C#에서 DataTable 정렬
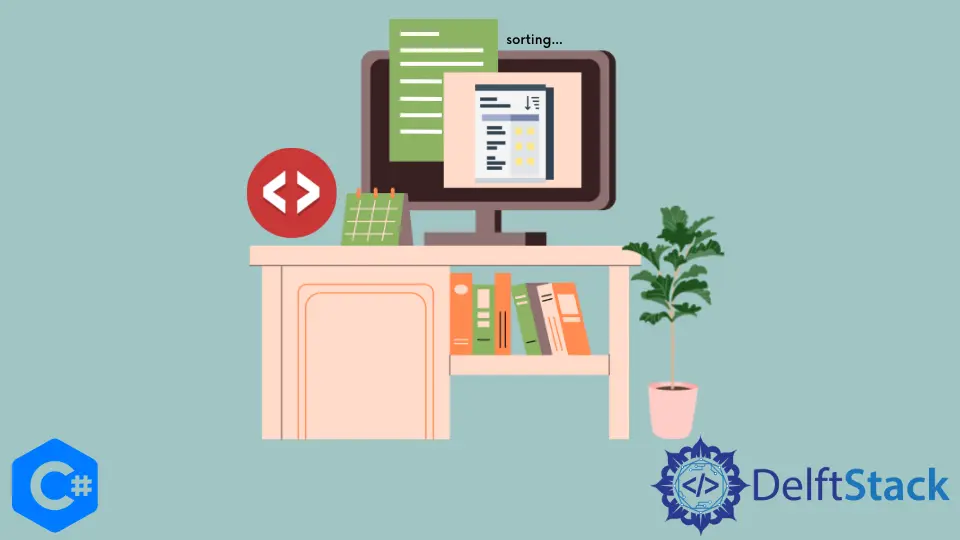
이 자습서에서는 C#에서 데이터 테이블을 정렬하는 방법을 소개합니다.
C#에서DataView.Sort
속성을 사용하여 DataTable 정렬
DataView.Sort
속성은 C#에서 데이터 테이블의 정렬 열을 가져 오거나 설정하는 데 사용됩니다. DataView.Sort = "Col_name"
과 같은 열 이름을 지정하여 데이터 테이블의 정렬 열을 설정할 수 있습니다. 기본적으로이 메서드는 데이터 테이블을 오름차순으로 정렬합니다. 열 이름 뒤에desc
를 지정하여 데이터 테이블을 내림차순으로 정렬 할 수 있습니다. 그런 다음 C#의DataView.ToTable()
함수를 사용하여이DataView
를DataTable
로 변환 할 수 있습니다. 다음 코드 예제는 C#에서DataView.Sort
속성을 사용하여 데이터 테이블을 정렬하는 방법을 보여줍니다.
using System;
using System.Data;
namespace datatable_sort {
class Program {
static void Main(string[] args) {
DataTable table1 = new DataTable();
DataColumn column1 = new DataColumn();
DataColumn column2 = new DataColumn();
column1.DataType = System.Type.GetType("System.Decimal");
column2.DataType = System.Type.GetType("System.Decimal");
column1.ColumnName = "Price";
column2.ColumnName = "Rating";
table1.Columns.Add(column1);
table1.Columns.Add(column2);
DataRow row;
for (int i = 0; i < 3; i++) {
row = table1.NewRow();
row["Price"] = i + 1;
row["Rating"] = i;
table1.Rows.Add(row);
}
// Displaying Original Values
Console.WriteLine("UnSorted Values");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
// Sorting the Table
DataView dv = table1.DefaultView;
dv.Sort = "Price desc";
DataTable sortedtable1 = dv.ToTable();
// Displaying Sorted Values
Console.WriteLine("Sorted Values by Descending order of Price");
foreach (DataRow r in sortedtable1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
}
}
}
출력:
UnSorted Values
Price = 1, Rating = 0
Price = 2, Rating = 1
Price = 3, Rating = 2
Sorted Values by Descending order of Price
Price = 3, Rating = 2
Price = 2, Rating = 1
Price = 1, Rating = 0
위의 코드에서 먼저 데이터 테이블table1
을 만들고Price
및Rating
에 두 개의 열을 추가했습니다. 그런 다음Price
의 내림차순으로 테이블을 정렬하고 새 값을 표시했습니다. DataView
클래스의 새 인스턴스를 생성하고DataView.Sort
속성으로 정렬했습니다. 그런 다음 C#의DataView.ToTable()
함수를 사용하여 정렬 된DataView
를DataTable
로 변환했습니다. 마지막으로 정렬 된 테이블에 데이터를 표시했습니다.
C#에서DataTable.DefaultView
속성을 사용하여 DataTable 정렬
DataTable.DefaultView
속성은 C#에서 데이터 테이블의 사용자 지정보기를 가져 오는 데 사용됩니다. DataTable.DefaultView.Sort
속성에 정렬 열을 지정하여 데이터 테이블을 정렬 할 수 있습니다. 기본적으로이 메서드는 데이터 테이블을 오름차순으로 정렬합니다. 열 이름 뒤에desc
를 지정하여 데이터 테이블을 내림차순으로 정렬 할 수 있습니다. 그런 다음 C#의DefaultView.ToTable()
함수를 사용하여 정렬 된 뷰를 데이터 테이블로 변환 할 수 있습니다. 다음 코드 예제는 C#에서DataTable.DefaultView
속성을 사용하여 데이터 테이블을 정렬하는 방법을 보여줍니다.
using System;
using System.Data;
namespace datatable_sort {
class Program {
static void Main(string[] args) {
DataTable table1 = new DataTable();
DataColumn column1 = new DataColumn();
DataColumn column2 = new DataColumn();
column1.DataType = System.Type.GetType("System.Decimal");
column2.DataType = System.Type.GetType("System.Decimal");
column1.ColumnName = "Price";
column2.ColumnName = "Rating";
table1.Columns.Add(column1);
table1.Columns.Add(column2);
DataRow row;
for (int i = 0; i < 3; i++) {
row = table1.NewRow();
row["Price"] = i + 1;
row["Rating"] = i;
table1.Rows.Add(row);
}
// Displaying Original Values
Console.WriteLine("UnSorted Values");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
// Sorting the Table
table1.DefaultView.Sort = "Price desc";
table1 = table1.DefaultView.ToTable(true);
// Displaying Sorted Values
Console.WriteLine("Sorted Values by Descending order of Price");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
}
}
}
출력:
UnSorted Values
Price = 1, Rating = 0
Price = 2, Rating = 1
Price = 3, Rating = 2
Sorted Values by Descending order of Price
Price = 3, Rating = 2
Price = 2, Rating = 1
Price = 1, Rating = 0
위의 코드에서 먼저 데이터 테이블table1
을 만들고Price
및Rating
이라는 두 개의 열을 추가했습니다. 그런 다음Price
의 내림차순으로table1
테이블을 정렬하고 새 값을 표시했습니다. table1.DefaultView.Sort
속성을 사용하여table1
테이블의 정렬 된보기를 만들었습니다. 그런 다음 C#의table1.DefaultView.ToTable(true)
함수를 사용하여 정렬 된 뷰를 테이블로 변환했습니다. 마지막으로 정렬 된 표에 데이터를 표시했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn