C#에서 여러 예외 포착
Muhammad Maisam Abbas
2023년10월12일
Csharp
Csharp Exception
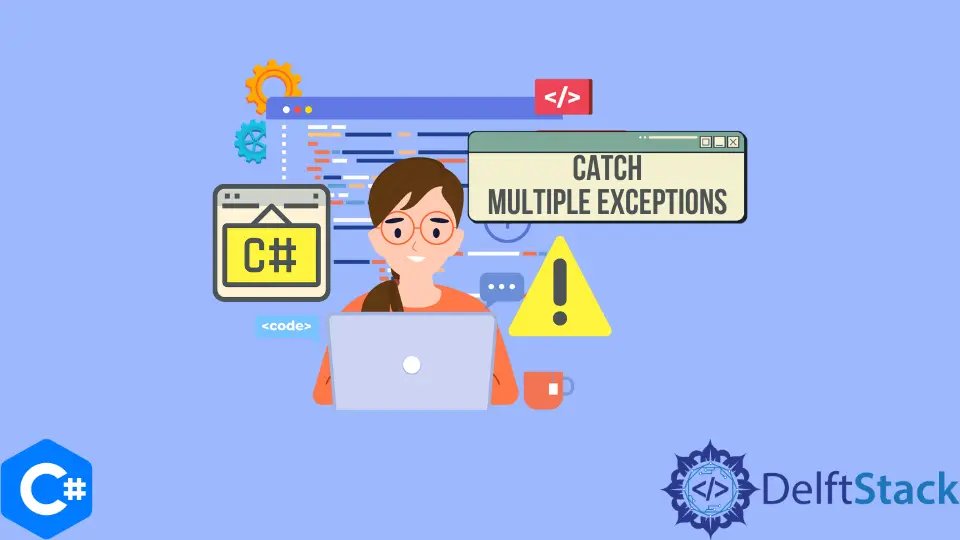
이 자습서에서는 C#에서 여러 예외를 포착하는 방법에 대해 설명합니다.
C#에서Exception
클래스를 사용하여 여러 예외 포착
Exception
클래스는 C#에서 일반 예외를 나타내는 데 사용됩니다. try...catch
절 내에서Exception
클래스를 사용하여 코드에서 발생한 모든 유형의 예외를 포착 할 수 있습니다. 다음 예제 코드를 참조하십시오.
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
try {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
} catch (Exception e) {
Console.WriteLine("An Exception Occured");
}
}
}
}
출력:
True
위의 코드에서 C#의Exception
클래스를 사용하여 코드에서 발생한 모든 유형의 예외를 포착합니다. 이 접근 방식은 문제와 문제 해결 방법에 대한 충분한 정보를 제공하지 않기 때문에 일반적으로 권장되지 않습니다. 우리는 항상이 일반적인 예외 유형보다 특정 예외 유형을 선호해야합니다.
C#에서if
문을 사용하여 여러 예외 포착
특정 예외를 사용하려면catch
절의 형태로 많은 코드를 작성해야합니다. if
문을 사용하여 C#에서 하나의catch
절로 여러 유형의 예외를 포착 할 수 있습니다. 다음 예제 코드를 참조하십시오.
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
try {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
} catch (Exception e) {
if (ex is FormatException || ex is OverflowException) {
Console.WriteLine("Format or Overflow Exception occured");
}
}
}
}
}
출력:
True
위의 코드에서는 C#의if
문을 사용하여catch
절 하나만 사용하여FormatException
및OverflowException
예외를 모두 포착합니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn