Unique Items to List in C#
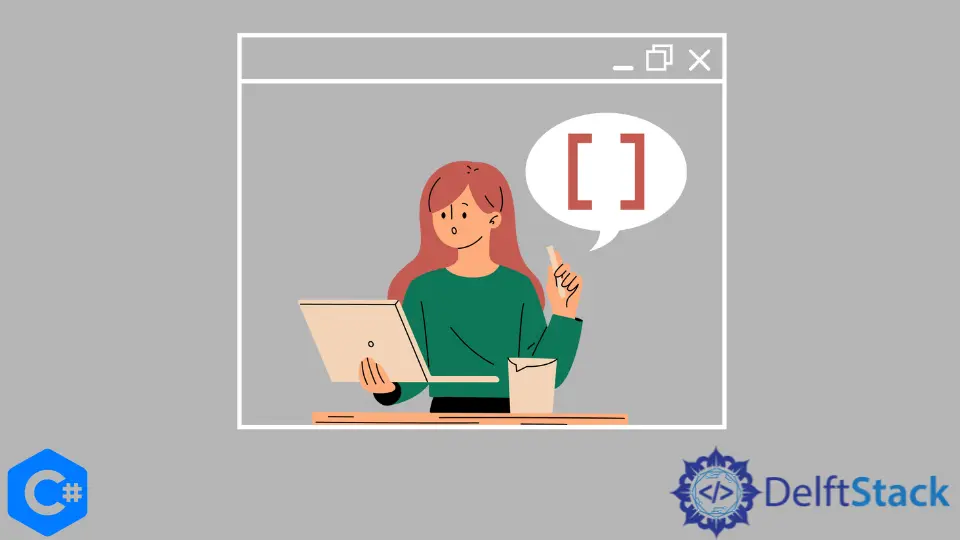
This article will introduce the work of a unique list in programming. In functional programming languages, the list data type is the most adaptable and is used to hold a group of similar data elements.
Arrays in object-oriented programming are related to this concept.
Reasons to Use List
in C#
Separated by comma, list items can be written in square brackets. The List
is used to store data of the same datatype.
There are many strong reasons to use List<T>
, including:
- It falls under
System.Collections.Generics
. TheList
is a dynamic data structure; it is used in such a scenario where the total length of data is unknown, which means that we often useList
when we need to add new data at runtime. - The
List
stores a data group with the same datatype. TheList
is generic; it provides compile-time type checking but does not conduct boxing or unboxing. - The index begins at zero; passing an index such as
ali_List[0]
allows you to access elements. TheList
is speedier and less prone to error thanArrayList
.
Implementation of Unique List in C#
A List
is a dynamic data structure used to store a data group of the same datatype. We use a list in cases where we do not know the total data length.
We also define the data type of list to store related data because it stores data of the same data type. So, it’s important to define the type of list.
One drawback of using list is that it allows us to add a new item even if it is already stored in list. Adding the same data again and again is a waste of memory, so we resolve this issue by resisting a new data item if it is already available in the list.
Work Flow of Unique List in C#
We get items from the user as input. If it is the first item, then we store it, but if it is not the first, then we check if it is already available in the list or not.
If it is available in the list, we do not add it again. But if it is not available, we will add it to the list.
Below is the code to illustrate the concept of List
.
The class Program
is the main class of the program, which have the public static
main method. We created an integer list named numbers
.
We used the while
loop to get values from users as on-demand. The below code is working to avoid adding a new item already available in the list.
{
public static void Main(string[] args) {
List<int> numbers = new List<int>();
Found:
numbers.Clear();
int n = 1;
while (n >= 1) {
Console.WriteLine("Enter any number to add in list (or) Enter 0 to stop this process ");
n = Convert.ToInt32(Console.ReadLine());
if (n >= 1) {
if (numbers.Contains(n)) {
} else {
numbers.Add(n);
}
} else {
}
}
Console.WriteLine("recorded List: ");
foreach (var item in numbers) {
Console.WriteLine(item);
}
Console.WriteLine("do you want to repeat new process 1 for repeat, 0 for close");
int c = Convert.ToInt32(Console.ReadLine());
if (c == 1) {
// Console.Clear();
goto Found;
} else {
Console.Clear();
}
Console.Read();
}
}
Below is the pictorial view of nested classes with output results.
It’s already cleared that the List
makes our program add new items at run time. List
saves our memory, but if we also implement this code in list, it’s an amazing strategy to utilize minimum resources and get good and fast results.