How to Create DataTable in C#
-
Create Data Table With the
DataTable
Class inC#
-
Add Rows to Data Table in
C#
-
Export Data of Data Table to XML in
C#
-
Export Structure of Data Table to XML in
C#
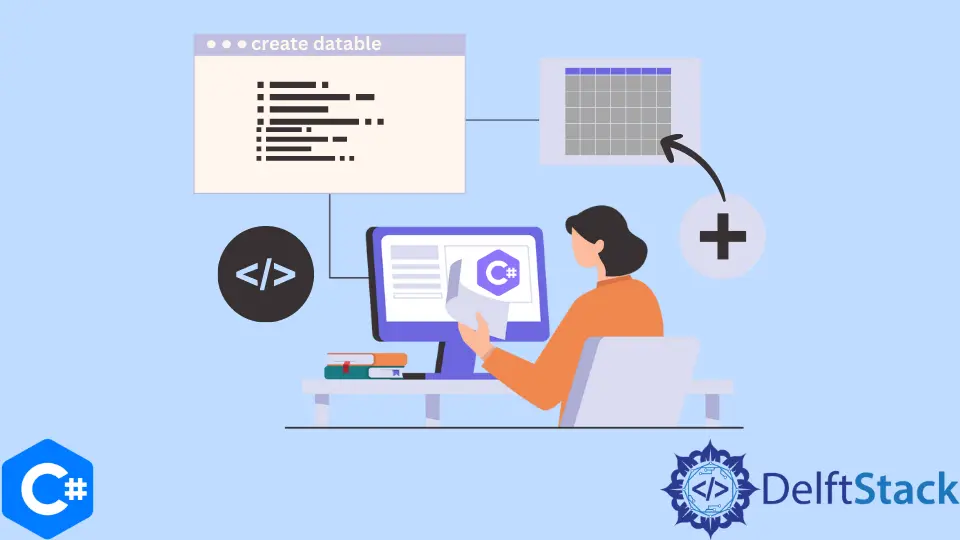
This tutorial will discuss the methods of creating and filling a data table in C#.
Create Data Table With the DataTable
Class in C#
The DataTable
class is used to create a data table in C#. A data table represents a table with relational data in C#. When we first create a data table with the DataTable
class, it does not have a specified schema. We have to create and add an object of the DataColumn
class to specify the structure of the data table. We can add the object of the DataColumn
class to the object of the DataTable
class with the DataTable.Columns.Add()
function in C#.
using System.Data;
namespace data_table {
class Program {
static void Main(string[] args) {
DataTable workTable = new DataTable("Customers");
DataColumn column1 = new DataColumn("Column1");
DataColumn column2 = new DataColumn("Column2");
workTable.Columns.Add(column1);
workTable.Columns.Add(column2);
}
}
}
In the above code, we created the data table workTable
with the name Customers
and two different columns, column1
and column2
, having names Column1
and Column2
, respectively. We specified the schema of the workTable
by adding both columns to it. Now we can add data to our table in the form of rows.
Add Rows to Data Table in C#
To add a row to our table, we have to create an object of the DataRow
class. The DataRow
class defines a new row to add data to our table in C#. We can add the object of the DataRow
class to the object of the DataTable
class with the DataTable.Row.Add()
function in C#. The following code example shows us how we can add data to our table with the DataRow
class in C#.
using System.Data;
namespace data_table {
class Program {
static void Main(string[] args) {
DataTable workTable = new DataTable("Customers");
DataColumn column1 = new DataColumn("Column1");
DataColumn column2 = new DataColumn("Column2");
workTable.Columns.Add(column1);
workTable.Columns.Add(column2);
DataRow row1 = workTable.NewRow();
row1["Column1"] = "value1";
row1["Column2"] = "value2";
workTable.Rows.Add(row1);
}
}
}
In the above code, we initialized the instance row1
of the DataRow
class with the workTable.NewRow()
function. We added data for both columns in the row1
and added row1
to the workTable
with the workTable.Rows.Add(row1)
function in C#.
We can also add a new row to our table with the following approach.
object[] o = { "object value1", "object value2" };
workTable.Rows.Add(o);
In the above code, we added a new row to the workTable
table without creating an instance of the DataRow
class. Another approach that can be used to insert a new row to our table in C# is given below.
workTable.Rows.Add("direct value1", "direct value2");
In the above code, we directly added values to a new row in the workTable
table without even declaring an array of objects.
Export Data of Data Table to XML in C#
Now that we have created our table with the DataTable
class, defined its structure with the DataColumn
class, and added multiple rows to our table, we can now display our table. We can export our whole table to an xml file with the DataTable.WriteXml()
function in C#. The DataTable.WriteXml(path)
function is used to write all the data of our table to an xml file in the path
. The following code example shows us how we can write our table data to an xml file with the DataTable.WriteXml()
function in C#.
using System.Data;
namespace data_table {
class Program {
static void Main(string[] args) {
DataTable workTable = new DataTable("Customers");
DataColumn column1 = new DataColumn("Column1");
DataColumn column2 = new DataColumn("Column2");
workTable.Columns.Add(column1);
workTable.Columns.Add(column2);
DataRow row1 = workTable.NewRow();
row1["Column1"] = "value1";
row1["Column2"] = "value2";
workTable.Rows.Add(row1);
object[] o = { "object value1", "object value2" };
workTable.Rows.Add(o);
workTable.Rows.Add("direct value1", "direct value2");
workTable.WriteXml("workTable.xml");
}
}
}
In the above code, we exported all the data inside the workTable
table to the xml file workTable.xml
with the workTable.WriteXml("workTable.xml")
function in C#. The contents of the workTable.xml
file are given below.
<?xml version="1.0" standalone="yes"?>
<DocumentElement>
<Customers>
<Column1>value1</Column1>
<Column2>value2</Column2>
</Customers>
<Customers>
<Column1>object value1</Column1>
<Column2>object value2</Column2>
</Customers>
<Customers>
<Column1>direct value1</Column1>
<Column2>direct value2</Column2>
</Customers>
</DocumentElement>
Export Structure of Data Table to XML in C#
Conversely, we can also export just the schema or structure of our table to an xml file in C#. The DataTable.WriteXmlSchema(path)
function is used to write the structure of our data table to an xml file in the path
. The following code example shows us how we can write our table’s structure to an xml file with the DataTable.WriteXmlSchema(path)
function in C#.
using System.Data;
namespace data_table {
class Program {
static void Main(string[] args) {
DataTable workTable = new DataTable("Customers");
DataColumn column1 = new DataColumn("Column1");
DataColumn column2 = new DataColumn("Column2");
workTable.Columns.Add(column1);
workTable.Columns.Add(column2);
DataRow row1 = workTable.NewRow();
row1["Column1"] = "value1";
row1["Column2"] = "value2";
workTable.Rows.Add(row1);
object[] o = { "object value1", "object value2" };
workTable.Rows.Add(o);
workTable.Rows.Add("direct value1", "direct value2");
workTable.WriteXml("workTable.xml");
workTable.WriteXmlSchema("workTableSchema.xml");
}
}
}
In the above code, we exported the structure of the workTable
table to the xml file workTableSchema.xml
with the workTable.WriteXmlSchema("workTableSchema.xml")
function in C#. The contents of the workTable.Schema.xml
file are given below.
<?xml version="1.0" standalone="yes"?>
<xs:schema id="NewDataSet" xmlns="" xmlns:xs="https://www.w3.org/2001/XMLSchema" xmlns:msdata="urn:schemas-microsoft-com:xml-msdata">
<xs:element name="NewDataSet" msdata:IsDataSet="true" msdata:MainDataTable="Customers" msdata:UseCurrentLocale="true">
<xs:complexType>
<xs:choice minOccurs="0" maxOccurs="unbounded">
<xs:element name="Customers">
<xs:complexType>
<xs:sequence>
<xs:element name="Column1" type="xs:string" minOccurs="0" />
<xs:element name="Column2" type="xs:string" minOccurs="0" />
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:choice>
</xs:complexType>
</xs:element>
</xs:schema>
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn