C# で DataTable をフィルター処理する
-
DataView.RowFilter
プロパティを使用してC#
でDataTable
をフィルター処理する -
CopyToDataTable()
メソッドを使用して、C#
でDataTable
をフィルタリングする -
Select()
メソッドを使用して、C#
でDataTable
をフィルタリングする
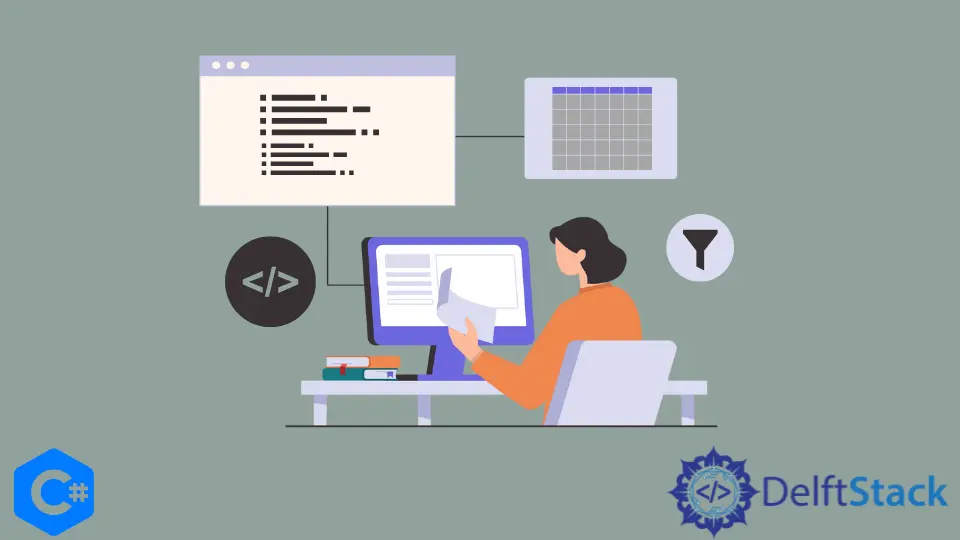
.NET Framework の DataView
は、フィルタリング用の databindtable
を表します。これは、C# の DataTable
のカスタマイズされたビューを表します。 DataTable
(DataView
が同期または接続されている) には、フィルタリングが必要なデータが含まれています。
DataView
はデータを格納できず、対応する DataTable
のデータのみを表します。 DataView
で実行されるすべての計算は、DataTable
に影響を与えます。
さらに、DataTable のデータを変更または修正すると、関連付けられているか接続されているすべての DataViews
に影響します。
DataView
クラスは、C# の System.Data
名前空間に存在します。 このチュートリアルでは、C# で DataTable
をフィルター処理する 3つの主な方法を説明します。
DataView.RowFilter
プロパティ、CopytoDataTable()
および Select()
メソッドは、C# で DataTable
をフィルター処理する主要な方法です。 データが大きくなり、高レベルの操作が必要になるため、フィルタリングはすべての C# アプリケーションに共通の要件です。
DataView.RowFilter
プロパティを使用して C#
で DataTable
をフィルター処理する
DataView.RowFilter
プロパティは、C# で DataTable
をフィルタリングする場合に非常に効果的です。 DataView
に関連付けられたデータを取得または設定することができ、各 DataTable
のデータをフィルタリングできる個々の DataView
オブジェクトを定義するのに役立ちます。
RowFilter
プロパティは、行をフィルター処理する方法を指定する文字列を表し、その文字列の値のデータ型は System.String
です。 RowFilter
値を username = "aUsername"
として割り当て、DataView
の行をフィルター処理して、フィルター処理されたデータを C# アプリケーションでプログラム的に表示できます。
RowFilter
プロパティの最も興味深い点は、DataView が動的ビューを提供する、基礎となる DataTable
のデータを変更/フィルター処理する機能です。 DataView の動的およびデータ バインディング機能により、データ バインディング C# アプリケーションに最適です。
DataTable
によって提供されるビューと同様に、C# の DataView
は単一のデータ セットを動的に表すことができ、さまざまなフィルターを実行できます。 RowFilter
プロパティを DataView
に適用できます。これにより、関連する DataTable
オブジェクトの行コレクションの、フィルター式または条件に一致しない不要なデータがすべて非表示になります。
using System;
using System.Data;
using System.Windows.Forms;
namespace DataTableExp {
public partial class Form1 : Form {
private DataSet dtSet;
public Form1() {
InitializeComponent();
CreateTable();
}
private void CreateTable() {
// Create a new DataTable.
DataTable filterExpCust = new DataTable("FilterUserDataTable");
DataColumn filterExpColumn;
DataRow filterExpDRow;
// create an `id` column
filterExpColumn = new DataColumn();
// define the column's type
filterExpColumn.DataType = typeof(Int32);
filterExpColumn.ColumnName = "id"; // column name
filterExpColumn.Caption = "User Account `ID`";
filterExpColumn.ReadOnly = false;
filterExpColumn.Unique = true;
// Add column to the DataColumnCollection.
filterExpCust.Columns.Add(filterExpColumn);
// create a `name` column
filterExpColumn = new DataColumn();
filterExpColumn.DataType = typeof(String);
filterExpColumn.ColumnName = "Name"; // column name
filterExpColumn.Caption = "User Account `Name`";
filterExpColumn.AutoIncrement = false;
filterExpColumn.ReadOnly = false;
filterExpColumn.Unique = false;
// Add column to the DataColumnCollection.
filterExpCust.Columns.Add(filterExpColumn);
// create an `address` column
filterExpColumn = new DataColumn();
filterExpColumn.DataType = typeof(String);
filterExpColumn.ColumnName = "Address"; // column name
filterExpColumn.Caption = "User Account Address";
filterExpColumn.ReadOnly = false;
filterExpColumn.Unique = false;
// add colume to the `filterExpColumn`
filterExpCust.Columns.Add(filterExpColumn);
// make the `id` the primary key of the table
DataColumn[] PrimaryKeyColumns = new DataColumn[1];
PrimaryKeyColumns[0] = filterExpCust.Columns["id"];
filterExpCust.PrimaryKey = PrimaryKeyColumns;
// create a new DataSet
dtSet = new DataSet();
// add `FilterUserDataTable` table to the DataSet.
dtSet.Tables.Add(filterExpCust);
// add data rows to the `FilterUserDataTable` table using the `NewRow` method
// first user details
filterExpDRow = filterExpCust.NewRow();
filterExpDRow["id"] = 1001;
filterExpDRow["Name"] = "Stephan Hawking";
filterExpDRow["Address"] = "79 Gulberg Road, Havana, Cuba";
filterExpCust.Rows.Add(filterExpDRow);
// second user details
filterExpDRow = filterExpCust.NewRow();
filterExpDRow["id"] = 1002;
filterExpDRow["name"] = "John Snow";
filterExpDRow["Address"] = " The Kings Landing, North California";
filterExpCust.Rows.Add(filterExpDRow);
// third user details
filterExpDRow = filterExpCust.NewRow();
filterExpDRow["id"] = 1003;
filterExpDRow["Name"] = "Scooby Doo";
filterExpDRow["Address"] = "194 St. Patrick Avenue, London, UK";
filterExpCust.Rows.Add(filterExpDRow);
}
private void button2_Click(object sender, EventArgs e) {
// Create a BindingSource
BindingSource bs = new BindingSource();
bs.DataSource = dtSet.Tables["FilterUserDataTable"];
// Bind data to DataGridView.DataSource
dataGridView1.DataSource = bs;
}
private void button1_Click(object sender, EventArgs e) {
// filter the datatable
// create a customers table and access it into a `dv` DataView
DataView dv = new DataView(dtSet.Tables["FilterUserDataTable"]);
// it will filter the row where `id` is `1001`
dv.RowFilter = "id = 1001";
// output the filtered datatable in a `dataGridView1` data grid view
dataGridView1.DataSource = dv;
}
}
}
出力:
*click `button2` to bind data to a data grid view*
*click `button2` to filter datatable*
id Name Address
1001 Stephan Hawking 79 Gulberg Road, Havana, Cuba
CreateTable()
メソッドは、id
、name
、および address
列を含む FilterUserDataTable
データテーブルを作成します。 button2
ボタンは、この DataTable
のデータを dataGridView1
にバインドできます。
button1
を押して DataTable
をフィルタリングし、フィルタリングされた結果を dataGridView1
に表示します。
CopyToDataTable()
メソッドを使用して、C#
で DataTable
をフィルタリングする
DataTable
をフィルタリングして、DataView
ではなく新しい DataTable
で結果を返すことができます。 その結果、新しい DataTable
には DataRow
オブジェクトのコピーが含まれ、入力 IEnumberable<T>
オブジェクトが与えられます。
DataTable
のソース IEnumerable<T>
を null
にすることはできません。 そうしないと、新しい DataTable
を作成できません。 DataTable
を選択し、その内容を DataGridView
のプロキシとして機能する BindingSource
にバインドされた新しいものにコピーするクエリに基づいています。
private void button1_Click(object sender, EventArgs e) {
DataTable _newFilteredDataTable =
dtSet.Tables["FilterUserDataTable"].Select("id = 1001").CopyToDataTable();
dataGridView1.DataSource = _newFilteredDataTable;
}
出力:
*click `button2` to bind data to a data grid view*
*click `button2` to filter datatable*
id Name Address
1001 Stephan Hawking 79 Gulberg Road, Havana, Cuba
_newFilteredDataTable
は、FilterUserDataTable
DataTable
のフィルタリングされたデータを保持します。ここで、id = 1001
です。 この C# コードは、この button1_Click
イベント コードを貼り付けることにより、前の C# の例で実行できます。
Select()
メソッドを使用して、C#
で DataTable
をフィルタリングする
Select()
メソッドは、DataTable
のシーケンスの各要素を新しいフォームに射影します。 これは System.Linq
名前空間に属しているため、LINQ を使用して C# の DataTable
からフィルター処理されたデータを取得することを楽しんでいる開発者にとっては朗報です。
このメソッドはオブジェクトを戻り値として利用し、アクションの実行に必要なすべての情報を格納します。 1つの文字列引数を使用して DataRow
オブジェクトのデータの配列を取得します。これがこの場合の条件です。
DataTable
を照会するパラメーターとして条件を指定して Select()
メソッドを使用できます。結果を取得した後、それを反復処理して必要なフィールドを出力できます。 DataTable.Select()
式を使用して、フィルター行を配列または DataTable
に割り当てます。
言い換えれば、DataTable
の Select()
メソッドはフィルターを受け取り、引き換えに引数をソートし、それらを DataRow
オブジェクトの配列に転送します。 フィルタリングされた DataTable
行を含む DataRow
オブジェクトは、FilterExpression
の条件を確認します。
// `dataTable` is the targeted DataTable in a C# program which contains data
// use `Select("id > 30")` to filter the data from `dataTable` where `id` is greater than `30`
// the `filterResult` will contain the filtered information of the `dataTable`
DataRow[] filterResult = dataTable.Select("id > 30");
foreach (DataRow filterRow in filterResult) {
Console.WriteLine("ID: {0} Name: {1} Address: {2}", filterRow[0], filterRow[1], filterRow[2]);
}
出力:
ID: 1003, Name: Scooby Doo, Address: 194 St. Patrick Avenue, London, UK
C# で大量のデータを扱う場合、DataTable
は極めて重要な役割を果たします。 このチュートリアルでは、C# で DataTable
をフィルター処理する 3つの異なる方法を説明しました。
DataTable
をフィルタリングする方法は、DataTable
からのデータのフェッチと操作において最適化され、効率的である必要があります。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub