在 C# 中捕獲多個異常
Muhammad Maisam Abbas
2023年10月12日
Csharp
Csharp Exception
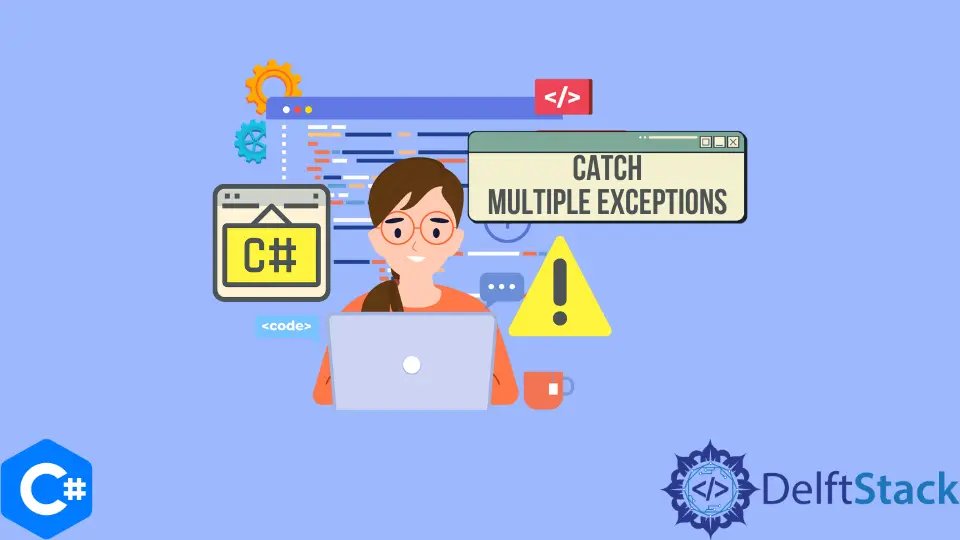
本教程將討論在 C# 中捕獲多個異常的方法。
使用 C# 中的 Exception
類捕獲多個異常
Exception
類用於表示 C# 中的一般異常。我們可以在 try...catch
子句中使用 Exception
類來捕獲程式碼丟擲的任何型別的異常。請參見以下示例程式碼。
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
try {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
} catch (Exception e) {
Console.WriteLine("An Exception Occured");
}
}
}
}
輸出:
True
在上面的程式碼中,我們使用 C# 中的 Exception
類來捕獲程式碼丟擲的任何型別的異常。通常不建議使用此方法,因為它不能為我們提供足夠的有關問題以及如何解決問題的資訊。我們應該始終偏向於特定的異常型別,而不是這種通用的異常型別。
使用 C# 中的 if
語句捕獲多個異常
使用特定的異常要求我們以 catch
子句的形式編寫大量程式碼。在 C# 中,我們可以使用 if
語句只用一個 catch
子句來捕獲多種型別的異常。請參見以下示例程式碼。
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
try {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
} catch (Exception e) {
if (ex is FormatException || ex is OverflowException) {
Console.WriteLine("Format or Overflow Exception occured");
}
}
}
}
}
輸出:
True
在上面的程式碼中,我們使用 C# 中的 if
語句通過一個 catch
子句來捕獲 FormatException
和 OverflowException
異常。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn