How to Add List to Another List in C#
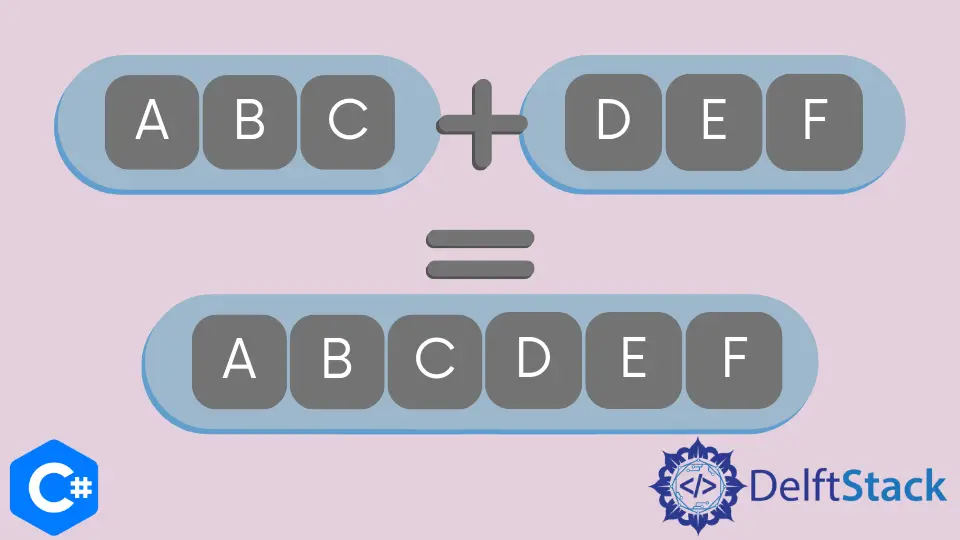
This tutorial will discuss methods to add one list’s elements at the end of another list in C#.
Add a List to Another List With the List.AddRange()
Function in C#
The easiest method for appending one list’s elements at the end of the other list is to use the List.AddRange()
method in C#. The List.AddRange(x)
method adds the elements of the collection x
in the list. The following code example shows us how to add one list to another list with the List.AddRange()
function in C#.
using System;
using System.Collections.Generic;
namespace add_list {
class Program {
static void Main(string[] args) {
List<string> first = new List<string> { "do", "rey", "me" };
List<string> second = new List<string> { "fa", "so", "la", "te" };
first.AddRange(second);
foreach (var e in first) {
Console.WriteLine(e);
}
}
}
}
Output:
do
rey
me
fa
so
la
te
We created and initialized 2 lists of strings, first
and second
, in the above code. We appended the second
list elements at the end of the first
list with the first.AddRange(second)
function. In the end, we displayed the elements of the first
list.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn