How to Compare Two Lists in C#
-
Compare Lists to Find Differences With the Linq Method in
C#
-
Compare Lists to Find Differences With the
List.Contains()
Function inC#
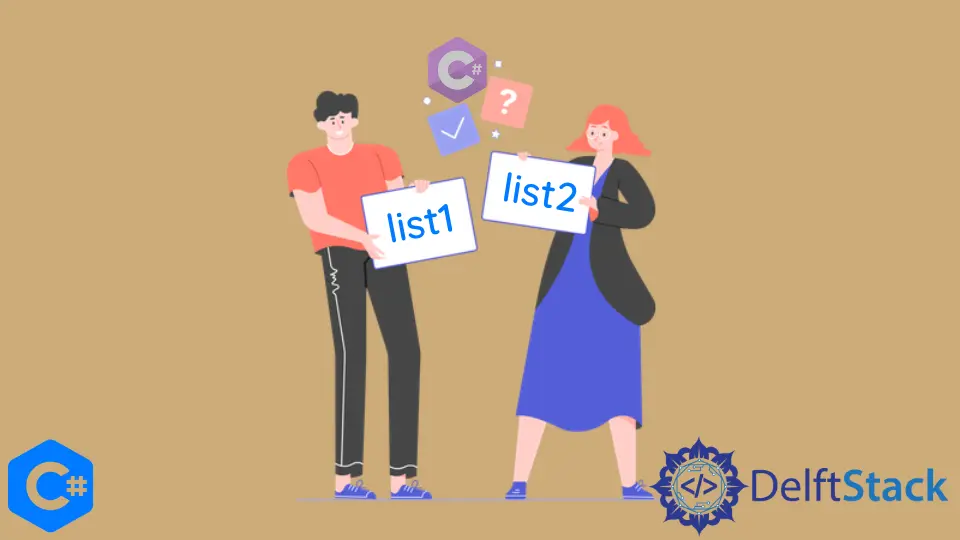
This tutorial will discuss methods to compare two lists to find differences in C#.
Compare Lists to Find Differences With the Linq Method in C#
Consider the following scenario, we have 2 lists, list1
and list2
, and we want to know which elements of the list1
are not present in list2
and which elements of list2
are not present in list1
. It can be done with the help of Except()
function in Linq. The Linq or language integrated query is used to query data structures in C#. The Except()
function returns a set of elements of one list not present in the other list. The following code example shows us how to compare two lists for differences with Linq in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace compare_lists {
class Program {
static void Main(string[] args) {
List<int> list1 = new List<int> { 1, 1, 2, 3, 4 };
List<int> list2 = new List<int> { 3, 4, 5, 6 };
List<int> firstNotSecond = list1.Except(list2).ToList();
var secondNotFirst = list2.Except(list1).ToList();
Console.WriteLine("Present in List1 But not in List2");
foreach (var x in firstNotSecond) {
Console.WriteLine(x);
}
Console.WriteLine("Present in List2 But not in List1");
foreach (var y in secondNotFirst) {
Console.WriteLine(y);
}
}
}
}
Output:
Present in List1 But not in List2
1
2
Present in List2 But not in List1
5
6
We compared the lists list1
and list2
for their differences in the above code. We first stored the elements present in list1
but not in list2
in the new list firstNotSecond
. We then stored the elements present in list2
but not in list1
in the new list secondNotFirst
. In the end, we printed the elements of both firstNotSecond
and secondNotFirst
lists. The only drawback is that any repeating values in one list which are not present in the other list will only be printed once.
Compare Lists to Find Differences With the List.Contains()
Function in C#
The List.Contains()
function is used to determine whether an element is present in a list or not in C#. The List.Contains(x)
function returns true if the element x
is present in the list and returns false if the element x
is not present. We can use List.Contains()
method with Linq to determine which elements are present in one list and not the other. The following code example shows us how we can compare two lists for differences with the List.Contains()
function in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace compare_lists {
class Program {
static void Main(string[] args) {
List<int> list1 = new List<int> { 1, 2, 3, 4 };
List<int> list2 = new List<int> { 3, 4, 5, 6 };
var firstNotSecond = list1.Where(i => !list2.Contains(i)).ToList();
var secondNotFirst = list2.Where(i => !list1.Contains(i)).ToList();
Console.WriteLine("Present in List1 But not in List2");
foreach (var x in firstNotSecond) {
Console.WriteLine(x);
}
Console.WriteLine("Present in List2 But not in List1");
foreach (var y in secondNotFirst) {
Console.WriteLine(y);
}
}
}
}
Output:
Present in List1 But not in List2
1
1
2
Present in List2 But not in List1
5
6
We compared the lists list1
and list2
for their differences in the above code. We first stored the elements present in list1
but not in list2
in the new list firstNotSecond
. We then stored the elements that were present in list2
but not in list1
in the new list secondNotFirst
. In the end, we printed the elements of both firstNotSecond
and secondNotFirst
lists. Here, the output is repeated as many times as the repeating value. This method should be preferred over the previous method if we want to consider repeating values.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn