How to Initialize a List With Values in C#
-
Use the
List<T>
Class to Initialize a List With Values in C# -
Use the
List.Add()
Method to Initialize a List With Values in C# - Use Collection Initializer to Initialize a List With Values in C#
-
Use the
List.AddRange
Method to Initialize a List With Values in C# - Conclusion
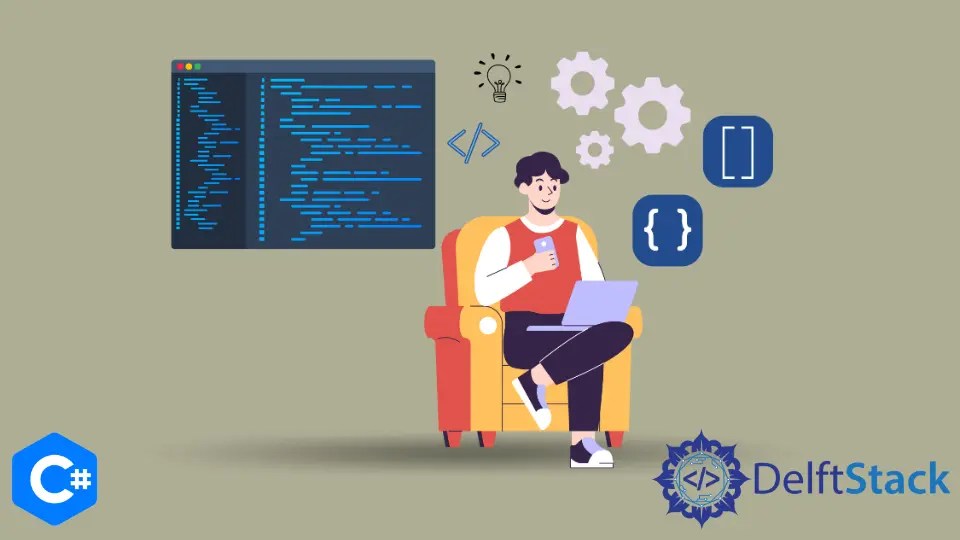
Initializing a list with string values in C# can be achieved through various methods, each offering its advantages in terms of readability, performance, and flexibility.
This tutorial will teach you different methods of initializing a list and handling its implementation details. C# enables programmers to instantiate a list, initialize it with the new
keyword, and perform member assignments in a single statement.
The primary objective of this tutorial is to familiarize the C# developers with the generic lists and their implementation using the List<T>
collection class. The generic List<T>
class belongs to the System.Collections.Generic
namespace and is one of the simplest collection classes defined by the .NET Framework.
A list features a simple ordered list of elements with the capability to store multiple types of objects. The List<T>
class in C# implements the ICollection
, IEnumerable
, IList
, ICollection<T>
, IEnumerable<T>
, and IList<T>
interfaces.
Use the List<T>
Class to Initialize a List With Values in C#
The List<T>
class is part of the System.Collections.Generic
namespace and represents a strongly typed list of objects. It allows for the storage and manipulation of elements of a specified type T
(such as string
, int
, custom objects, etc.).
The T
in the List<T>
class represents the declaration indicating the class interconnectivity with certain types. The declaration of the list class (List<T> listName;
) clarifies what data type it will work with and manipulate.
Use the new
keyword with the default constructor to initialize an empty list to be stored temporarily in the listName
variable (listName = new List<T>();
). As the default capacity of a list is zero items, you can assign a different capacity to a list with the useful list.Capacity<T>
property.
Before initializing a List<T>
with values, it’s important to create an instance of the list:
List<string> stringList = new List<string>();
This line creates an empty List<string>
that can store strings.
Example Code:
using System;
using System.Collections.Generic;
public class listInitialization {
public static void Main(string[] args) {
// array as a parameter
string[] array = { "value1", "value2", "value3" };
// list initialization with values
List<string> expList = new List<string>(array);
// an alternative approach to initialize a list with values using the `List<T>` class | it is
// called single-line list initialization with values
List<string> expListAlt = new List<string>() { "value1", "value2", "value3" };
// display the `expList` list
Console.WriteLine("You initialized a list with " + expList.Count + " values.");
}
}
Output:
You initialized a list with 3 values.
The List<T>
interface is the generic equivalent of the ArrayList
class in C# and uses an array that can be dynamically increased as per one’s requirements.
As the capacity of a list can be increased to two billion elements on a 64-bit system, you can implement your custom synchronization to enable it to be accessed by multiple threads for value manipulation (read and write) that ensures the thread’s safety by locking a list.
Use the List.Add()
Method to Initialize a List With Values in C#
The list’s syntax is at first confusing, but it’s ideal for C# programs that lack strict memory or performance constraints. You may use the List.Add()
method to add new elements to the list after its declaration, but you can also create elements at the time of the declaration.
Creating a list of strings with a capacity of 0
with the help of a parameterless constructer is a universal way of list initialization. You can use the Add()
method of the List
class to add several string values to the list.
Here’s the syntax for using the Add()
method:
List<T> myList = new List<T>();
myList.Add(element);
Explanation:
List<T>
: This represents the generic list class in C#.myList
: An instance of theList<T>
class.Add(element)
: TheAdd()
method adds the specifiedelement
to the end of the listmyList
.
Example Code:
using System;
using System.Collections.Generic;
public class listInitialization {
public static void Main(string[] args) {
// using the `Add()` method to initialize a list with string values
List<string> expList = new List<string>();
expList.Add("value1");
expList.Add("value2");
expList.Add("value3");
expList.Add("value4");
expList.Add("value5");
// display the `expList` list
Console.WriteLine("You initialized a list with " + expList.Count + " values.");
}
}
Output:
You initialized a list with 5 values.
The code initializes a List<string>
called expList
by adding five string values ("value1"
to "value5"
) using the Add()
method. Finally, it displays the count of elements in expList
using Console.WriteLine()
.
The most interesting thing about this method is that it automatically checks the list’s capacity and resizes it whenever it feels necessary. It adds value at the end of the list and returns the value’s index so it can be easier to extend its capacity.
Use Collection Initializer to Initialize a List With Values in C#
The collection initializer syntax was first introduced in C# 3.0, and since then, it’s been helpful to assign values to collections. It’s a shortened syntax that uses curly brackets notation to initialize a list, and when it comes to the declaration, all of its values are assigned at once.
In general, it’s known to be a shorthand notation for calling the Add()
method multiple times by the C# compiler. You can initialize a list by using List<string> name = new List<string>
and use curly brackets to define its values like {new Element() {Id = 1, Name = "first"}
by populating objects of the Element
class.
The collection initializer syntax uses braces {}
to specify the initial elements within a List<T>
during its creation.
List<T> myList = new List<T> { value1, value2, value3, ... };
Individual object initializers enable you to create a list and populate it with an initial set of values enclosed in braces and separated by commas. Creating a list in C# from a set of known values became incredibly effortless.
You can identify a collection initializer by using the From
keyword followed by braces {}
, similar to the array literal syntax. It consists of a list of comma-separated values enclosed in braces {}
, preceded by the From
keyword.
Example Code:
using System;
using System.Collections.Generic;
public class listInitialization {
public static void Main(string[] args) {
// using `C# Collection Initializers` to initialize a list with values
List<Element> expList = new List<Element>() { new Element() { Id = 1, Name = "value1" },
new Element() { Id = 2, Name = "value2" },
new Element() { Id = 3, Name = "value3" },
new Element() { Id = 4, Name = "value4" } };
// display the `expList` list
Console.WriteLine("You initialized a list with " + expList.Count + " values.");
}
}
// create a class to define list values
public class Element {
public int Id { get; set; }
public string Name { get; set; }
}
Output:
You initialized a list with 4 values.
The code exemplifies the use of C# Collection Initializers to initialize a List<Element>
with instances of the custom Element
class. It creates a list named expList
, populates it with four Element
objects having distinct Id
and Name
properties, and then displays the count of elements in the list.
Collection Initializer With Inline Declaration
Inline declaration, introduced in C# 3.0, facilitates the creation of variables without explicitly specifying the data type. It relies on the var
keyword to infer the type of the variable based on the assigned value.
var myNumber = 10;
Here, myNumber
is implicitly declared as an integer based on the assigned value of 10
.
Combining collection initializers with inline declaration provides a concise and expressive way to initialize a list with values. This approach streamlines the code and enhances readability by declaring and populating the list in a single line.
var stringList = new List<string> { "apple", "banana", "orange" };
The var
keyword infers the type of stringList
as List<string>
based on the initialization expression, and the collection initializer within the curly braces populates the list with string values.
This example combines the inline declaration of a list with collection initializer syntax.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Initializing a list of strings using collection initializer and inline declaration
var names = new List<string> { "Alice", "Bob", "Charlie", "David" };
Console.WriteLine("List of names:");
foreach (var name in names) {
Console.Write(name + " ");
}
Console.WriteLine();
}
}
Output:
List of names:
Alice Bob Charlie David
This code demonstrates the use of a collection initializer and inline declaration in C# to initialize a list of strings named names
. Within the Main()
method, a List<string>
named names
is created and initialized with four string values: "Alice"
, "Bob"
, "Charlie"
, and "David"
.
The Console.WriteLine()
statement prints "List of names:"
to the console, followed by a foreach
loop that iterates through each string in the names
list and prints them to the console on the same line separated by spaces using Console.Write()
.
Finally, Console.WriteLine()
is used to move to the next line, resulting in the output displaying the list of names ("Alice Bob Charlie David"
) in a single line in the console.
Use the List.AddRange
Method to Initialize a List With Values in C#
When working with lists in C#, initializing them with predefined values is a common requirement. The List<T>
class provides numerous methods to manipulate and manage lists efficiently.
One such method, AddRange
, proves particularly useful for initializing lists by appending multiple elements in a single operation.
The AddRange
method in C# enables the concatenation of elements from a collection or an array to the end of a list. This method significantly simplifies the process of populating a list by allowing the addition of multiple elements in one swift action.
The syntax for the List.AddRange
method in C# is as follows:
public void AddRange(IEnumerable<T> collection)
Explanation of the components:
public
: Denotes that the method is accessible outside its containing class.void
: Indicates that the method does not return any value (it modifies the list it’s called on).AddRange
: Method name.<IEnumerable<T>>
: Type parameterT
represents the type of elements in the collection.IEnumerable<T>
signifies that theAddRange
method accepts a collection that implements theIEnumerable
interface for a specific typeT
.collection
: Parameter name. It expects an argument of typeIEnumerable<T>
, which is a collection or sequence of elements of the same type as the list.
This method is used to append elements from the specified collection to the end of the list. It’s particularly useful for adding multiple elements from arrays, other lists, or any collection implementing IEnumerable<T>
into an existing list in a single operation.
Let’s consider a straightforward example to illustrate the usage of AddRange
in initializing a list with values:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
var names = new List<string>();
string[] nameArray = { "Alice", "Bob", "Charlie", "David" };
names.AddRange(nameArray);
Console.WriteLine("List of names:");
foreach (var name in names) {
Console.Write(name + " ");
}
Console.WriteLine();
}
}
In this code, a List<string>
named names
is initialized, and a string array nameArray
containing "Alice"
, "Bob"
, "Charlie"
, and "David"
is created.
The AddRange
method is then used to add all elements from the nameArray
to the names
list. Subsequently, a foreach
loop iterates through the names
list, printing each name to the console.
Output:
List of names:
Alice Bob Charlie David
Conclusion
Initializing lists with string values in C# offers multiple methods for efficient and readable code. The List<T>
class, from System.Collections.Generic
, allows various approaches for populating lists:
- Basic Initialization: Using the
new
keyword withList<T>
and optionally assigning initial values provides a straightforward approach. Add
Method: Employing theAdd()
method allows incremental addition of elements to a list, making it flexible for dynamically growing lists.- Collection Initializer: Utilizing the collection initializer syntax
{}
enables concise list initialization in a single line, suitable for known values. AddRange
Method: This method stands out for its efficiency, allowing bulk addition of elements from arrays or collections to the list, streamlining initialization, and enhancing performance.
Each approach caters to specific needs—ranging from gradual element addition to quick, one-liner initializations or efficient bulk population. Understanding and employing these methods empower developers to efficiently handle list initialization in C#, ensuring flexibility and readability in code implementation.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub