C# 中为无效参数或参数引发的异常类型
-
为
C#
中的无效或意外参数抛出ArgumentException
-
在
C#
中为无效或意外的参数抛出ArgumentNullException
-
在
C#
中为无效或意外参数抛出ArgumentOutOfRangeException
-
在
C#
中为无效或意外参数创建用户定义的异常
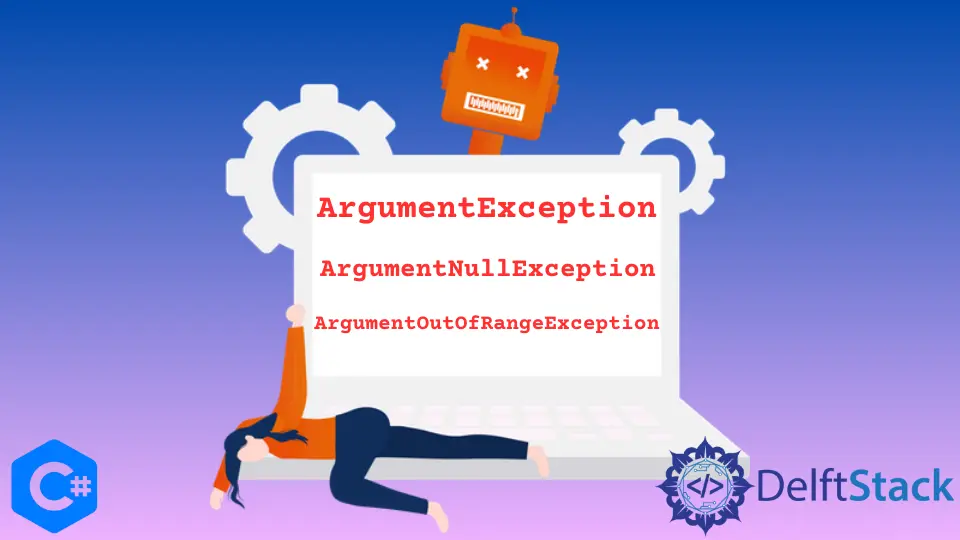
异常提供有关 C# 程序中的运行时错误或预期不会发生或违反系统/应用程序约束的条件的信息。在本教程中,你将学习与无效参数或参数相关的三种不同异常,以及如何在 C# 程序中抛出它们。
定义一个在捕获无效参数或参数时引发异常的代码块是处理意外错误的最简单方法。错误的代码或用户输入可能会导致错误,它们的检测需要 C# 应用程序代码或运行时抛出异常。
为 C#
中的无效或意外参数抛出 ArgumentException
当方法接收到无效参数时,会引发 ArgumentException
。它使用 ArgumentException.GetType().Name
属性来显示异常对象的名称。
使用 Message
属性,你可以显示异常消息的文本,其中包含其发生背后的原因。重要的是,每当你在 C# 中引发异常时,该异常的 Message
属性必须包含有意义的消息来描述无效参数和参数的预期值范围。
ArgumentNullException
和 ArgumentOutOfRangeException
是 ArgumentException
类的派生类。ArgumentException
类本身继承自 System.SystemException
类。
通常,System.Exception
是 C# 中为无效参数或参数引发的每个异常的基类。
using System;
namespace ArgumentExceptionExp {
class UserEnrollment {
static void Main(string[] args) {
Console.WriteLine("Please enter your first name and last name for enrollment.");
Console.WriteLine("First Name: ");
var userFirstN = Console.ReadLine();
Console.WriteLine("Last Name: ");
var userLastN = Console.ReadLine();
try {
var userFullN = ShowFullUserName(userFirstN, userLastN);
Console.WriteLine($"Congratulations, {userFullN}!");
} catch (ArgumentException ex) {
Console.WriteLine("Something went wrong, please try again!");
Console.WriteLine(ex);
}
}
static string ShowFullUserName(string userFirstN, string userLastN) {
if (userFirstN == "" || userLastN == "")
throw new ArgumentException(
"Attention, please! Your first or last user name cannot be empty.");
return $"{userFirstN} {userLastN}";
}
}
}
输出:
Please enter your first name and last name for enrollment.
First Name:
Last Name:
Kazmi
Something went wrong, please try again!
System.ArgumentException: Attention, please! Your first or last user name cannot be empty.
at ArgumentExceptionExp.UserEnrollment.ShowFullUserName (System.String userFirstN, System.String userLastN) [0x00020] in <8fb4997067094d929e0f81a3893882f9>:0
at ArgumentExceptionExp.UserEnrollment.Main (System.String[] args) [0x0002f] in <8fb4997067094d929e0f81a3893882f9>:0
在这种情况下,只有当意外或无效活动阻止方法完成其正常功能时,才会抛出 ArgumentException
。将信息添加到重新抛出的异常以在调试时提供更多信息是一种很好的编程实践。
我们可以使用 throw
关键字引发异常,后跟从 System.Exception
类派生的新实例。 .NET
框架提供了许多标准的预定义异常类型,你可以使用它们来处理无效的参数和参数。
在 C#
中为无效或意外的参数抛出 ArgumentNullException
当 C# 程序中的方法接收到不接受它作为有效参数的空引用时,需要抛出 ArgumentNullException
以了解错误。当将未实例化的对象传递给方法以防止错误时,它会在运行时引发。
此外,当从方法调用返回的对象作为参数传递给第二个方法时,它会被抛出,但原始返回对象的值为 null
。
它的行为与 ArgumentException
相同;但是,它使用 HRESULT E_POINTER
,其值为 0x80004003
。
using System;
namespace TryCatchArgNullExcep {
class ArgNullExpProgram {
static void Main(string[] args) {
try {
var ArgNullEVariable = new ArgNullMember(null, 32);
} catch (ArgumentNullException ex) {
Console.WriteLine("Sorry for the inconvenience, something went wrong...");
Console.WriteLine(ex);
}
}
class ArgNullMember {
public ArgNullMember(string memberName, int memberAge) {
if (memberName == null)
throw new ArgumentNullException(
nameof(memberName), "The member name cannot be `null` or empty. Please, try again!");
MemberName = memberName;
MemberAge = memberAge;
}
public string MemberName { get; private set; }
public int MemberAge { get; private set; }
}
}
}
输出:
Sorry for the inconvenience, something went wrong...
System.ArgumentNullException: The member name cannot be `null` or empty. Please, try again!
Parameter name: memberName
at TryCatchArgNullExcep.ArgNullExpProgram+ArgNullMember..ctor (System.String memberName, System.Int32 memberAge) [0x00010] in <1001b7741efd42ec97508d09c5fc60a2>:0
at TryCatchArgNullExcep.ArgNullExpProgram.Main (System.String[] args) [0x00002] in <1001b7741efd42ec97508d09c5fc60a2>:0
在 C#
中为无效或意外参数抛出 ArgumentOutOfRangeException
当参数的值超出 C# 中调用的方法定义的允许值范围时,将引发此异常。通常,开发人员错误会导致 ArgumentOutOfRangeException
。
与其在 try/catch
块中处理它,你应该消除异常的原因或在将参数传递给方法之前验证参数。
类在 System.Collections
和 System.IO
命名空间、数组类和 String
类中的字符串操作方法中广泛使用它。它由 CLR 或 C# 中的其他类库引发,并指示开发人员错误。
using System;
namespace ArgumentOutOfRangeExceptionExp {
class userInfo {
static void Main(string[] args) {
try {
Console.WriteLine("Enter User Name: ");
string Hassan = Console.ReadLine();
Console.WriteLine("Enter your Age: ");
int ageU = Int32.Parse(Console.ReadLine());
var user = new userInfoProcess(Hassan, ageU);
} catch (ArgumentOutOfRangeException ex) {
Console.WriteLine("Please be patient, something went wrong.");
Console.WriteLine(ex);
}
}
}
class userInfoProcess {
public userInfoProcess(string userName, int userAge) {
if (userName == null) {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
} else if (userName == "") {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
}
if (userAge < 18 || userAge > 50)
throw new ArgumentOutOfRangeException(
nameof(userAge), "Your age is outside the allowable range, please enter a valid user.");
ArgNullUName = userName;
ArgNullUAge = userAge;
}
public string ArgNullUName { get; private set; }
public int ArgNullUAge { get; private set; }
}
}
输出:
Enter User Name:
Hassan
Enter your Age:
11
Please be patient, something went wrong.
System.ArgumentOutOfRangeException: Your age is outside the allowable range, please enter a valid user.
Parameter name: userAge
at ArgumentOutOfRangeExceptionExp.userInfoProcess..ctor (System.String userName, System.Int32 userAge) [0x00052] in <b199b92ace93448f8cc4c37cc4d5df33>:0
at ArgumentOutOfRangeExceptionExp.userInfo.Main (System.String[] args) [0x00029] in <b199b92ace93448f8cc4c37cc4d5df33>:0
在 C#
中为无效或意外参数创建用户定义的异常
你还可以为 C# 中的无效参数或参数定义异常。用户定义的异常类派生自 Exception
类。
C# 中的异常处理由 try/catch
和 finally
块支持,是一种检测和处理代码运行时错误的机制。
using System;
namespace UserDefinedExceptionExp {
class ExceptionHandlingC {
static void Main(string[] args) {
string Name = "Hassan";
int Age = 18;
Check temp = new Check();
try {
temp.checkTemp(Name, Age);
} catch (InvalidNameException e) {
Console.WriteLine("InvalidNameException: {0}", e.Message);
}
Console.ReadKey();
}
}
}
public class InvalidNameException : Exception {
public InvalidNameException(string message) : base(message) {}
}
public class Check {
public void checkTemp(string name, int age) {
// this exception will be thrown if the user name will be `Hassan`
if (name == "Hassan") {
throw(new InvalidNameException("User is not valid."));
} else {
Console.WriteLine("You are successfully signed in with the name: {0}", name);
}
}
}
输出:
InvalidNameException: User is not valid.
在本教程中,你学习了三种常见的无效实参和参数异常类型,以及如何在 C# 中创建用户定义的异常。
诀窍是使用并抛出正确的异常,该异常可提供有关出错原因、出错原因以及如何在 C# 程序中修复它的详细信息。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub