How to Create an Input Dialog Box in C#
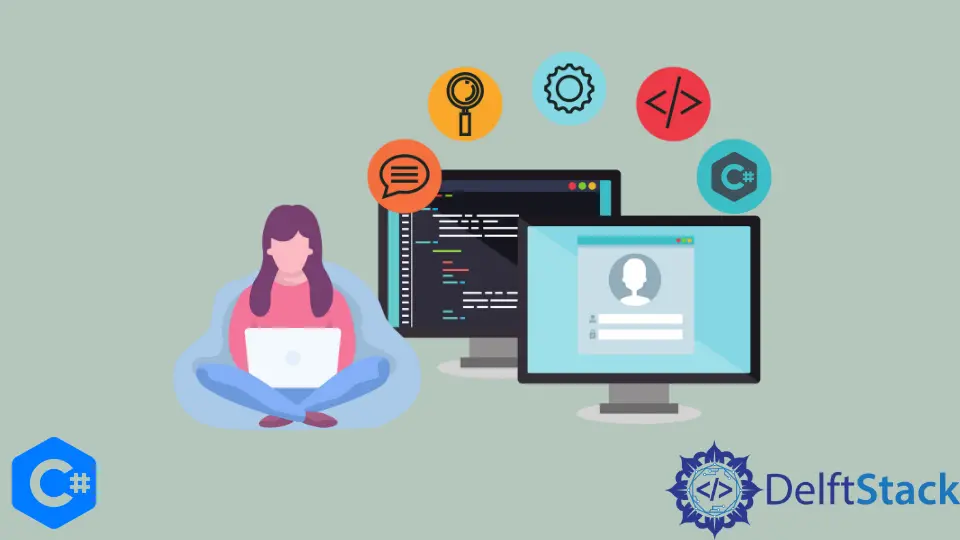
This tutorial will demonstrate to create an input dialog box in C# using two different methods similar to that in VB.Net.
An Input Dialog Box is a pop-up window that displays a message prompt and requests input from a user. The input can then be used in the code.
There is no version of VB.NET’s Input Dialog box in C# so that you can use one of two methods. The first and simplest is using the InputBox
provided in Microsoft.VisualBasic.Interaction
. The other method is by creating your own custom dialog box using System.Windows.Forms
and System.Drawing
.
Input Dialog Box Using Microsoft Visual Basic
As C# does not have its own version of an input dialog box like the one in VB.NET, you can add a reference to Microsoft.VisualBasic
and use its InputBox
.
To utilize Microsoft.VisualBasic
, you must first add it to the project’s references by following the steps below.
-
Navigate to the Solution Explorer
-
Right-click on References
-
Click
Add Reference
-
Click the
Assemblies
tab on the left -
Find the
Microsoft.VisualBasic.dll
file and clickOK
After successfully adding the reference, you can then use its using statement in your code.
using Microsoft.VisualBasic;
The Input Box itself is created by supplying the following parameters.
Prompt
: The message to be displayed within the window. This is the only mandatory parameter that needs to be passed.Title
: The window title to be displayedDefault
: The default value of the input text box- X Coordinate: The X Coordinate of the Input Box’s startup position
- Y Coordinate: The Y Coordinate of the Input Box’s startup position
string input = Interaction.InputBox("Prompt", "Title", "Default", 10, 10);
Once this input box has been called, the program will wait for a response before continuing on with the rest of the code.
Example:
using System;
using Microsoft.VisualBasic;
namespace VisualBasic_Example {
class Program {
static void Main(string[] args) {
// Create the input dialog box with the parameters below
string input =
Interaction.InputBox("What is at the end of the rainbow?", "Riddle", "...", 10, 10);
// After the user has provided input, print to the console
Console.WriteLine(input);
Console.ReadLine();
}
}
}
In the example above, we create an input box with a riddle prompt that appears in the top left corner of the screen. After input is submitted, the value is printed to the console.
Output:
The letter w
Custom Input Dialog Box Using Windows Forms in C#
Another option for creating an input dialog box is creating your own custom input dialog box. One benefit of creating an input dialog box is that it allows you to customize the window more than the first method. To utilize the code in the example below, you must first add a reference to System.Windows.Forms.dll
and System.Drawing.dll
by using the steps below.
-
Navigate to the Solution Explorer
-
Right-click on References
-
Click
Add Reference
-
Click the
Assemblies
tab on the left -
Find the
Microsoft.VisualBasic.dll
andSystem.Drawing.dll
files and clickOK
After successfully adding the reference, you can use their using statements in your code.
Example:
using System;
using System.Windows.Forms;
using System.Drawing;
namespace CustomDialog_Example {
class Program {
static void Main(string[] args) {
// Initialize the input variable which will be referenced by the custom input dialog box
string input = "...";
// Display the custom input dialog box with the following prompt, window title, and dimensions
ShowInputDialogBox(ref input, "What is at the end of the rainbow?", "Riddle", 300, 200);
// Print the input provided by the user
Console.WriteLine(input);
Console.ReadLine();
}
private static DialogResult ShowInputDialogBox(ref string input, string prompt,
string title = "Title", int width = 300,
int height = 200) {
// This function creates the custom input dialog box by individually creating the different
// window elements and adding them to the dialog box
// Specify the size of the window using the parameters passed
Size size = new Size(width, height);
// Create a new form using a System.Windows Form
Form inputBox = new Form();
inputBox.FormBorderStyle = FormBorderStyle.FixedDialog;
inputBox.ClientSize = size;
// Set the window title using the parameter passed
inputBox.Text = title;
// Create a new label to hold the prompt
Label label = new Label();
label.Text = prompt;
label.Location = new Point(5, 5);
label.Width = size.Width - 10;
inputBox.Controls.Add(label);
// Create a textbox to accept the user's input
TextBox textBox = new TextBox();
textBox.Size = new Size(size.Width - 10, 23);
textBox.Location = new Point(5, label.Location.Y + 20);
textBox.Text = input;
inputBox.Controls.Add(textBox);
// Create an OK Button
Button okButton = new Button();
okButton.DialogResult = DialogResult.OK;
okButton.Name = "okButton";
okButton.Size = new Size(75, 23);
okButton.Text = "&OK";
okButton.Location = new Point(size.Width - 80 - 80, size.Height - 30);
inputBox.Controls.Add(okButton);
// Create a Cancel Button
Button cancelButton = new Button();
cancelButton.DialogResult = DialogResult.Cancel;
cancelButton.Name = "cancelButton";
cancelButton.Size = new Size(75, 23);
cancelButton.Text = "&Cancel";
cancelButton.Location = new Point(size.Width - 80, size.Height - 30);
inputBox.Controls.Add(cancelButton);
// Set the input box's buttons to the created OK and Cancel Buttons respectively so the window
// appropriately behaves with the button clicks
inputBox.AcceptButton = okButton;
inputBox.CancelButton = cancelButton;
// Show the window dialog box
DialogResult result = inputBox.ShowDialog();
input = textBox.Text;
// After input has been submitted, return the input value
return result;
}
}
}
In the example above, we created a custom function that uses elements from System.Windows.Forms
and individually adds them to the Dialog Box. While you could hard code the different elements, you could also add parameters and reference them within the function to have as much customization as you’d like. After building and showing the dialog box, the program waits for the user to provide input to print it within the console.
Output:
the letter w