How to Catch Multiple Exceptions in C#
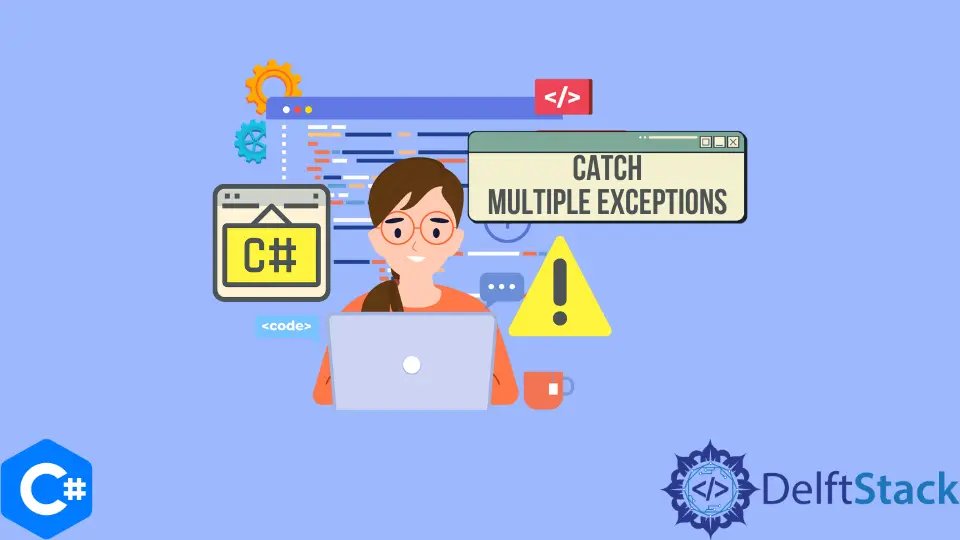
When programming in C#, handling exceptions effectively is crucial for creating robust applications. Sometimes, your code might encounter different types of exceptions that need to be addressed simultaneously. Fortunately, C# provides flexible methods to catch multiple exceptions, ensuring that your application can manage various error scenarios gracefully.
In this article, we’ll explore two primary methods: using the Exception class and implementing conditional logic within the catch clause. By the end, you’ll have a clear understanding of how to catch multiple exceptions in C#, enabling you to write cleaner and more efficient code.
Using the Exception Class
One of the simplest ways to catch multiple exceptions in C# is by leveraging the base Exception class. This method allows you to catch any exception that derives from the Exception class, which includes virtually all exceptions in C#. By using a single catch block, you can handle multiple exceptions without needing to specify each one individually.
Here’s how you can implement this method:
try
{
// Code that may throw multiple exceptions
int[] numbers = { 1, 2, 3 };
Console.WriteLine(numbers[5]); // This will throw an IndexOutOfRangeException
int result = 10 / 0; // This will throw a DivideByZeroException
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
Output:
An error occurred: Index was outside the bounds of the array.
This code snippet demonstrates a try-catch block where we attempt to access an out-of-bounds index in an array and perform a division by zero. Instead of having separate catch blocks for each exception type, we catch all exceptions with the base Exception class. This method is particularly useful when you want to log errors or perform general error handling without needing to differentiate between exception types.
However, it’s essential to use this approach judiciously. Catching all exceptions can sometimes obscure the specific issues in your code, making debugging more challenging. Therefore, it’s advisable to use this method when you want a broad catch-all for error handling, particularly in scenarios where the specific exception type isn’t critical to your application’s flow.
Using Conditional Logic in Catch Clauses
Another effective method for catching multiple exceptions in C# is to utilize conditional logic within your catch clauses. By using an if statement, you can check the type of the exception and handle it accordingly. This approach allows you to catch specific exceptions while still managing multiple types of errors in a structured way.
Here’s an example of how to implement this method:
try
{
// Code that may throw multiple exceptions
int[] numbers = { 1, 2, 3 };
Console.WriteLine(numbers[5]); // This will throw an IndexOutOfRangeException
int result = 10 / 0; // This will throw a DivideByZeroException
}
catch (IndexOutOfRangeException ex)
{
Console.WriteLine($"Index error: {ex.Message}");
}
catch (DivideByZeroException ex)
{
Console.WriteLine($"Division error: {ex.Message}");
}
catch (Exception ex)
{
Console.WriteLine($"An unexpected error occurred: {ex.Message}");
}
Output:
Index error: Index was outside the bounds of the array.
In this snippet, we have three catch blocks—one for each specific exception type and a final catch block for any unexpected exceptions. This method provides a more granular level of error handling, allowing you to tailor responses based on the specific exception encountered.
Using conditional logic in your catch clauses can enhance the maintainability of your code. It allows you to implement different recovery strategies or logging mechanisms based on the type of error. This way, you can provide more informative feedback to the user or take specific actions depending on the nature of the exception.
However, be cautious not to overcomplicate your error handling. Too many catch blocks can make your code harder to read and maintain. Aim for a balance between specificity and simplicity, ensuring that your code remains clear and easy to follow.
Conclusion
Catching multiple exceptions in C# is a fundamental skill that every developer should master. Whether you choose to use the base Exception class for a broad catch-all approach or implement conditional logic for more specific error handling, both methods have their merits. By understanding and applying these techniques, you can enhance the robustness of your applications, providing a better experience for users while simplifying your debugging process. Remember, effective exception handling is not just about catching errors; it’s about managing them in a way that keeps your application running smoothly.
FAQ
-
What is the purpose of catching exceptions in C#?
Catching exceptions allows developers to handle errors gracefully, preventing applications from crashing and providing a better user experience. -
Can I catch multiple exceptions in a single catch block?
Yes, you can catch multiple exceptions in a single catch block using the base Exception class, which captures all exceptions derived from it. -
Is it better to catch specific exceptions or use a general catch-all?
It depends on the scenario. Specific catches provide more control and tailored error handling, while a catch-all can simplify code when the exact exception type is less critical. -
How do I log exceptions in C#?
You can log exceptions by writing the exception message and stack trace to a file, database, or logging service within the catch block. -
Can I rethrow an exception after catching it in C#?
Yes, you can rethrow an exception using thethrow;
statement within the catch block, which preserves the original stack trace.
effectively. Discover two main methods: using the Exception class for broad error handling and implementing conditional logic in catch clauses for specific exceptions. Enhance your application’s robustness with these techniques.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn