C# の無効な引数またはパラメーターに対してスローされる例外の種類
-
C#
の無効または予期しないパラメータに対してArgumentException
をスローする -
C#
の無効または予期しないパラメータに対してArgumentNullException
をスローする -
C#
の無効または予期しないパラメーターに対してArgumentOutOfRangeException
をスローする -
C#
の無効または予期しないパラメーターに対してユーザー定義の例外を作成する
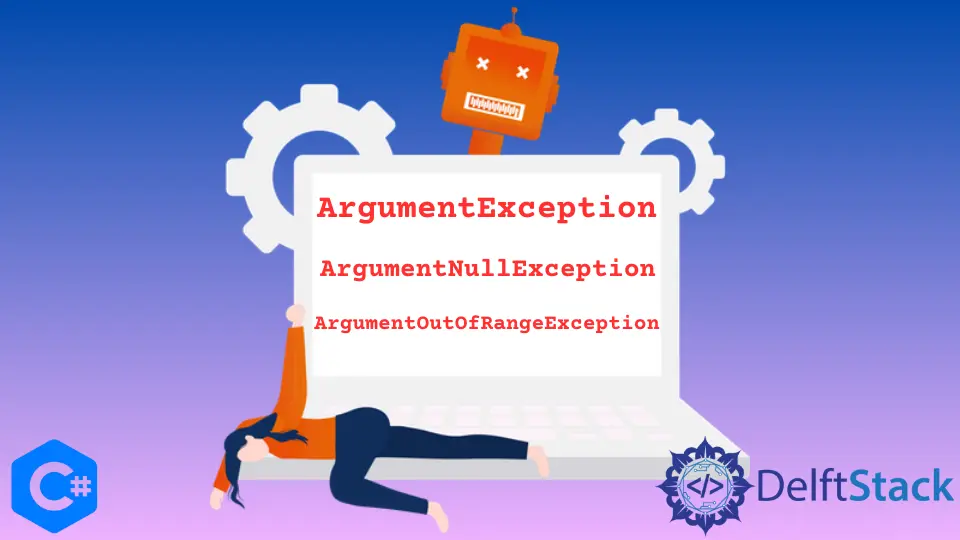
例外は、C# プログラムの実行時エラー、または発生することが予期されていない、またはシステム/アプリケーションの制約に違反している状態に関する情報を提供します。このチュートリアルでは、無効な引数またはパラメーターに関連する 3つの異なる例外と、それらを C# プログラムでスローする方法を学習します。
無効な引数またはパラメーターをキャッチしたときに例外をスローするコードのブロックを定義することは、予期しないエラーを処理する最も簡単な方法です。不正なコードまたはユーザー入力はエラーを引き起こす可能性があり、それらの検出には、C# アプリケーションコードまたはランタイムによってスローされる例外が必要です。
C#
の無効または予期しないパラメータに対して ArgumentException
をスローする
メソッドが無効な引数を受け取ると、ArgumentException
がスローされます。ArgumentException.GetType().Name
プロパティを使用して、例外オブジェクトの名前を表示します。
Message
プロパティを使用すると、例外メッセージのテキストを表示できます。これには、その発生の背後にある理由が含まれています。C# で例外をスローするときは常に、その例外の Message
プロパティに、無効な引数と引数の予想される値の範囲を説明する意味のあるメッセージが含まれている必要があります。
ArgumentNullException
および ArgumentOutOfRangeException
は、ArgumentException
クラスの派生クラスです。ArgumentException
クラス自体は、System.SystemException
クラスを継承します。
一般に、System.Exception
は、C# の無効な引数またはパラメーターに対してスローされるすべての例外の基本クラスです。
using System;
namespace ArgumentExceptionExp {
class UserEnrollment {
static void Main(string[] args) {
Console.WriteLine("Please enter your first name and last name for enrollment.");
Console.WriteLine("First Name: ");
var userFirstN = Console.ReadLine();
Console.WriteLine("Last Name: ");
var userLastN = Console.ReadLine();
try {
var userFullN = ShowFullUserName(userFirstN, userLastN);
Console.WriteLine($"Congratulations, {userFullN}!");
} catch (ArgumentException ex) {
Console.WriteLine("Something went wrong, please try again!");
Console.WriteLine(ex);
}
}
static string ShowFullUserName(string userFirstN, string userLastN) {
if (userFirstN == "" || userLastN == "")
throw new ArgumentException(
"Attention, please! Your first or last user name cannot be empty.");
return $"{userFirstN} {userLastN}";
}
}
}
出力:
Please enter your first name and last name for enrollment.
First Name:
Last Name:
Kazmi
Something went wrong, please try again!
System.ArgumentException: Attention, please! Your first or last user name cannot be empty.
at ArgumentExceptionExp.UserEnrollment.ShowFullUserName (System.String userFirstN, System.String userLastN) [0x00020] in <8fb4997067094d929e0f81a3893882f9>:0
at ArgumentExceptionExp.UserEnrollment.Main (System.String[] args) [0x0002f] in <8fb4997067094d929e0f81a3893882f9>:0
この場合、ArgumentException
は、予期しないアクティビティまたは無効なアクティビティによってメソッドが通常の機能を完了できない場合にのみスローされます。デバッグ時に詳細情報を提供するために再スローされる例外に情報を追加することは、プログラミングの良い習慣です。
throw
キーワードの後にSystem.Exception
クラスから派生した新しいインスタンスを使用して、例外をスローできます。 .NET
フレームワークは、無効な引数とパラメーターを処理するために使用できる多くの標準の事前定義された例外タイプを提供します。
C#
の無効または予期しないパラメータに対して ArgumentNullException
をスローする
C# プログラムのメソッドが、有効な引数として受け入れない null 参照を受け取った場合、エラーを理解するために ArgumentNullException
をスローする必要があります。エラーを防ぐために、インスタンス化されていないオブジェクトがメソッドに渡されたときにスローされます。
さらに、メソッド呼び出しから返されたオブジェクトが引数として 2 番目のメソッドに渡されたときにスローされますが、元の返されたオブジェクトの値は null
です。
その動作は ArgumentException
と同じです。ただし、値が 0x80004003
の HRESULT E_POINTER
を使用します。
using System;
namespace TryCatchArgNullExcep {
class ArgNullExpProgram {
static void Main(string[] args) {
try {
var ArgNullEVariable = new ArgNullMember(null, 32);
} catch (ArgumentNullException ex) {
Console.WriteLine("Sorry for the inconvenience, something went wrong...");
Console.WriteLine(ex);
}
}
class ArgNullMember {
public ArgNullMember(string memberName, int memberAge) {
if (memberName == null)
throw new ArgumentNullException(
nameof(memberName), "The member name cannot be `null` or empty. Please, try again!");
MemberName = memberName;
MemberAge = memberAge;
}
public string MemberName { get; private set; }
public int MemberAge { get; private set; }
}
}
}
出力:
Sorry for the inconvenience, something went wrong...
System.ArgumentNullException: The member name cannot be `null` or empty. Please, try again!
Parameter name: memberName
at TryCatchArgNullExcep.ArgNullExpProgram+ArgNullMember..ctor (System.String memberName, System.Int32 memberAge) [0x00010] in <1001b7741efd42ec97508d09c5fc60a2>:0
at TryCatchArgNullExcep.ArgNullExpProgram.Main (System.String[] args) [0x00002] in <1001b7741efd42ec97508d09c5fc60a2>:0
C#
の無効または予期しないパラメーターに対して ArgumentOutOfRangeException
をスローする
この例外は、引数の値が C# で呼び出されたメソッドによって定義された値の許容範囲外にある場合にスローされます。一般に、ArgumentOutOfRangeException
は開発者エラーが原因で発生します。
try/catch
ブロックで処理する代わりに、例外の原因を取り除くか、引数をメソッドに渡す前に検証する必要があります。
クラスは、System.Collections
および System.IO
名前空間、配列クラス、および String
クラスの文字列操作メソッドで広く使用されています。これは、CLR または C# の別のクラスライブラリによってスローされ、開発者エラーを示します。
using System;
namespace ArgumentOutOfRangeExceptionExp {
class userInfo {
static void Main(string[] args) {
try {
Console.WriteLine("Enter User Name: ");
string Hassan = Console.ReadLine();
Console.WriteLine("Enter your Age: ");
int ageU = Int32.Parse(Console.ReadLine());
var user = new userInfoProcess(Hassan, ageU);
} catch (ArgumentOutOfRangeException ex) {
Console.WriteLine("Please be patient, something went wrong.");
Console.WriteLine(ex);
}
}
}
class userInfoProcess {
public userInfoProcess(string userName, int userAge) {
if (userName == null) {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
} else if (userName == "") {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
}
if (userAge < 18 || userAge > 50)
throw new ArgumentOutOfRangeException(
nameof(userAge), "Your age is outside the allowable range, please enter a valid user.");
ArgNullUName = userName;
ArgNullUAge = userAge;
}
public string ArgNullUName { get; private set; }
public int ArgNullUAge { get; private set; }
}
}
出力:
Enter User Name:
Hassan
Enter your Age:
11
Please be patient, something went wrong.
System.ArgumentOutOfRangeException: Your age is outside the allowable range, please enter a valid user.
Parameter name: userAge
at ArgumentOutOfRangeExceptionExp.userInfoProcess..ctor (System.String userName, System.Int32 userAge) [0x00052] in <b199b92ace93448f8cc4c37cc4d5df33>:0
at ArgumentOutOfRangeExceptionExp.userInfo.Main (System.String[] args) [0x00029] in <b199b92ace93448f8cc4c37cc4d5df33>:0
C#
の無効または予期しないパラメーターに対してユーザー定義の例外を作成する
C# で無効な引数またはパラメーターの例外を定義することもできます。ユーザー定義の例外クラスは、Exception
クラスから派生します。
try/catch
および finally
ブロックでサポートされている C# での例外処理は、コードのランタイムエラーを検出して処理するメカニズムです。
using System;
namespace UserDefinedExceptionExp {
class ExceptionHandlingC {
static void Main(string[] args) {
string Name = "Hassan";
int Age = 18;
Check temp = new Check();
try {
temp.checkTemp(Name, Age);
} catch (InvalidNameException e) {
Console.WriteLine("InvalidNameException: {0}", e.Message);
}
Console.ReadKey();
}
}
}
public class InvalidNameException : Exception {
public InvalidNameException(string message) : base(message) {}
}
public class Check {
public void checkTemp(string name, int age) {
// this exception will be thrown if the user name will be `Hassan`
if (name == "Hassan") {
throw(new InvalidNameException("User is not valid."));
} else {
Console.WriteLine("You are successfully signed in with the name: {0}", name);
}
}
}
出力:
InvalidNameException: User is not valid.
このチュートリアルでは、無効な引数とパラメーターで一般的に発生する 3つの例外タイプと、C# でユーザー定義の例外を作成する方法を学習しました。
秘訣は、何がうまくいかなかったのか、なぜ間違っているのか、C# プログラムで修正する方法についての詳細を提供する正しい例外を使用してスローすることです。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub