C# で DataTable を並べ替える
-
C# の
DataView.Sort
プロパティを使用して DataTable を並べ替える -
C# の
DataTable.DefaultView
プロパティを使用して DataTable を並べ替える
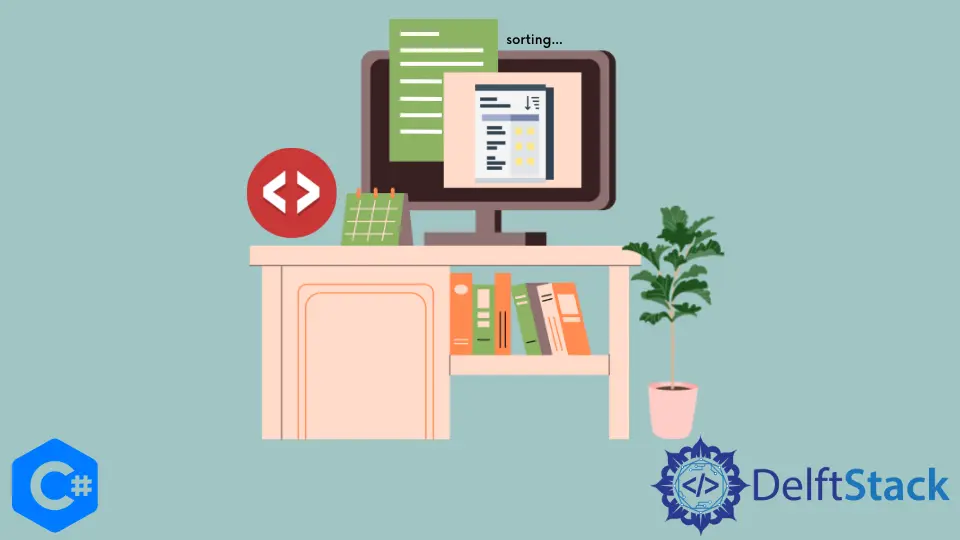
このチュートリアルでは、C# でデータテーブルを並べ替える方法を紹介します。
C# の DataView.Sort
プロパティを使用して DataTable を並べ替える
DataView.Sort
プロパティは、C# でデータテーブルの並べ替え列を取得または設定するために使用されます。DataView.Sort = "Col_name"
のように列名を指定することで、データテーブルの並べ替え列を設定できます。デフォルトでは、このメソッドはデータテーブルを昇順で並べ替えます。列名の後に desc
を指定して、データテーブルを降順で並べ替えることができます。次に、C# の DataView.ToTable()
関数を使用して、この DataView
を DataTable
に変換できます。次のコード例は、C# の DataView.Sort
プロパティを使用してデータテーブルを並べ替える方法を示しています。
using System;
using System.Data;
namespace datatable_sort {
class Program {
static void Main(string[] args) {
DataTable table1 = new DataTable();
DataColumn column1 = new DataColumn();
DataColumn column2 = new DataColumn();
column1.DataType = System.Type.GetType("System.Decimal");
column2.DataType = System.Type.GetType("System.Decimal");
column1.ColumnName = "Price";
column2.ColumnName = "Rating";
table1.Columns.Add(column1);
table1.Columns.Add(column2);
DataRow row;
for (int i = 0; i < 3; i++) {
row = table1.NewRow();
row["Price"] = i + 1;
row["Rating"] = i;
table1.Rows.Add(row);
}
// Displaying Original Values
Console.WriteLine("UnSorted Values");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
// Sorting the Table
DataView dv = table1.DefaultView;
dv.Sort = "Price desc";
DataTable sortedtable1 = dv.ToTable();
// Displaying Sorted Values
Console.WriteLine("Sorted Values by Descending order of Price");
foreach (DataRow r in sortedtable1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
}
}
}
出力:
UnSorted Values
Price = 1, Rating = 0
Price = 2, Rating = 1
Price = 3, Rating = 2
Sorted Values by Descending order of Price
Price = 3, Rating = 2
Price = 2, Rating = 1
Price = 1, Rating = 0
上記のコードでは、最初にデータテーブル table1
を作成し、それに Price
と Rating
の 2つの列を追加しました。次に、Price
の降順でテーブルを並べ替え、新しい値を表示しました。DataView
クラスの新しいインスタンスを作成し、DataView.Sort
プロパティで並べ替えました。次に、C# の DataView.ToTable()
関数を使用して、並べ替えられた DataView
を DataTable
に変換しました。最後に、ソートされたテーブルにデータを表示しました。
C# の DataTable.DefaultView
プロパティを使用して DataTable を並べ替える
DataTable.DefaultView
プロパティは、C# でデータテーブルのカスタマイズされたビューを取得するために使用されます。DataTable.DefaultView.Sort
プロパティで sort 列を指定することで、データテーブルを並べ替えることができます。デフォルトでは、このメソッドはデータテーブルを昇順で並べ替えます。列名の後に desc
を指定して、データテーブルを降順で並べ替えることができます。次に、C# の DefaultView.ToTable()
関数を使用して、並べ替えられたビューをデータテーブルに変換できます。次のコード例は、C# の DataTable.DefaultView
プロパティを使用してデータテーブルを並べ替える方法を示しています。
using System;
using System.Data;
namespace datatable_sort {
class Program {
static void Main(string[] args) {
DataTable table1 = new DataTable();
DataColumn column1 = new DataColumn();
DataColumn column2 = new DataColumn();
column1.DataType = System.Type.GetType("System.Decimal");
column2.DataType = System.Type.GetType("System.Decimal");
column1.ColumnName = "Price";
column2.ColumnName = "Rating";
table1.Columns.Add(column1);
table1.Columns.Add(column2);
DataRow row;
for (int i = 0; i < 3; i++) {
row = table1.NewRow();
row["Price"] = i + 1;
row["Rating"] = i;
table1.Rows.Add(row);
}
// Displaying Original Values
Console.WriteLine("UnSorted Values");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
// Sorting the Table
table1.DefaultView.Sort = "Price desc";
table1 = table1.DefaultView.ToTable(true);
// Displaying Sorted Values
Console.WriteLine("Sorted Values by Descending order of Price");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
}
}
}
出力:
UnSorted Values
Price = 1, Rating = 0
Price = 2, Rating = 1
Price = 3, Rating = 2
Sorted Values by Descending order of Price
Price = 3, Rating = 2
Price = 2, Rating = 1
Price = 1, Rating = 0
上記のコードでは、最初にデータテーブル table1
を作成し、Price
と Rating
の 2つの列を追加しました。次に、テーブル table1
を Price
の降順で並べ替え、新しい値を表示しました。table1.DefaultView.Sort
プロパティを使用して、table1
テーブルの並べ替えられたビューを作成しました。次に、そのソートされたビューを、C# の table1.DefaultView.ToTable(true)
関数を使用してテーブルに変換しました。最後に、ソートされたテーブルにデータを表示しました。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn