ArrayList vs List in C#
-
ArrayLists
in C# -
Lists
in C# -
Similarities Between
ArrayLists
andLists
in C# -
Differences Between
ArrayLists
andLists
in C# - Conclusion
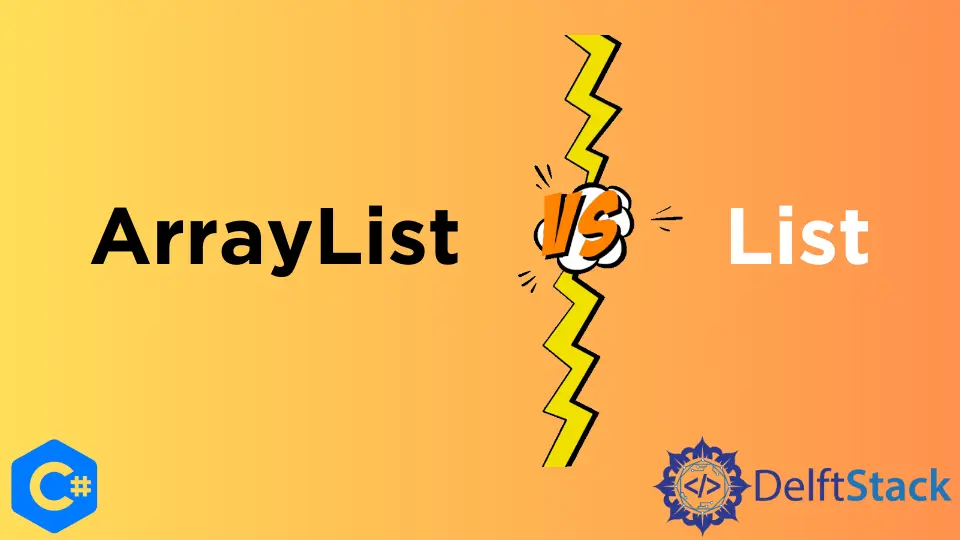
Collections play a crucial role in C# development, and choosing the right data structure can significantly impact the performance, maintainability, and functionality of your code. In C#, both ArrayLists
and Lists
serve as popular choices for managing collections of objects.
In this article, we will explore the similarities and differences between ArrayLists
and Lists
, helping you make informed decisions when selecting the appropriate data structure for your specific use case.
ArrayLists
in C#
The ArrayList
class, found in the System.Collections
namespace, is a widely used data structure in C#. It is a dynamic array that can resize itself to accommodate a variable number of elements, making it a valuable tool for scenarios where the size of the collection may vary.
ArrayList
stores object references, allowing it to hold data of various types, such as integers, strings, custom objects, and more. This flexibility sets it apart from strongly typed collections, such as arrays or generic lists.
Dynamic Sizing
One of the primary advantages of ArrayLists
is their dynamic sizing capability. Unlike fixed-size arrays, ArrayLists
can automatically grow or shrink their internal arrays as needed to accommodate the number of elements you add.
This feature is incredibly useful when you don’t know in advance how many elements you need to store.
var dynamicList = new ArrayList();
dynamicList.Add(1);
dynamicList.Add(2);
dynamicList.Add(3);
dynamicList.Add(4);
// No need to specify the size; it adjusts automatically.
Here, the dynamicList
automatically increases its capacity to store all the added elements.
Lack of Type Safety
While ArrayList
provides flexibility, it has a significant limitation: it lacks type safety. Since ArrayList
can store objects of different data types, it doesn’t offer compile-time type checking.
This can lead to runtime errors if you attempt to access elements incorrectly.
var mixedData = new ArrayList();
mixedData.Add(1);
mixedData.Add("Hello");
int num = (int)mixedData[1]; // Invalid cast, may result in runtime error
In this example, trying to cast a string to an integer could lead to unexpected runtime errors.
Despite their limitations, ArrayLists
are suitable in certain scenarios:
-
If you’re working with older code that utilizes
ArrayLists
, you might need to continue using them to maintain compatibility. -
When you need to store a collection of objects of various types,
ArrayList
can be an appropriate choice. However, you should be cautious about type-related runtime errors. -
If you’re unsure about the size of your collection and need it to dynamically adjust as elements are added or removed,
ArrayList
is a convenient option.
Lists
in C#
In contrast to ArrayLists
, Lists
belong to the System.Collections.Generic
namespace and are a part of C# generics, introduced with .NET
2.0. Generics allow you to create strongly typed collections that work with a specific data type.
Lists
are designed to provide type safety, better performance, and compile-time type checking, making them a preferred choice in modern C# development.
Strong Typing
The most prominent feature of Lists
is their strong typing. When you declare a List
, you specify the data type it will hold, ensuring that only elements of the specified data type can be added.
This strong typing offers type safety, preventing common programming errors.
List<int> numbers = new List<int>();
numbers.Add(1);
numbers.Add(2);
numbers.Add(3);
// Only integers can be added to the 'numbers' list.
In this example, the List
enforces type safety, preventing the addition of elements of different data types.
Performance Benefits
One of the key advantages of Lists
over ArrayLists
is their performance. Since Lists
are strongly typed, they do not involve the overhead of boxing and unboxing when dealing with value types.
Boxing and unboxing are processes that convert value types to reference types and vice versa, which can impact performance in large collections.
List<int> intList = new List<int>();
intList.Add(1);
intList.Add(2);
intList.Add(3);
// No boxing/unboxing for integers.
This better performance is particularly evident when dealing with value types like integers.
In contemporary C# development, Lists
are the preferred choice for storing collections due to their type safety, better performance, and integration with newer language features and libraries.
They are also more compatible with Language Integrated Query (LINQ), a powerful feature for querying and manipulating collections.
Similarities Between ArrayLists
and Lists
in C#
Despite their differences, ArrayLists
and Lists
share some fundamental characteristics:
Collection Storage
Both ArrayLists
and Lists
are used to store collections of objects. You can add, remove, and manipulate items within both data structures.
Code Example:
using System;
using System.Collections;
using System.Collections.Generic;
public class Program {
public static void Main() {
var arrayList = new ArrayList();
List<int> list = new List<int>();
// Adding elements to ArrayList and List
arrayList.Add(1);
arrayList.Add(2);
list.Add(1);
list.Add(2);
// Iterating through ArrayList and List
Console.WriteLine("ArrayList elements:");
foreach (var item in arrayList) {
Console.WriteLine(item);
}
Console.WriteLine("List elements:");
foreach (var item in list) {
Console.WriteLine(item);
}
}
}
In this code example, we demonstrate that both ArrayList
and List
can store and iterate through elements.
Output:
ArrayList elements:
1
2
List elements:
1
2
Both ArrayList
and List
store and iterate through their respective elements.
Dynamic Sizing
ArrayLists
and Lists
can dynamically adjust their size to accommodate more or fewer elements, making them flexible and suitable for situations where the number of items may vary over time.
Code Example:
using System;
using System.Collections;
using System.Collections.Generic;
public class Program {
public static void Main() {
var arrayList = new ArrayList();
List<int> list = new List<int>();
for (int i = 1; i <= 5; i++) {
arrayList.Add(i);
list.Add(i);
}
Console.WriteLine("ArrayList elements:");
foreach (var item in arrayList) {
Console.WriteLine(item);
}
Console.WriteLine("List elements:");
foreach (var item in list) {
Console.WriteLine(item);
}
}
}
In this code example, we add elements to both ArrayList
and List
, and they dynamically adjust their sizes to accommodate the added elements.
Output:
ArrayList elements:
1
2
3
4
5
List elements:
1
2
3
4
5
Both ArrayList
and List
dynamically adjust their sizes to accommodate the added elements.
Iteration
You can iterate through the elements of both ArrayLists
and Lists
using foreach
loops, making them compatible with standard collection processing techniques.
Code Example:
using System;
using System.Collections;
using System.Collections.Generic;
public class Program {
public static void Main() {
var arrayList = new ArrayList();
List<int> list = new List<int>();
arrayList.Add("Apple");
arrayList.Add("Banana");
arrayList.Add("Cherry");
list.Add(1);
list.Add(2);
list.Add(3);
Console.WriteLine("ArrayList elements:");
foreach (var item in arrayList) {
Console.WriteLine(item);
}
Console.WriteLine("List elements:");
foreach (var item in list) {
Console.WriteLine(item);
}
}
}
In this code example, we iterate through both ArrayList
and List
to print their elements.
Output:
ArrayList elements:
Apple
Banana
Cherry
List elements:
1
2
3
Both ArrayList
and List
allow iteration through their elements.
Differences Between ArrayLists
and Lists
in C#
Now, let’s summarize the key differences between ArrayLists
and Lists
:
Type Safety
ArrayLists
lack type safety and can store objects of any data type, whereas Lists
are strongly typed, ensuring type safety by allowing only elements of a specific data type.
Code Example:
using System;
using System.Collections;
using System.Collections.Generic;
public class Program {
public static void Main() {
var arrayList = new ArrayList();
List<int> list = new List<int>();
// Adding elements of different types to ArrayList and List
arrayList.Add(1);
arrayList.Add("Hello");
list.Add(1);
// list.Add("Hello"); // Uncommenting this line will result in a compile-time error
Console.WriteLine("ArrayList elements:");
foreach (var item in arrayList) {
Console.WriteLine(item);
}
Console.WriteLine("List elements:");
foreach (var item in list) {
Console.WriteLine(item);
}
}
}
In this code example, you can see that adding elements of different data types to ArrayList
is allowed but can lead to runtime errors, while attempting to add a string to a strongly typed List<int>
results in a compile-time error.
Output:
ArrayList elements:
1
Hello
List elements:
1
In the ArrayList
section, adding elements of different types is allowed, which can lead to runtime errors. In the List
section, attempting to add a string to a strongly typed List<int>
results in a compile-time error.
Performance
Lists
perform better than ArrayLists
, especially with value types, due to the absence of boxing and unboxing operations and better memory management.
Code Example:
using System;
using System.Collections;
using System.Collections.Generic;
public class Program {
public static void Main() {
var arrayList = new ArrayList();
List<int> list = new List<int>();
for (int i = 1; i <= 1000000; i++) {
arrayList.Add(i);
list.Add(i);
}
DateTime arrayListStartTime = DateTime.Now;
foreach (var item in arrayList) {
var value = (int)item;
}
DateTime arrayListEndTime = DateTime.Now;
TimeSpan arrayListElapsedTime = arrayListEndTime - arrayListStartTime;
DateTime listStartTime = DateTime.Now;
foreach (var item in list) {
var value = item;
}
DateTime listEndTime = DateTime.Now;
TimeSpan listElapsedTime = listEndTime - listStartTime;
Console.WriteLine("ArrayList Elapsed Time: " + arrayListElapsedTime);
Console.WriteLine("List Elapsed Time: " + listElapsedTime);
}
}
Output:
ArrayList Elapsed Time: 00:00:00.0203570
List Elapsed Time: 00:00:00.0038110
In this code example, we compare the performance of ArrayList
and List
when iterating through a large collection of integers, and you can observe the performance difference.
Here, the actual elapsed times may vary depending on your system’s performance. List
is expected to perform significantly faster than ArrayList
due to the absence of boxing and unboxing operations.
Generics
Lists
are part of C# generics, while ArrayLists
are not. Generics allow you to create type-safe data structures.
LINQ Compatibility
Lists
are more compatible with Language Integrated Query (LINQ) due to their type safety.
Code Example:
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
public class Program {
public static void Main() {
var arrayList = new ArrayList();
List<int> list = new List<int>();
for (int i = 1; i <= 5; i++) {
arrayList.Add(i);
list.Add(i);
}
var arrayListQuery = arrayList.Cast<int>().Where(x => x > 2);
var listQuery = list.Where(x => x > 2);
Console.WriteLine("ArrayList Query:");
foreach (var item in arrayListQuery) {
Console.WriteLine(item);
}
Console.WriteLine("List Query:");
foreach (var item in listQuery) {
Console.WriteLine(item);
}
}
}
In this code example, we demonstrate the use of LINQ with both ArrayList
and List
, showing that LINQ is more seamlessly integrated with List
.
Output:
ArrayList Query:
3
4
5
List Query:
3
4
5
Both ArrayList
and List
allow LINQ queries, but List
is more seamlessly integrated with LINQ, resulting in similar query results.
Modern Development
Lists
are the preferred choice in modern C# development due to their type safety, better performance, and compatibility with newer language features and libraries.
Conclusion
In conclusion, when choosing between ArrayLists
and Lists
in C#, you should consider your specific use case. While ArrayLists
may have a place in legacy or mixed-data-type scenarios, Lists
are generally the more modern, efficient, and type-safe choice for modern C# development.
Understanding these differences and similarities empowers you to make informed decisions when working with collections in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn