How to Call Constructor From Another Constructor in C#
-
Call One Constructor From Another Constructor of the Same Class With the
this
Keyword in C# -
Call One Constructor From Another Constructor of the Same Class With the
base
Keyword in C# - Conclusion
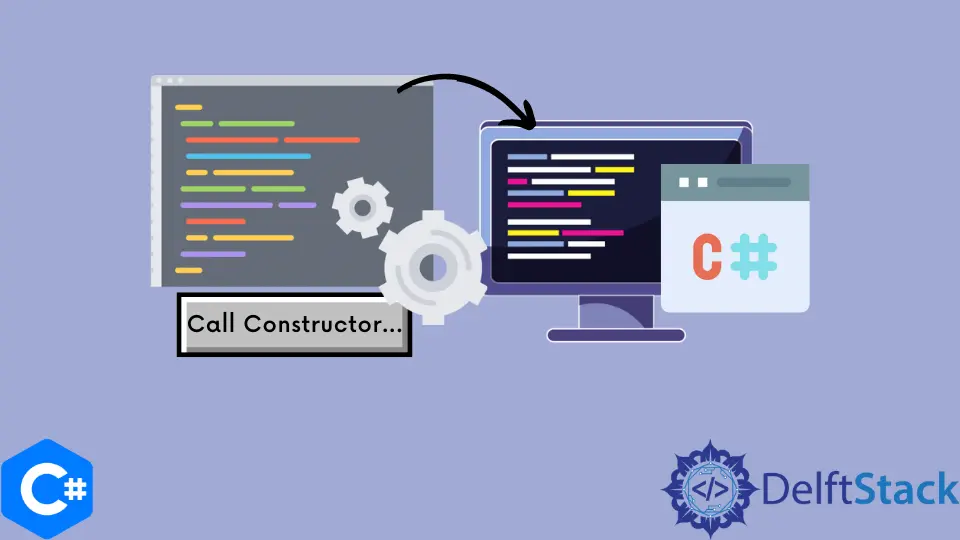
In the realm of C# programming, constructors play a pivotal role in initializing objects. Constructors are not just about setting values; they embody the concept of constructing an object’s state in a controlled, predictable manner.
C# offers a nuanced approach to constructor management, particularly in handling scenarios where one constructor needs to leverage the logic of another. This article delves into two significant facets of this mechanism: calling one constructor from another within the same class using the this
keyword and invoking a base class constructor from a derived class using base()
.
These techniques are more than syntactical conveniences; they are fundamental to writing clean, efficient, and maintainable code in C#.
Call One Constructor From Another Constructor of the Same Class With the this
Keyword in C#
If our class has multiple constructors and we want to call one constructor from another constructor, we can use the this
keyword in C#. The this
keyword is a reference to the instance of the current class in C#.
The syntax of using this()
in C# involves calling it within a constructor. this()
acts as a method call and can include parameters that match the signature of another constructor in the class.
Syntax:
public ClassName(ConstructorParameters) : this(OtherConstructorParameters) {
// Additional initialization
}
In this syntax, ClassName
is the class name, ConstructorParameters
are the parameters for this constructor, and OtherConstructorParameters
are the parameters for the constructor being called.
The following code example shows us how we can call one constructor of a class from another constructor of the same class with the this
keyword in C#.
using System;
namespace call_another_constructor {
class sample {
public sample() {
Console.WriteLine("Constructor 1");
}
public sample(int x) : this() {
Console.WriteLine("Constructor 2, value: {0}", x);
}
public sample(int x, int y) : this(x) {
Console.WriteLine("Constructor 3, value1: {0} value2: {1}", x, y);
}
}
class Program {
static void Main(string[] args) {
sample s1 = new sample(12, 13);
}
}
}
In our sample
class, we demonstrate constructor chaining with three constructors. Our default constructor, Constructor 1, simply outputs "Constructor 1"
.
When we use Constructor 2, which takes a single integer x
, it first chains to Constructor 1 using this()
and then proceeds to output "Constructor 2, value: {x}"
. For more complex initialization, we have Constructor 3 that takes two integers, x
and y
, and chains to Constructor 2 using this(x)
, thus also invoking Constructor 1 in the process.
Upon completion of these chained calls, Constructor 3 outputs "Constructor 3, value1: {x} value2: {y}"
. This sequence of constructor calls, when we instantiate sample
with two arguments, elegantly demonstrates the utility and efficiency of constructor chaining in C#.
Output:
Call One Constructor From Another Constructor of the Same Class With the base
Keyword in C#
In C#, constructors in derived classes can call constructors from their base classes using the base()
method. This is particularly useful in inheritance scenarios, where a derived class extends the functionality of a base class but also needs to inherit some of its base initialization logic.
The base()
method is used to ensure that the base class’s constructor is executed, allowing the derived class to build upon the established base initialization. This is crucial in object-oriented programming for maintaining a consistent state across an inheritance hierarchy.
When defining a constructor in a derived class, base()
is called as part of the constructor declaration.
Syntax:
public DerivedClassConstructor(Parameters) : base(BaseConstructorParameters) {
// Additional initialization for the derived class
}
Here, DerivedClassConstructor
is the constructor of the derived class, Parameters
are its parameters, and BaseConstructorParameters
are the parameters that match a constructor in the base class.
using System;
namespace ConstructorInheritanceExample {
class BaseClass {
public BaseClass() {
Console.WriteLine("Base class constructor called.");
}
}
class DerivedClass : BaseClass {
public DerivedClass() : base() {
Console.WriteLine("Derived class constructor called.");
}
}
class Program {
static void Main(string[] args) {
DerivedClass instance = new DerivedClass();
}
}
}
In this example, we have two classes: BaseClass
and DerivedClass
, where DerivedClass
inherits from BaseClass
. The BaseClass
has a default constructor that outputs a message.
The DerivedClass
also has a default constructor, and it explicitly calls the base class constructor using base()
. When we create an instance of DerivedClass
, the base()
call ensures that the constructor of BaseClass
is executed first, followed by the execution of DerivedClass's
own constructor.
Output:
Conclusion
The journey through the nuances of constructor chaining and inheritance in C# brings us to a fundamental understanding of object-oriented programming principles. Utilizing the this
and base
keywords for constructor calls isn’t merely a matter of syntax; it’s about architecting classes in a way that promotes code reuse, maintainability, and clarity.
In the case of this
, we observed how constructors within the same class could elegantly delegate tasks to one another, reducing redundancy. Meanwhile, base
serves as a bridge connecting derived classes to their ancestors, ensuring a harmonious and orderly initialization chain.
These mechanisms reflect the depth and flexibility of C#, offering programmers robust tools to craft efficient and structured applications. Whether you are a beginner grasping the fundamentals or an experienced developer refining your craft, understanding these aspects of C# is crucial in your journey of mastering object-oriented programming.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn