Trier DataTable en C#
-
Trier DataTable avec la propriété
DataView.Sort
enC#
-
Trier DataTable avec la propriété
DataTable.DefaultView
enC#
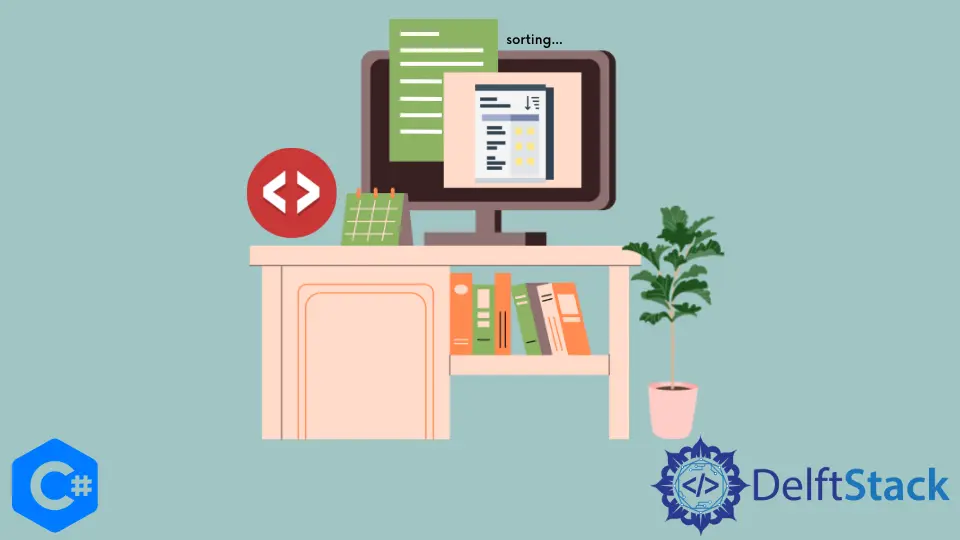
Ce didacticiel présentera les méthodes pour trier une table de données en C#.
Trier DataTable avec la propriété DataView.Sort
en C#
La propriété DataView.Sort
permet d’obtenir ou de définir la colonne de tri d’une table de données en C#. Nous pouvons définir la colonne de tri de notre datatable en spécifiant le nom de la colonne comme DataView.Sort = "Col_name"
. Par défaut, cette méthode trie la table de données dans l’ordre croissant. Nous pouvons spécifier desc
après le nom de la colonne pour trier la datatable par ordre décroissant. On peut ensuite convertir ce DataView
en DataTable
avec la fonction DataView.ToTable()
en C#. L’exemple de code suivant nous montre comment trier une table de données avec la propriété DataView.Sort
en C#.
using System;
using System.Data;
namespace datatable_sort {
class Program {
static void Main(string[] args) {
DataTable table1 = new DataTable();
DataColumn column1 = new DataColumn();
DataColumn column2 = new DataColumn();
column1.DataType = System.Type.GetType("System.Decimal");
column2.DataType = System.Type.GetType("System.Decimal");
column1.ColumnName = "Price";
column2.ColumnName = "Rating";
table1.Columns.Add(column1);
table1.Columns.Add(column2);
DataRow row;
for (int i = 0; i < 3; i++) {
row = table1.NewRow();
row["Price"] = i + 1;
row["Rating"] = i;
table1.Rows.Add(row);
}
// Displaying Original Values
Console.WriteLine("UnSorted Values");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
// Sorting the Table
DataView dv = table1.DefaultView;
dv.Sort = "Price desc";
DataTable sortedtable1 = dv.ToTable();
// Displaying Sorted Values
Console.WriteLine("Sorted Values by Descending order of Price");
foreach (DataRow r in sortedtable1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
}
}
}
Production:
UnSorted Values Price = 1, Rating = 0 Price = 2, Rating = 1 Price = 3,
Rating = 2 Sorted Values by Descending order of Price Price = 3,
Rating = 2 Price = 2, Rating = 1 Price = 1, Rating = 0
Dans le code ci-dessus, nous avons d’abord créé une table1
datable et y avons ajouté deux colonnes Price
et Rating
. Ensuite, nous avons trié le tableau par ordre décroissant de Prix
et affiché les nouvelles valeurs. Nous avons créé une nouvelle instance de la classe DataView
et l’avons triée avec la propriété DataView.Sort
. Nous avons ensuite converti le DataView
trié en DataTable
avec la fonction DataView.ToTable()
en C#. En fin de compte, nous avons affiché les données dans le tableau trié.
Trier DataTable avec la propriété DataTable.DefaultView
en C#
La propriété DataTable.DefaultView
permet d’obtenir une vue personnalisée d’une table de données en C#. Nous pouvons trier notre datatable en spécifiant la colonne de tri dans la propriété DataTable.DefaultView.Sort
. Par défaut, cette méthode trie la table de données dans l’ordre croissant. On peut spécifier desc
après le nom de la colonne pour trier la datatable par ordre décroissant. Nous pouvons ensuite convertir cette vue triée en une table de données avec la fonction DefaultView.ToTable()
en C#. L’exemple de code suivant nous montre comment trier une table de données avec la propriété DataTable.DefaultView
en C#.
using System;
using System.Data;
namespace datatable_sort {
class Program {
static void Main(string[] args) {
DataTable table1 = new DataTable();
DataColumn column1 = new DataColumn();
DataColumn column2 = new DataColumn();
column1.DataType = System.Type.GetType("System.Decimal");
column2.DataType = System.Type.GetType("System.Decimal");
column1.ColumnName = "Price";
column2.ColumnName = "Rating";
table1.Columns.Add(column1);
table1.Columns.Add(column2);
DataRow row;
for (int i = 0; i < 3; i++) {
row = table1.NewRow();
row["Price"] = i + 1;
row["Rating"] = i;
table1.Rows.Add(row);
}
// Displaying Original Values
Console.WriteLine("UnSorted Values");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
// Sorting the Table
table1.DefaultView.Sort = "Price desc";
table1 = table1.DefaultView.ToTable(true);
// Displaying Sorted Values
Console.WriteLine("Sorted Values by Descending order of Price");
foreach (DataRow r in table1.Rows) {
Console.WriteLine("Price = {0}, Rating = {1}", r[0], r[1]);
}
}
}
}
Production:
UnSorted Values Price = 1, Rating = 0 Price = 2, Rating = 1 Price = 3,
Rating = 2 Sorted Values by Descending order of Price Price = 3,
Rating = 2 Price = 2, Rating = 1 Price = 1, Rating = 0
Dans le code ci-dessus, nous avons d’abord créé une table1
datable et ajouté deux colonnes, Price
et Rating
. Ensuite, nous avons trié le tableau table1
par ordre décroissant de Price
et affiché les nouvelles valeurs. Nous avons créé une vue triée de notre table table1
avec la propriété table1.DefaultView.Sort
. Nous avons ensuite converti cette vue triée en table avec la fonction table1.DefaultView.ToTable(true)
en C#. En fin de compte, nous avons affiché les données dans le tableau trié.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn