How to Sort DataTable in C#
-
Sort
DataTable
Using theDataView.Sort
Property in C# -
Sort
DataTable
Using theDataTable.DefaultView
Property in C# -
Sort
DataTable
Using LINQ in C# -
Sort
DataTable
Using theDataTable.Select
Method in C# -
Sort
DataTable
UsingDataView
andIComparer
in C# - Conclusion
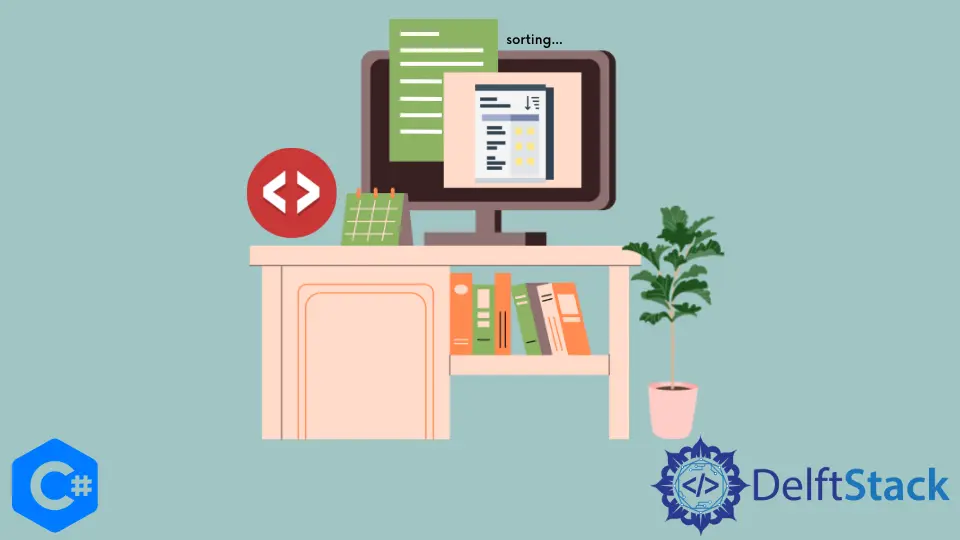
Sorting a DataTable
in C# is a common task in data manipulation, and there are several methods available to achieve this. In this article, we’ll explore various approaches, including the DataView.Sort
Property, DataTable.DefaultView
Property, LINQ Sorting, DataTable.Select
Method, and Custom Sorting using DataView
and IComparer
.
Each method has its unique syntax and use cases, offering flexibility based on specific sorting requirements.
Sort DataTable
Using the DataView.Sort
Property in C#
The DataView.Sort
property in C# provides a convenient way to sort a DataTable
. This property allows us to set the sort column and order for a DataTable
.
The syntax is simple: we create a DataView
from our DataTable
and assign the desired sorting expression to DataView.Sort
. The expression typically consists of the column name and an optional ASC
(ascending) or DESC
(descending) keyword.
Here’s the basic syntax:
DataView dataView = dataTable.DefaultView;
dataView.Sort = "ColumnName DESC";
DataTable sortedTable = dataView.ToTable();
Here, dataTable
is the DataTable
we want to sort, and ColumnName
is the column based on which we’re sorting in descending order.
Let’s walk through a complete working code example that demonstrates how to sort a DataTable
using DataView.Sort
in C#:
using System;
using System.Data;
namespace DataTableSortingExample {
class Program {
static void Main(string[] args) {
DataTable employeeTable = new DataTable();
employeeTable.Columns.Add("ID", typeof(int));
employeeTable.Columns.Add("Name", typeof(string));
employeeTable.Columns.Add("Salary", typeof(decimal));
employeeTable.Rows.Add(101, "John", 50000.00);
employeeTable.Rows.Add(102, "Jane", 60000.00);
employeeTable.Rows.Add(103, "Doe", 45000.00);
Console.WriteLine("Original Employee DataTable:");
foreach (DataRow row in employeeTable.Rows) {
Console.WriteLine($"ID: {row["ID"]}, Name: {row["Name"]}, Salary: {row["Salary"]}");
}
DataView dataView = employeeTable.DefaultView;
dataView.Sort = "Salary DESC";
DataTable sortedEmployeeTable = dataView.ToTable();
Console.WriteLine("\nSorted Employee DataTable (by Salary in Descending Order):");
foreach (DataRow row in sortedEmployeeTable.Rows) {
Console.WriteLine($"ID: {row["ID"]}, Name: {row["Name"]}, Salary: {row["Salary"]}");
}
}
}
}
In this example, we create a DataTable
named employeeTable
with columns ID
, Name
, and Salary
. After adding sample employee data, we display the original values.
We then create a DataView
(dataView
) from the DataTable
and use DataView.Sort
to sort the data based on the Salary
column in descending order. The sorted DataView
is then converted back to a DataTable
(sortedEmployeeTable
).
Finally, we display the sorted employee data. The output of the code will be:
Original Employee DataTable:
ID: 101, Name: John, Salary: 50000
ID: 102, Name: Jane, Salary: 60000
ID: 103, Name: Doe, Salary: 45000
Sorted Employee DataTable (by Salary in Descending Order):
ID: 102, Name: Jane, Salary: 60000
ID: 101, Name: John, Salary: 50000
ID: 103, Name: Doe, Salary: 45000
This output demonstrates the successful sorting of the DataTable based on the Salary
column in descending order using DataView.Sort
in C#.
Sort DataTable
Using the DataTable.DefaultView
Property in C#
When it comes to sorting a DataTable
in C#, another powerful tool at our disposal is the DataTable.DefaultView
property. This property provides a customized view of the DataTable
, allowing us to sort the data based on specified criteria.
To sort the DataTable
, we can utilize the DefaultView.Sort
property. The syntax involves setting the sort expression, similar to DataView.Sort
.
The expression typically comprises the column name and an optional ASC
(ascending) or DESC
(descending) keyword.
Here’s a basic representation:
dataTable.DefaultView.Sort = "ColumnName DESC";
dataTable = dataTable.DefaultView.ToTable(true);
Here, dataTable
represents the DataTable
we want to sort, and ColumnName
is the column based on which we’re sorting in descending order.
Let’s dive into a code example that demonstrates how to sort a DataTable
using DataTable.DefaultView
in C#:
using System;
using System.Data;
namespace DataTableSortingExample {
class Program {
static void Main(string[] args) {
DataTable productTable = new DataTable();
productTable.Columns.Add("ProductID", typeof(int));
productTable.Columns.Add("ProductName", typeof(string));
productTable.Columns.Add("Price", typeof(decimal));
productTable.Rows.Add(101, "Laptop", 1200.00);
productTable.Rows.Add(102, "Smartphone", 800.00);
productTable.Rows.Add(103, "Tablet", 500.00);
Console.WriteLine("Original Product DataTable:");
foreach (DataRow row in productTable.Rows) {
Console.WriteLine(
$"ID: {row["ProductID"]}, Name: {row["ProductName"]}, Price: {row["Price"]}");
}
productTable.DefaultView.Sort = "Price DESC";
productTable = productTable.DefaultView.ToTable(true);
Console.WriteLine("\nSorted Product DataTable (by Price in Descending Order):");
foreach (DataRow row in productTable.Rows) {
Console.WriteLine(
$"ID: {row["ProductID"]}, Name: {row["ProductName"]}, Price: {row["Price"]}");
}
}
}
}
In this example, a DataTable
named productTable
with columns ProductID
, ProductName
, and Price
is created. After adding sample product data, the original values are displayed.
The DataTable.DefaultView.Sort
property is then used to sort the data based on the Price
column in descending order. The sorted view is converted back to the original DataTable
(productTable
).
Finally, the sorted product data is displayed.
Output:
Original Product DataTable:
ID: 101, Name: Laptop, Price: 1200.00
ID: 102, Name: Smartphone, Price: 800.00
ID: 103, Name: Tablet, Price: 500.00
Sorted Product DataTable (by Price in Descending Order):
ID: 101, Name: Laptop, Price: 1200.00
ID: 102, Name: Smartphone, Price: 800.00
ID: 103, Name: Tablet, Price: 500.00
This output showcases the successful sorting of the DataTable
based on the Price
column in descending order using DataTable.DefaultView
in C#.
Sort DataTable
Using LINQ in C#
Sorting a DataTable
in C# can also be done using LINQ. LINQ (Language-Integrated Query) provides a concise and expressive syntax for querying and manipulating data.
To sort a DataTable
using LINQ, we use the OrderBy
or OrderByDescending
methods on the Enumerable
class. The syntax involves specifying the column by which we want to sort.
Here’s a basic representation:
var sortedRows = dataTable.AsEnumerable().OrderBy(row => row.Field<DataType>("ColumnName"));
DataTable sortedTable = sortedRows.CopyToDataTable();
Here, dataTable
represents the DataTable
we want to sort, and ColumnName
is the column based on which we’re sorting in ascending order. The OrderByDescending
method can be used for descending order.
Let’s proceed with a complete working code example demonstrating how to sort a DataTable
using LINQ in C#:
using System;
using System.Data;
using System.Linq;
namespace DataTableSortingExample {
class Program {
static void Main(string[] args) {
DataTable customerTable = new DataTable();
customerTable.Columns.Add("CustomerID", typeof(int));
customerTable.Columns.Add("FirstName", typeof(string));
customerTable.Columns.Add("LastName", typeof(string));
customerTable.Columns.Add("OrderCount", typeof(int));
customerTable.Rows.Add(101, "John", "Doe", 5);
customerTable.Rows.Add(102, "Jane", "Smith", 8);
customerTable.Rows.Add(103, "Bob", "Johnson", 3);
Console.WriteLine("Original Customer DataTable:");
foreach (DataRow row in customerTable.Rows) {
Console.WriteLine(
$"ID: {row["CustomerID"]}, Name: {row["FirstName"]} {row["LastName"]}, Orders: {row["OrderCount"]}");
}
var sortedRows =
customerTable.AsEnumerable().OrderByDescending(row => row.Field<int>("OrderCount"));
DataTable sortedCustomerTable = sortedRows.CopyToDataTable();
Console.WriteLine("\nSorted Customer DataTable (by OrderCount in Descending Order):");
foreach (DataRow row in sortedCustomerTable.Rows) {
Console.WriteLine(
$"ID: {row["CustomerID"]}, Name: {row["FirstName"]} {row["LastName"]}, Orders: {row["OrderCount"]}");
}
}
}
}
In this example, a DataTable
named customerTable
with columns CustomerID
, FirstName
, LastName
, and OrderCount
is created. After adding sample customer data, the original values are displayed.
LINQ is then used to sort the DataTable based on the OrderCount
column in descending order. The sorted rows are converted back to a DataTable (sortedCustomerTable
). Finally, the sorted customer data is displayed.
Output:
Original Customer DataTable:
ID: 101, Name: John Doe, Orders: 5
ID: 102, Name: Jane Smith, Orders: 8
ID: 103, Name: Bob Johnson, Orders: 3
Sorted Customer DataTable (by OrderCount in Descending Order):
ID: 102, Name: Jane Smith, Orders: 8
ID: 101, Name: John Doe, Orders: 5
ID: 103, Name: Bob Johnson, Orders: 3
This output demonstrates the successful sorting of the DataTable
based on the OrderCount
column in descending order using LINQ in C#.
Sort DataTable
Using the DataTable.Select
Method in C#
Another approach to sort a DataTable
in C# involves using the DataTable.Select
method. The DataTable.Select
method allows us to filter and sort rows based on specified criteria.
To achieve sorting, we can utilize this method with sorting expressions. The syntax involves specifying the filter expression and sort order within the method call.
Here’s a basic representation:
DataRow[] sortedRows = dataTable.Select("", "ColumnName DESC");
DataTable sortedTable = sortedRows.CopyToDataTable();
Here, dataTable
represents the DataTable
we want to sort, and ColumnName
is the column based on which we’re sorting in descending order. The first argument of Select
is the filter expression (empty in this case), and the second argument is the sort expression.
Let’s proceed with a complete working code example demonstrating how to sort a DataTable
using DataTable.Select
in C#:
using System;
using System.Data;
namespace DataTableSortingExample {
class Program {
static void Main(string[] args) {
DataTable studentTable = new DataTable();
studentTable.Columns.Add("StudentID", typeof(int));
studentTable.Columns.Add("FirstName", typeof(string));
studentTable.Columns.Add("LastName", typeof(string));
studentTable.Columns.Add("GPA", typeof(double));
studentTable.Rows.Add(201, "Alice", "Johnson", 3.8);
studentTable.Rows.Add(202, "Bob", "Smith", 3.5);
studentTable.Rows.Add(203, "Charlie", "Williams", 4.0);
Console.WriteLine("Original Student DataTable:");
foreach (DataRow row in studentTable.Rows) {
Console.WriteLine(
$"ID: {row["StudentID"]}, Name: {row["FirstName"]} {row["LastName"]}, GPA: {row["GPA"]}");
}
DataRow[] sortedRows = studentTable.Select("", "GPA DESC");
DataTable sortedStudentTable = sortedRows.CopyToDataTable();
Console.WriteLine("\nSorted Student DataTable (by GPA in Descending Order):");
foreach (DataRow row in sortedStudentTable.Rows) {
Console.WriteLine(
$"ID: {row["StudentID"]}, Name: {row["FirstName"]} {row["LastName"]}, GPA: {row["GPA"]}");
}
}
}
}
In this example, a DataTable
named studentTable
with columns StudentID
, FirstName
, LastName
, and GPA
is created. After adding sample student data, the original values are displayed.
The Select
method is then used to sort the DataTable based on the GPA
column in descending order. The selected rows are copied back to a DataTable
(sortedStudentTable
), and the sorted student data is displayed.
Output:
Original Student DataTable:
ID: 201, Name: Alice Johnson, GPA: 3.8
ID: 202, Name: Bob Smith, GPA: 3.5
ID: 203, Name: Charlie Williams, GPA: 4
Sorted Student DataTable (by GPA in Descending Order):
ID: 203, Name: Charlie Williams, GPA: 4
ID: 201, Name: Alice Johnson, GPA: 3.8
ID: 202, Name: Bob Smith, GPA: 3.5
This output demonstrates the successful sorting of the DataTable
based on the GPA
column in descending order using DataTable.Select
in C#.
Sort DataTable
Using DataView
and IComparer
in C#
In certain scenarios, custom sorting logic may be necessary when sorting a DataTable
in C#. The combination of DataView
and IComparer
provides a solution that allows us to define a custom comparison method for sorting.
Custom sorting with DataView
and IComparer
involves creating a class that implements the IComparer
interface, providing a custom comparison method. The DataView
is then used to apply the custom sorting logic.
The syntax can be outlined as follows:
public class CustomComparer : IComparer {
public int Compare(object x, object y) {
// Custom comparison logic here
}
}
// ...
DataView dv = new DataView(dataTable);
dv.Sort = "ColumnName";
DataTable sortedTable = dv.ToTable();
Here, CustomComparer
is a class implementing the IComparer
interface, and the custom comparison logic is defined in the Compare
method.
Let’s proceed with a complete working code example demonstrating how to perform custom sorting of a DataTable
using DataView
and IComparer
in C#:
using System;
using System.Collections;
using System.Data;
namespace DataTableSortingExample {
class CustomComparer : IComparer {
public int Compare(object x, object y) {
DataRow rowX = (DataRow)x;
DataRow rowY = (DataRow)y;
return String.Compare(rowX["Name"].ToString(), rowY["Name"].ToString(),
StringComparison.OrdinalIgnoreCase);
}
}
class Program {
static void Main(string[] args) {
DataTable cityTable = new DataTable();
cityTable.Columns.Add("CityID", typeof(int));
cityTable.Columns.Add("Name", typeof(string));
cityTable.Columns.Add("Population", typeof(int));
cityTable.Rows.Add(301, "New York", 8537673);
cityTable.Rows.Add(302, "Los Angeles", 3979576);
cityTable.Rows.Add(303, "Chicago", 2716000);
Console.WriteLine("Original City DataTable:");
foreach (DataRow row in cityTable.Rows) {
Console.WriteLine(
$"ID: {row["CityID"]}, Name: {row["Name"]}, Population: {row["Population"]}");
}
DataView dv = new DataView(cityTable);
dv.Sort = "Name";
DataTable sortedCityTable = dv.ToTable();
Console.WriteLine("\nSorted City DataTable (by Name in Case-Insensitive Order):");
foreach (DataRow row in sortedCityTable.Rows) {
Console.WriteLine(
$"ID: {row["CityID"]}, Name: {row["Name"]}, Population: {row["Population"]}");
}
}
}
}
In this example, a DataTable
named cityTable
with columns CityID
, Name
, and Population
is created. After adding sample city data, the original values are displayed.
A custom comparer class, CustomComparer
, is defined, implementing the IComparer
interface with custom sorting logic based on the Name
column. The Compare()
method compares two DataRow
objects (rowX
and rowY
), which is case-insensitive, as specified by the StringComparison.OrdinalIgnoreCase
parameter in the String.Compare
method.
The DataView
is then used to apply the custom sorting logic, resulting in the sortedCityTable
.
Finally, the sorted city data is displayed.
Output:
Original City DataTable:
ID: 301, Name: New York, Population: 8537673
ID: 302, Name: Los Angeles, Population: 3979576
ID: 303, Name: Chicago, Population: 2716000
Sorted City DataTable (by Name in Case-Insensitive Order):
ID: 303, Name: Chicago, Population: 2716000
ID: 302, Name: Los Angeles, Population: 3979576
ID: 301, Name: New York, Population: 8537673
This output demonstrates the successful custom sorting of the DataTable
based on the Name
column in case-insensitive order using DataView
and IComparer
in C#.
Conclusion
In this article, we’ve explored multiple methods for sorting a DataTable
in C#. Depending on your specific needs and preferences, you can choose the method that best suits your application.
Whether it’s basic sorting using DataView
or more complex scenarios with LINQ or custom comparers, these methods allow you to efficiently organize and present data within a DataTable
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn