Types of Exceptions Thrown for Invalid Arguments or Parameters in C#
-
Throw
ArgumentException
for Invalid or Unexpected Parameters inC#
-
Throw
ArgumentNullException
for Invalid or Unexpected Parameters inC#
-
Throw
ArgumentOutOfRangeException
for Invalid or Unexpected Parameters inC#
-
Create a User-Defined Exception for Invalid or Unexpected Parameters in
C#
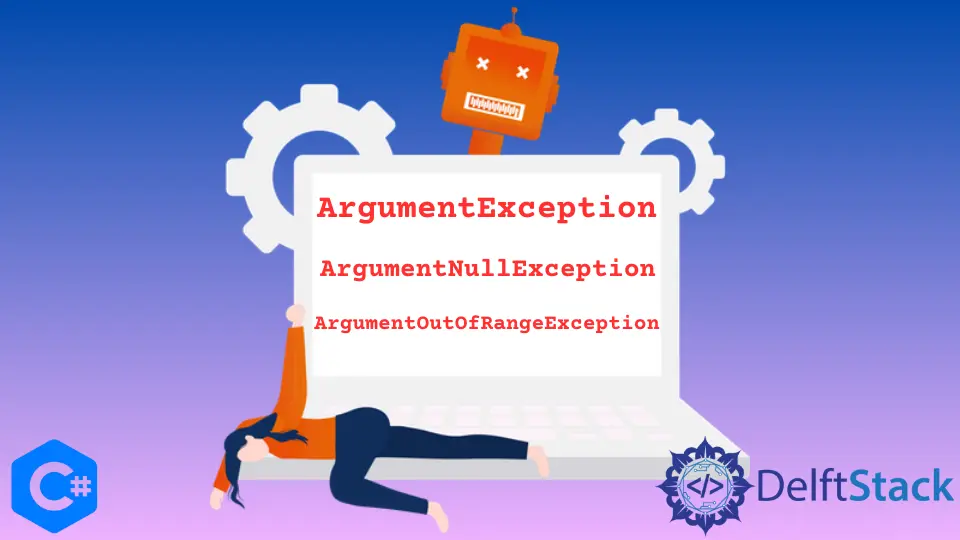
Exceptions provide information about a runtime error in a C# program or a condition that is not expected to occur or violates a system/application constraint. In this tutorial, you will learn three different exceptions related to invalid arguments or parameters and how to throw them in a C# program.
Defining a block of code that throws an exception when it catches an invalid argument or parameter is the easiest way to handle unexpected errors. Bad code or user input can cause errors, and their detection requires an exception thrown by the C# application code or the runtime.
Throw ArgumentException
for Invalid or Unexpected Parameters in C#
The ArgumentException
is thrown when a method receives an invalid argument. It uses the ArgumentException.GetType().Name
property to display exception object’s name.
Using the Message
property, you can display the text of the exception message, which contains the reason behind its occurrence. It’s important that whenever you throw exceptions in C#, the Message
property of that exception must contain a meaningful message to describe the invalid argument and the expected range of values of the argument.
The ArgumentNullException
and ArgumentOutOfRangeException
are the derived classes of ArgumentException
class. The ArgumentException
class itself inherits from the System.SystemException
class.
In general, System.Exception
is a base class of every exception thrown for invalid arguments or parameters in C#.
using System;
namespace ArgumentExceptionExp {
class UserEnrollment {
static void Main(string[] args) {
Console.WriteLine("Please enter your first name and last name for enrollment.");
Console.WriteLine("First Name: ");
var userFirstN = Console.ReadLine();
Console.WriteLine("Last Name: ");
var userLastN = Console.ReadLine();
try {
var userFullN = ShowFullUserName(userFirstN, userLastN);
Console.WriteLine($"Congratulations, {userFullN}!");
} catch (ArgumentException ex) {
Console.WriteLine("Something went wrong, please try again!");
Console.WriteLine(ex);
}
}
static string ShowFullUserName(string userFirstN, string userLastN) {
if (userFirstN == "" || userLastN == "")
throw new ArgumentException(
"Attention, please! Your first or last user name cannot be empty.");
return $"{userFirstN} {userLastN}";
}
}
}
Output:
Please enter your first name and last name for enrollment.
First Name:
Last Name:
Kazmi
Something went wrong, please try again!
System.ArgumentException: Attention, please! Your first or last user name cannot be empty.
at ArgumentExceptionExp.UserEnrollment.ShowFullUserName (System.String userFirstN, System.String userLastN) [0x00020] in <8fb4997067094d929e0f81a3893882f9>:0
at ArgumentExceptionExp.UserEnrollment.Main (System.String[] args) [0x0002f] in <8fb4997067094d929e0f81a3893882f9>:0
In this case, the ArgumentException
will be thrown only when an unexpected or invalid activity prevents a method from completing its normal function. It’s a good programming practice to add information to an exception that is re-thrown to provide more information when debugged.
We can throw an exception using the throw
keyword followed by a new instance derived from the System.Exception
class. The .NET
framework provides many standard pre-defined exception types that you can use to handle invalid arguments and parameters.
Throw ArgumentNullException
for Invalid or Unexpected Parameters in C#
When a method in the C# program receives a null reference that does not accept it as a valid argument requires ArgumentNullException
to be thrown to understand the error. It’s thrown at the run time when an uninstantiated object is passed to a method to prevent the error.
Furthermore, it’s thrown when an object returned from a method call is passed as an argument to a second method, but the value of the original returned object is null
.
Its behavior is identical to the ArgumentException
; however, it uses the HRESULT E_POINTER
, which has the value 0x80004003
.
using System;
namespace TryCatchArgNullExcep {
class ArgNullExpProgram {
static void Main(string[] args) {
try {
var ArgNullEVariable = new ArgNullMember(null, 32);
} catch (ArgumentNullException ex) {
Console.WriteLine("Sorry for the inconvenience, something went wrong...");
Console.WriteLine(ex);
}
}
class ArgNullMember {
public ArgNullMember(string memberName, int memberAge) {
if (memberName == null)
throw new ArgumentNullException(
nameof(memberName), "The member name cannot be `null` or empty. Please, try again!");
MemberName = memberName;
MemberAge = memberAge;
}
public string MemberName { get; private set; }
public int MemberAge { get; private set; }
}
}
}
Output:
Sorry for the inconvenience, something went wrong...
System.ArgumentNullException: The member name cannot be `null` or empty. Please, try again!
Parameter name: memberName
at TryCatchArgNullExcep.ArgNullExpProgram+ArgNullMember..ctor (System.String memberName, System.Int32 memberAge) [0x00010] in <1001b7741efd42ec97508d09c5fc60a2>:0
at TryCatchArgNullExcep.ArgNullExpProgram.Main (System.String[] args) [0x00002] in <1001b7741efd42ec97508d09c5fc60a2>:0
Throw ArgumentOutOfRangeException
for Invalid or Unexpected Parameters in C#
This exception is thrown when the value of an argument is outside the allowable range of values defined by the invoked method in C#. In general, an ArgumentOutOfRangeException
results from the developer error.
Instead of handling it in a try/catch
block, you should eliminate the cause of the exception or validate arguments before passing them to the method.
Classes extensively use it in the System.Collections
and System.IO
namespaces, the array class, and string manipulation methods in the String
class. It’s thrown by the CLR or another class library in C# and indicates developer error.
using System;
namespace ArgumentOutOfRangeExceptionExp {
class userInfo {
static void Main(string[] args) {
try {
Console.WriteLine("Enter User Name: ");
string Hassan = Console.ReadLine();
Console.WriteLine("Enter your Age: ");
int ageU = Int32.Parse(Console.ReadLine());
var user = new userInfoProcess(Hassan, ageU);
} catch (ArgumentOutOfRangeException ex) {
Console.WriteLine("Please be patient, something went wrong.");
Console.WriteLine(ex);
}
}
}
class userInfoProcess {
public userInfoProcess(string userName, int userAge) {
if (userName == null) {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
} else if (userName == "") {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
}
if (userAge < 18 || userAge > 50)
throw new ArgumentOutOfRangeException(
nameof(userAge), "Your age is outside the allowable range, please enter a valid user.");
ArgNullUName = userName;
ArgNullUAge = userAge;
}
public string ArgNullUName { get; private set; }
public int ArgNullUAge { get; private set; }
}
}
Output:
Enter User Name:
Hassan
Enter your Age:
11
Please be patient, something went wrong.
System.ArgumentOutOfRangeException: Your age is outside the allowable range, please enter a valid user.
Parameter name: userAge
at ArgumentOutOfRangeExceptionExp.userInfoProcess..ctor (System.String userName, System.Int32 userAge) [0x00052] in <b199b92ace93448f8cc4c37cc4d5df33>:0
at ArgumentOutOfRangeExceptionExp.userInfo.Main (System.String[] args) [0x00029] in <b199b92ace93448f8cc4c37cc4d5df33>:0
Create a User-Defined Exception for Invalid or Unexpected Parameters in C#
You can also define your exceptions for invalid arguments or parameters in C#. User-defined exception classes are derived from the Exception
class.
Exception handling in C#, supported by the try/catch
and finally
block, is a mechanism to detect and handle code runtime errors.
using System;
namespace UserDefinedExceptionExp {
class ExceptionHandlingC {
static void Main(string[] args) {
string Name = "Hassan";
int Age = 18;
Check temp = new Check();
try {
temp.checkTemp(Name, Age);
} catch (InvalidNameException e) {
Console.WriteLine("InvalidNameException: {0}", e.Message);
}
Console.ReadKey();
}
}
}
public class InvalidNameException : Exception {
public InvalidNameException(string message) : base(message) {}
}
public class Check {
public void checkTemp(string name, int age) {
// this exception will be thrown if the user name will be `Hassan`
if (name == "Hassan") {
throw(new InvalidNameException("User is not valid."));
} else {
Console.WriteLine("You are successfully signed in with the name: {0}", name);
}
}
}
Output:
InvalidNameException: User is not valid.
In this tutorial, you learned three commonly encountered exception types for invalid arguments and parameters and how to create the user-defined exceptions in C#.
The trick is to use and throw the right exception that delivers details about what went wrong, why it is wrong, and how to fix it in a C# program.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub