List of Exceptions in C#
-
System Exceptions in
C#
-
System.Data
Exceptions inC#
-
System.IO
Exceptions inC#
-
System.Net
Exceptions inC#
-
System.Net.Mail
Exceptions inC#
-
System.Net.NetworkInformation
Exceptions inC#
-
System.Resources
Exceptions inC#
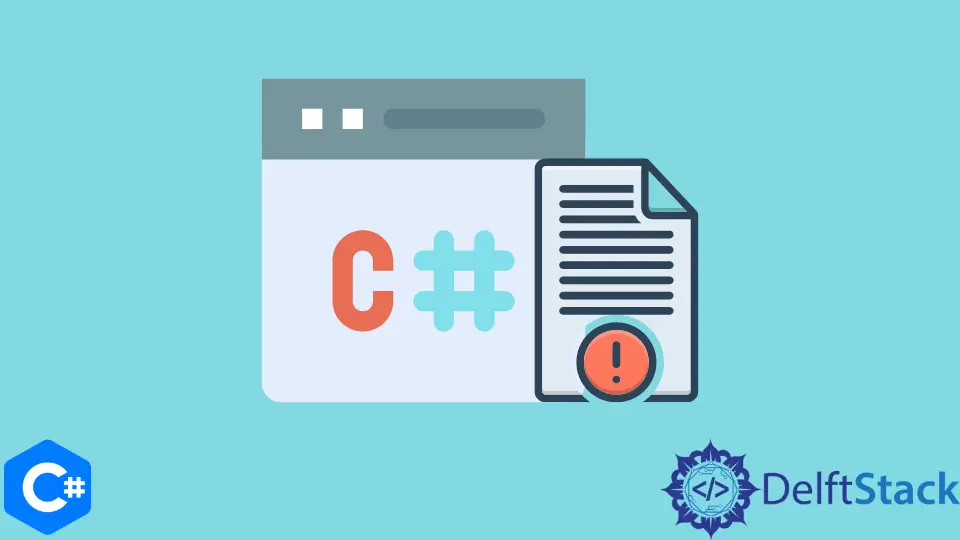
This article is about the exceptions that come with pre-defined C#’s Exception
class.
The System Exception
class is a pre-defined Exception class in the C# programming language that is available in programming. Select an exception that might arise from your code and insert it into the appropriate catch block.
This chapter will provide a comprehensive list of all system exception classes. You may use this exception to write code that is both errors free and very resilient.
System Exceptions in C#
- AccessViolationException - The exception is generated whenever an attempt to read from or write to protected memory.
- AppDomainUnloadedException - An exception is generated whenever an attempt to access an application domain has not yet been loaded.
- ApplicationException - The exception will be thrown if an application error is not fatal.
- ArgumentException - The error message is shown to the user when one of the parameters supplied to a method is invalid.
- ArgumentNullException - The exception is generated whenever a procedure that does not accept a null reference as a valid parameter receives a reference to a null value (referred to as Nothing in Visual Basic).
- ArgumentOutOfRangeException - When an argument’s value falls outside the range of permissible values established by the method that has been called, the exception is thrown.
- ArithmeticException - The exception is thrown if a computation, casting, or conversion action results in an error.
- ArrayTypeMismatchException - When an attempt is made to save an element of the incorrect type inside an array, the exception is issued.
- BadImageFormatException - When a dynamic link library (DLL) or an executable program has a corrupted file image, the exception is raised.
- CannotUnloadAppDomainException - If a failed effort is made to unload an application domain, the exception is thrown.
- ContextMarshalException - An exception is thrown if an object cannot be marshaled over a context border.
- DataMisalignedException - If a unit of data is read from or written to an address that isn’t a multiple of the data size, an exception is thrown. When the data size is not a multiple of the address, an exception is thrown.
- DivideByZeroException - The exception is produced whenever an attempt is made to divide a decimal number or an integral value by zero.
- DllNotFoundException - The exception is if a dynamic link library (DLL) specified in a DLL import cannot be located.
- DuplicateWaitObjectException - An exception is thrown if an array contains numerous instances of the same object.
- EntryPointNotFoundException - The exception is produced if a failed attempt to load a class because the lack of an entry method causes the class to be loaded.
- ExecutionEngineException - The exception is raised whenever the common language runtime’s execution engine encounters an error local to the system.
- FieldAccessException - The exception is generated whenever an invalid attempt is made to access a private or protected field that is included inside a class.
- FormatException - This occurs when the structure of an argument does not match the parameters supplied by the method call.
- IndexOutOfRangeException - The exception is issued if an attempt is made to access an element of an array with an index beyond the array’s limits. This class cannot have its inheritance passed down to other classes.
- InsufficientMemoryException - The exception is triggered if a check for adequate, accessible memory returns an incorrect result. This class cannot have its inheritance passed down to other classes.
- InvalidCastException - The exception will be triggered if the casting procedure is faulty or an explicit conversion is done.
- InvalidOperationException - The exception is raised whenever a method call cannot be carried out because of the present state of the object.
- InvalidProgramException - If a program has incorrect Microsoft Intermediate Language (MSIL) or metadata, the exception is raised. In most cases, this is an indication that there is a problem with the compiler that was used to build the program.
- MemberAccessException - The exception is raised whenever an unsuccessful attempt is made to access a class member.
- MethodAccessException - The exception occurs whenever an invalid attempt is made to access a private or protected method inside a class.
- MissingFieldException - The exception is generated whenever someone attempts to dynamically access a field that does not exist in the database.
- MissingMemberException - The exception is issued if there is an attempt to dynamically access a class member that does not exist.
- MissingMethodException - The exception is if someone attempts to dynamically access a method that does not exist in the program.
- MulticastNotSupportedException - The exception is raised if a combination of two delegates based on the type rather than the type is attempted.
- NotFiniteNumberException - Any value that is either positive or negative infinity, or does not exist, will cause an exception to be raised (NaN).
- NotImplementedException - The exception is raised if a requested method or action cannot be carried out because it is not implemented.
- NotSupportedException - The exception is raised when a method that has been called does not support the functionality that has been invoked or when an attempt is made to read, seek, or write to a stream that doesn’t support the functionality that has been invoked.
- NullReferenceException - The error is raised if a de-reference operation is attempted on an object reference that has been previously configured to
null
. - ObjectDisposedException - The exception is generated if an operation is carried out on an object that has been disposed of.
- OperationCanceledException - The exception is raised in a thread if the thread is stopped from carrying out an activity previously carried out.
- OutOfMemoryException - The exception is raised if there is insufficient memory to continue the execution of a program. Also known as the memory exception.
- OverflowException - The exception is raised if an action involving casting, conversion, or arithmetic results in an overflow when performed in a checked context.
- PlatformNotSupportedException - The exception is raised if a feature is attempted to be executed on a platform for which it is not supported.
- RankException - The exception is raised if a method receives a parameter with an array with an incorrect number of dimensions.
- StackOverflowException - The exception is raised if the execution stack overflows due to having an excessive number of nested method calls.
- SystemException - This function specifies the base class for any exceptions established inside the namespace.
- TimeoutException - The exception is issued if the amount of time permitted for a procedure or action has run out.
- TypeInitializationException - The exception thrown around is the exception thrown by the class initializer when it is wrapped up and thrown.
- TypeLoadException - The exception is raised if there is a problem with the type loading.
- TypeUnloadedException - The exception is generated if a class that has not yet been loaded is attempted to be accessed.
- UnauthorizedAccessException - The exception is raised if the operating system refuses to provide access because of a problem with input or output (I/O) or a particular kind of security fault.
System.Data
Exceptions in C#
- ConstraintException - This object represents the exception that is raised when an action is attempted that would violate a constraint.
- DataException - The exception is delivered if an error is produced by utilizing
ADO.NET
components. - DBConcurrencyException - The exception is issued when doing an insert, update, or delete action if the number of rows impacted is equal to zero.
- DeletedRowInaccessibleException - It is used to represent the exception that is raised if an action is attempted to be performed on a that has been removed.
- DuplicateNameException - Represents the exception produced if an add operation is performed on a linked object and a database object name is found to be duplicated in use.
- EvaluateException - A representation of the exception is raised when a property evaluation cannot occur.
- InRowChangingEventException - Represents the error message shown when the method is called from inside the event.
- InvalidConstraintException - The exception is produced if an attempt is made to access or construct a relation improperly is represented by this class.
- InvalidExpressionException - This object represents the error produced when an attempt is made to add a DataColumn to a DataColumnCollection when the DataColumn includes an incorrect Expression.
- MissingPrimaryKeyException - When a row in a table that doesn’t have a primary key is tried to be accessed, this exception is thrown.
- NoNullAllowedException - The exception will be issued if you attempt to insert a null value into a column with the property set to false where it should not be.
- OperationAbortedException - This exception is produced if a user cancels a currently running action.
- ReadOnlyException - The exception is produced whenever an attempt is made to modify the value of a column that is restricted to read-only access.
- RowNotInTableException - The exception is raised when you attempt to execute an action on a that is not included in a. Represents the error message that is shown when the exception is thrown.
- StrongTypingException - The exception is generated by a highly typed system whenever a user attempts to access a DBNull variable.
- SyntaxErrorException - The exception is thrown when the property of a has a syntax mistake is represented by this object.
- TypedDataSetGeneratorException - If a name conflict arises when a strongly typed is generated, the exception is raised.
- VersionNotFoundException - The exception is raised if an attempt is made to return a version that has since been removed from existence.
System.IO
Exceptions in C#
- DirectoryNotFoundException - The exception is generated if a section of a file or directory cannot be located.
- DriveNotFoundException - The exception is raised if a disk or share is attempted to be accessed that is currently unavailable.
- EndOfStreamException - The exception is produced if an attempt is made to read data that has been written beyond the end of a stream.
- FileLoadException - This exception is issued if a managed assembly is located but cannot be loaded.
- FileNotFoundException - If an unsuccessful attempt is made to read from or write to a file that doesn’t exist on the disk, an exception is thrown.
- IOException - The exception is raised if there is a problem with the I/O.
- PathTooLongException - The error is issued if a pathname or filename is larger than the maximum length that has been pre-defined by the system.
System.Net
Exceptions in C#
- HttpListenerException - If something goes wrong while processing an HTTP request, the exception is thrown.
- ProtocolViolationException - An exception is thrown if a network protocol is utilized improperly because of the error.
- WebException - The exception is issued if there is a problem when attempting to contact the network through a pluggable protocol.
System.Net.Mail
Exceptions in C#
- SmtpException - Identifies the error condition that causes the to throw an exception when it cannot act.
- SmtpFailedRecipientException - This object represents the exception issued when the can’t finish sending a message or performing an action to a certain receiver.
- SmtpFailedRecipientsException - The exception is generated if an email is sent using a and cannot be sent to all recipients.
System.Net.NetworkInformation
Exceptions in C#
- NetworkInformationException - The exception is raised if there is a failure in getting network information.
- PingException - The exception is generated whenever a method (or method) calls another method (or method) that generates an exception.
System.Resources
Exceptions in C#
- MissingManifestResourceException - If the satellite assembly does not have enough resources for the neutral culture, an exception will be made because there is not enough room for a satellite assembly.
- MissingSatelliteAssemblyException - This rule does not apply when the neutral culture’s satellite assembly is not present, which is the only time it is appropriate to break it.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn