext()
while (enumerator.MoveNext()) {
string currentValue = enumerator.Current;
Console.WriteLine("Element = {0}", currentValue);
}
}
}
}
Output:
Element = value1
Element = value2
Element = value3
In this example, we first obtain the enumerator for the list using the GetEnumerator()
method. We then use a while
loop and call MoveNext()
on the enumerator.
The MoveNext()
method advances the enumerator to the next element in the collection and returns true
if there is a next element. We can then access the current element using the Current
property of the enumerator.
We efficiently traverse the list and process each element sequentially until there are no more elements to process by using a while
loop in combination with the MoveNext()
method. This technique is particularly useful when you need to iterate through a collection without using a for
or foreach
loop.
Conclusion
This article has explored efficient methods to iterate through a list in C#. We covered the classic for
loop for index-based iteration and the foreach
loop for effortless element traversal.
We also highlighted the ForEach
method with lambda expressions and demonstrated efficient iteration using MoveNext()
with a while
loop and enumerators. By understanding and utilizing these different iteration methods in C#, you can tailor your approach to suit specific programming requirements and optimize the efficiency of your code.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn